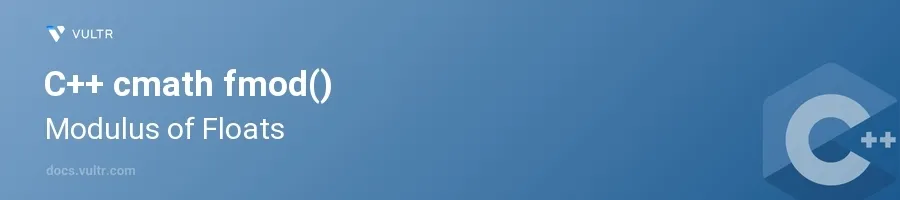
Introduction
The fmod()
function in C++ is part of the <cmath>
library and is used to compute the floating-point remainder of the division of two numbers. Unlike the modulus operator %
that works with integers, fmod()
deals specifically with floating-point numbers and is essential for mathematical calculations requiring precision with decimals.
In this article, you will learn how to effectively utilize the fmod()
function to handle various scenarios involving floating-point arithmetic. We will explore its basic usage and also demonstrate how to apply it for more complex calculations and error handling.
Using the fmod() Function
Basic Usage of fmod()
Include the
<cmath>
library in your C++ program to access thefmod()
function.Prepare two floating-point numbers for which you need to find the modulus.
Use
fmod()
by passing these numbers as arguments.Capture and print the result.
cpp#include <iostream> #include <cmath> int main() { double numerator = 5.3; double denominator = 2.1; double result = fmod(numerator, denominator); std::cout << "The result of fmod(" << numerator << ", " << denominator << ") is: " << result << std::endl; return 0; }
In the above example,
fmod(5.3, 2.1)
calculates the remainder when 5.3 is divided by 2.1, which gives a result of approximately 1.1. This demonstrates howfmod()
can handle calculations that include floating-point numbers.
Handling Special Cases with fmod()
Consider the behavior when division involves zero or negative values.
Check
fmod()
with a denominator of zero and with negative values to ensure you understand its error handling and results.cpp#include <iostream> #include <cmath> int main() { double result1 = fmod(4.2, 0); // Undefined, typically returns NaN double result2 = fmod(-7.3, 3.1); std::cout << "fmod(4.2, 0) yields: " << result1 << std::endl; std::cout << "fmod(-7.3, 3.1) yields: " << result2 << std::endl; return 0; }
Here, the
fmod(4.2, 0)
typically yields NaN (Not a Number), demonstrating the importance of checking for a zero denominator in production code. The callfmod(-7.3, 3.1)
correctly computes a negative result, demonstrating handling of negative numbers.
Conclusion
Using fmod()
function in C++ allows for precise handling of floating-point computations, especially when finding the remainder of division operations involving decimals. This function supplements the lack of support for floating-point modulus in the basic C++ %
operator and is crucial in fields requiring meticulous decimal calculations such as physics and finance. By understanding and utilizing fmod()
, you ensure accuracy and flexibility in numeric computations, handling errors effectively when unexpected values are encountered.
No comments yet.