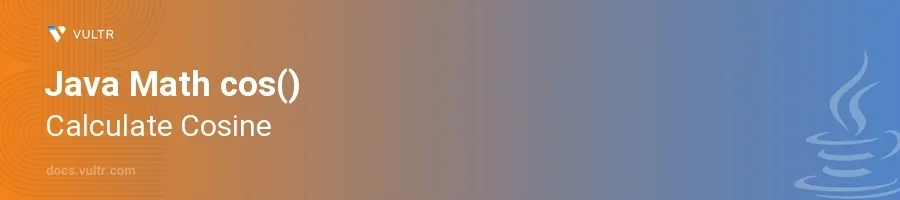
Introduction
The Math.cos()
function in Java calculates the cosine of a specified angle given in radians. This mathematical function is crucial in various computing tasks, particularly in fields involving geometry, physics, and simulations where trigonometric computations are essential.
In this article, you will learn how to effectively utilize the Math.cos()
function to compute the cosine of angles. Explore practical examples that demonstrate the accuracy and efficiency of using this function in Java programming.
Calculating Cosine with Math.cos()
Basic Usage of Math.cos()
Understand that
Math.cos()
expects the angle in radians, not degrees.To find the cosine of an angle in degrees, first convert the angle to radians and then apply the
cos()
function.javadouble degrees = 60; double radians = Math.toRadians(degrees); double cosineValue = Math.cos(radians); System.out.println("Cosine of " + degrees + " degrees is: " + cosineValue);
In the Java code snippet above, the angle in degrees (60 degrees) is first converted into radians using
Math.toRadians()
, and then theMath.cos()
function calculates the cosine of the angle.
Working with Negative Angles
Note that cosine function is even, which means
cos(theta)
is the same ascos(-theta)
.Apply
Math.cos()
to a negative angle and observe the output, confirming the even nature of the cosine function.javadouble negativeDegrees = -45; double radians = Math.toRadians(negativeDegrees); double cosineValue = Math.cos(radians); System.out.println("Cosine of " + negativeDegrees + " degrees is: " + cosineValue);
In the code above, despite the angle being negative, the output for cosine demonstrates symmetry, as cosine inherently handles negative values by its periodic and even function characteristics.
Using Math.cos() in Loop for Multiple Calculations
Use a loop to compute cosine values for a range of angles, which can be particularly useful in simulations or animations where trigonometric functions form the backbone of the operations.
Implement a loop to calculate and print cosine values for every 30 degrees from 0 to 360 degrees.
javafor (int degrees = 0; degrees <= 360; degrees += 30) { double radians = Math.toRadians(degrees); double cosineValue = Math.cos(radians); System.out.println("Cosine of " + degrees + " degrees is: " + cosineValue); }
This example will output the cosine values for angles from 0 to 360 degrees in 30-degree increments. It's a practical demonstration of how looping structures can handle repeated calculations efficiently with
Math.cos()
.
Conclusion
The Math.cos()
function in Java is a vital tool for performing trigonometric calculations, crucial in numerous developmental scenarios where precise mathematical computations are necessary. By understanding how to convert degrees to radians and applying the function across different conditions, including negative angles and multiple iterative calculations, you enhance your mathematical processing capabilities in Java applications. Implement these techniques to ensure your mathematical calculations are accurate, efficient, and effective.
No comments yet.