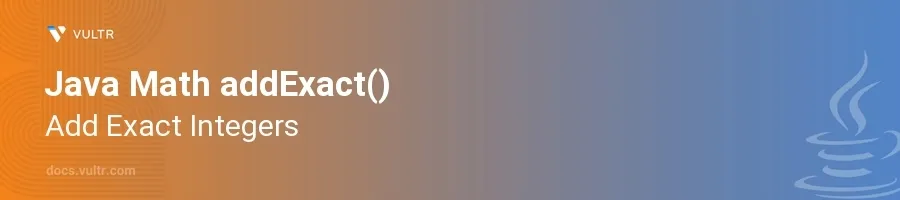
Introduction
The Math.addExact()
method in Java is a precision tool designed to perform addition with an exact result, throwing an exception if the operation results in overflow. This method is part of the Math
class in Java's standard library, specifically useful in scenarios where maintaining precise calculations and avoiding erroneous results due to overflow is crucial.
In this article, you will learn how to effectively use the Math.addExact()
method in Java. Explore scenarios where this method becomes essential and understand how to integrate it into your Java applications to ensure exact calculation results while managing potential overflows gracefully.
Understanding Java Math.addExact()
The Math.addExact()
method provides a safer way to handle integer addition, guaranteeing that the exact result of the operation is obtained or indicating a problem if an overflow occurs.
Basic Usage of addExact()
Review the method signature:
Math.addExact(int x, int y)
orMath.addExact(long x, long y)
. This method can accept either two integers or two longs.Use
Math.addExact()
to add two numbers with overflow checking.javaint result = Math.addExact(2147483640, 8); System.out.println("Result: " + result);
This code adds
2147483640
and8
safely. The result will be printed as2147483648
.
Handling Overflow
Understand that
Math.addExact()
throws anArithmeticException
if the addition results in an overflow.Implement exception handling to manage overflows.
javatry { int result = Math.addExact(2147483647, 1); System.out.println("Result: " + result); } catch (ArithmeticException e) { System.out.println("Overflow occurred: " + e.getMessage()); }
This example attempts to add
2147483647
and1
. Since this operation causes an overflow, it catches theArithmeticException
and prints an overflow message.
Practical Application: Financial Calculations
Consider using
Math.addExact()
in financial applications where precise computation of large sums of money is critical.Handle potential overflows to protect the integrity of financial data.
javatry { long totalAmount = Math.addExact(9999999999L, 8888888888L); System.out.println("Total Amount: " + totalAmount); } catch (ArithmeticException e) { System.out.println("Transaction overflow: " + e.getMessage()); }
In this example, exact addition ensures the correct total amount of money being processed. Overflow handling is crucial to avoid discrepancies.
Conclusion
The Math.addExact()
method in Java is an essential function for applications needing exact arithmetic operations without the risk of unnoticed overflows. It enhances the reliability and accuracy of your applications, especially in environments like financial computing, where precision is paramount. By integrating the Math.addExact()
method, ensure precise results and manage overflows effectively, maintaining the integrity and reliability of computational results.
No comments yet.