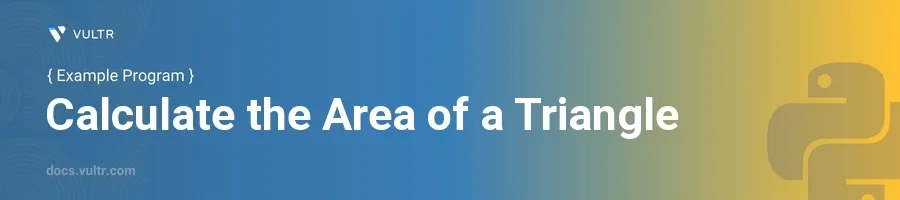
Introduction
Calculating the area of a triangle is a fundamental problem that often arises in geometry and various practical applications, from civil engineering to computer graphics. Understanding how to compute this efficiently can aid in numerous technical fields. Python, with its simplicity and readability, provides a straightforward approach to solving this problem using basic mathematical formulas.
In this article, you will learn how to create a Python program that calculates the area of a triangle. This will cover different methods including the use of the standard geometric formula and Heron's formula. Each method will be demonstrated through clear, concise examples.
Using the Standard Area Formula
Calculate Area with Base and Height
The most direct approach to determine the area of a triangle when the base and height are known uses the formula: Area = 0.5 * base * height.
Prompt the user to input the base and height of the triangle.
Calculate the area using the formula.
Display the result.
pythonbase = float(input("Enter the base of the triangle: ")) height = float(input("Enter the height of the triangle: ")) area = 0.5 * base * height print("The area of the triangle is:", area)
In this snippet, the program collects the base and height from the user, calculates the area using the arithmetic operation
0.5 * base * height
, and then prints the calculated area.
Using Heron's Formula
Heron's formula provides a way to calculate the area of a triangle when you know the lengths of all three sides. First, compute the semi-perimeter, then use it to determine the area.
Get input from the user for the lengths of the sides of the triangle.
Calculate the semi-perimeter.
Use Heron's formula to find the area.
Output the area.
pythonimport math a = float(input("Enter the length of side a: ")) b = float(input("Enter the length of side b: ")) c = float(input("Enter the length of side c: ")) s = (a + b + c) / 2 area = math.sqrt(s * (s - a) * (s - b) * (s - c)) print("The area of the triangle is:", area)
Here, the program first calculates the semi-perimeter
s
of the triangle. It then applies Heron's formula using Python'smath.sqrt()
function to compute the square root necessary for this method. The area is then printed.
Conclusion
This article demonstrated how to compute the area of a triangle in Python using two distinct methods: one employing the basic formula with base and height, and the other using Heron's formula for when all side lengths are known. By mastering these techniques, you can quickly apply them to solve problems in real-world applications and academic settings. The provided examples serve as a foundation to extend and apply these calculations in various Python projects and geometrical computations.
No comments yet.