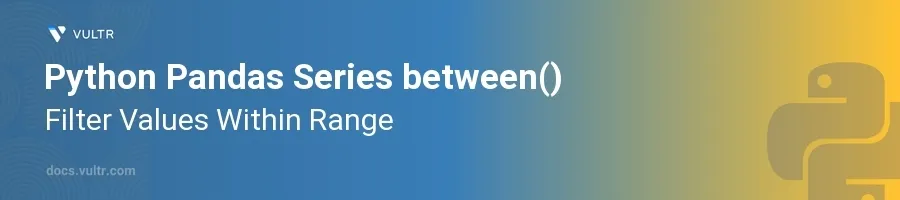
Introduction
Python's Pandas library is a powerhouse for data manipulation and analysis, particularly popular among data scientists and analysts for its robust features that handle complex data operations effortlessly. One such feature in Pandas is the between()
method applied on Series
objects. This method is incredibly useful for filtering data within a specified range, making it easier to narrow down data sets according to specific requirements.
In this article, you will learn how to utilize the between()
method to filter values within a range effectively. You will explore various examples that include checking numeric ranges, date ranges, and conditional checks within categorical data. This guide also demonstrates how to handle inclusive and exclusive ranges based on your data filtering needs.
Using between() with Numeric Data
Filter Numeric Range in Series
Import the Pandas library and create a
Series
containing numeric data.Use the
between()
method to specify the range of interest.pythonimport pandas as pd data = pd.Series([10, 20, 30, 40, 50]) filtered_data = data.between(20, 40) print(filtered_data)
This code filters the
Series
to identify elements within the 20 to 40 range, inclusive of the boundary values. The result is a BooleanSeries
indicatingTrue
for elements within the range andFalse
otherwise.
Understanding the Inclusivity of Ranges
Remember that
between()
includes values at boundary points by default.Adjust inclusivity using the
inclusive
parameter.pythoninclusive_filter = data.between(20, 40, inclusive="both") # Default behavior exclusive_filter = data.between(20, 40, inclusive="neither") print("Inclusive filter:\n", inclusive_filter) print("Exclusive filter:\n", exclusive_filter)
The inclusive filter includes elements equal to 20 and 40. The exclusive filter excludes these boundary values, focusing strictly on values between them.
Using between() with Date Ranges
Filter Date Range in Time Series Data
Parse a
Series
of dates using Pandas.Employ the
between()
method to filter dates within a specified range.pythondates = pd.Series(pd.date_range("2021-01-01", periods=5, freq='D')) date_filtered = dates.between("2021-01-02", "2021-01-04") print(date_filtered)
This snippet sets up a
Series
of consecutive dates and filters those that fall between January 2, 2021, and January 4, 2021, inclusive.
Handling Non-standard Date Formats
Convert strings to datetime objects if your series contains unrecognized date formats.
Filter using the
between()
method.pythondate_strs = pd.Series(['2021/01/01', '2021/01/02', '2021/01/03']) dates_converted = pd.to_datetime(date_strs, format='%Y/%m/%d') date_range_filter = dates_converted.between("2021-01-02", "2021-01-03") print(date_range_filter)
In this example, date strings are converted to standard datetime objects before filtering, ensuring accuracy in range checking.
Using between() with Categorical Data
Filter Categorical Data Based on Lexicographical Order
Create a
Series
of categorical data.Utilize
between()
to filter data based on alphabet sequence or custom criteria.pythoncategories = pd.Series(['apple', 'banana', 'cherry', 'date']) cat_filtered = categories.between('banana', 'date') print(cat_filtered)
This code filters the fruits between 'banana' and 'date' based on their lexicographical order, including both 'banana' and 'date'.
Conclusion
The between()
method in Pandas Series is a versatile tool for filtering data within specific ranges, whether numeric, date-related, or categorical. By understanding how to manipulate the inclusivity parameters and dealing with different data types, you can master data filtering to meet your analysis needs. Apply these techniques in your day-to-day data tasks to improve the precision and efficiency of your data-driven decisions. Explore different parameters and conditions to best tailor the functionality to your specific data scenarios.
No comments yet.