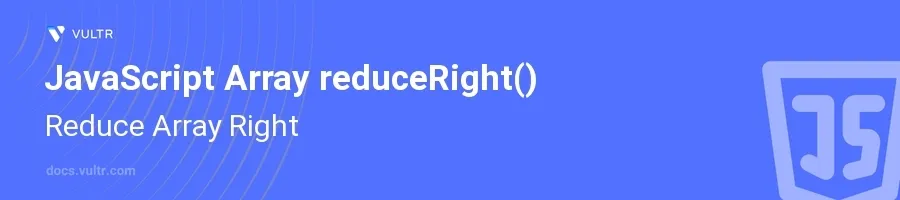
Introduction
The reduceRight()
method in JavaScript processes an array from right to left, applying a reducer function to each element to produce a single output value. This feature is especially valuable when the order of execution affects the outcome, such as in mathematical operations that depend on the arrangement of values or when handling actions in reverse sequence.
In this article, you will learn how to utilize the reduceRight()
method effectively. Discover how this method functions with arrays to perform cumulative or chain-based operations where the processing order is crucial.
Understanding reduceRight() Method
Basic Usage of reduceRight()
Understand that
reduceRight()
takes up to four arguments in its callback function: accumulator, currentValue, currentIndex, and array.Initialize an array and use
reduceRight()
to perform a simple summation.javascriptconst numbers = [1, 2, 3, 4]; const sum = numbers.reduceRight((accumulator, currentValue) => accumulator + currentValue); console.log(sum);
The
reduceRight()
function here processes each element from right to left, summing up the elements (4 + 3 + 2 + 1
) to return10
.
Handling Initial Values
Specify an initial value for the accumulator in the
reduceRight()
method to determines its starting value.Use
reduceRight()
to concatenate strings starting from the last element.javascriptconst words = ['ends', 'this', 'reverse', 'to', 'Welcome']; const sentence = words.reduceRight((accumulator, currentValue) => accumulator + ' ' + currentValue, ''); console.log(sentence);
This code snippet concatenates the strings from right to left, starting with an empty string as the initial value, resulting in the sentence "Welcome to reverse this ends".
Practical Applications
Complex Accumulations
Apply
reduceRight()
in scenarios where detailed accumulation logic, such as composite objects or nested arrays, is required.Construct a cumulative object that categorizes elements based on their type.
javascriptconst items = [1, 'hello', true, false, 'world', 42]; const categorized = items.reduceRight((accumulator, item) => { const type = typeof item; accumulator[type] = (accumulator[type] || []).concat(item); return accumulator; }, {}); console.log(categorized);
In this example,
reduceRight()
is used to categorize items into types (numbers, strings, booleans), starting from the last element and moving to the first. The result is a composite object with arrays of elements, segregated by type.
Use with Arrays of Objects
Utilize
reduceRight()
to process arrays of objects, particularly useful in data manipulation tasks like summing values from object properties.Calculate the total amount from a list of transactions.
javascriptconst transactions = [ {id: 1, amount: 100}, {id: 2, amount: 200}, {id: 3, amount: 300} ]; const totalAmount = transactions.reduceRight((sum, transaction) => sum + transaction.amount, 0); console.log(totalAmount);
Steps through each transaction object from right to left (starting with the last transaction), summing up the amounts to compute a total of
600
.
Conclusion
The reduceRight()
function in JavaScript is an invaluable tool for reducing arrays into a single value, from right to left, enabling more controlled and order-sensitive data processing tasks. Flexible enough to handle everything from simple arithmetic to complex object manipulations, this method proves essential in many different programming contexts. By making use of the techniques discussed, leverage the full potential of array transformations in your projects, maintaining both clarity and efficiency in your code.
No comments yet.