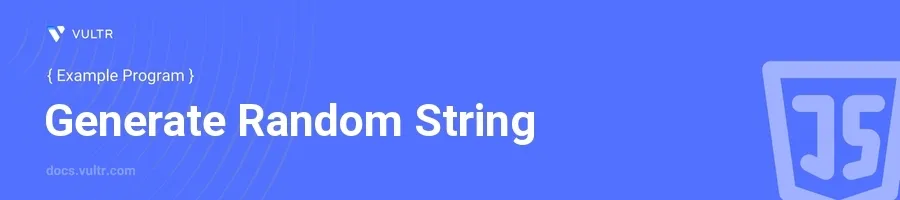
Introduction
The ability to generate random strings in JavaScript is a fundamental skill, useful in many applications such as creating unique identifiers, generating passwords, or handling tokens for session management. Random strings can be customized to various lengths and character sets depending on the specific needs of the application.
In this article, you will learn how to write a JavaScript program to generate random strings. Explore examples that demonstrate generating strings of different lengths and compositions, ensuring you can tailor the functionality to your specific requirements.
Generating a Basic Random String
Create a Function to Generate Random Strings
Define a function that accepts the desired length of the string as a parameter.
Inside the function, define a variable for the characters that can be included in the random string. Typically, this includes uppercase and lowercase letters, and optionally numbers and symbols.
Initialize a variable to hold the resulting random string.
Use a loop to select random characters from the defined character set until the length of the desired string is reached.
javascriptfunction generateRandomString(length) { const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; let result = ''; for (let i = 0; i < length; i++) { const randomIndex = Math.floor(Math.random() * characters.length); result += characters.charAt(randomIndex); } return result; }
This function generates a random string by selecting characters from a predefined set.
Math.random()
generates a random number, which is then scaled by the length of thecharacters
string to select a character at a random index.
Test the Function
Call the function with different lengths to see it in action.
javascriptconsole.log(generateRandomString(10)); console.log(generateRandomString(20));
Each call to
generateRandomString
outputs a random string of 10 or 20 characters long, using a mix of uppercase and lowercase letters and numbers.
Advanced Usage
Customizing the Character Set
Modify the
generateRandomString
function to accept an additional parameter for custom characters.Replace the fixed character set with the custom set provided by the user.
javascriptfunction generateRandomString(length, customCharacters) { const characters = customCharacters || 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; let result = ''; for (let i = 0; i < length; i++) { const randomIndex = Math.floor(Math.random() * characters.length); result += characters.charAt(randomIndex); } return result; }
This version allows for the injection of a custom character set. If none is provided, it defaults to the standard alphanumeric set.
Generate String with Custom Characters
Call the modified function with a custom set of characters.
javascriptconsole.log(generateRandomString(15, 'abcdef12345'));
This generates a random string of 15 characters long, but only using the characters 'a' to 'f' and '1' to '5'.
Conclusion
Generating random strings in JavaScript is straightforward with the use of Math.random()
and a basic loop mechanism. By customizing the function, you can adapt the random string generator for various purposes, including testing, unique identifiers, or security features in web applications. Utilizing these techniques, tailor random string generation to meet any project's requirements, ensuring flexibility and functionality in your JavaScript programming.
No comments yet.