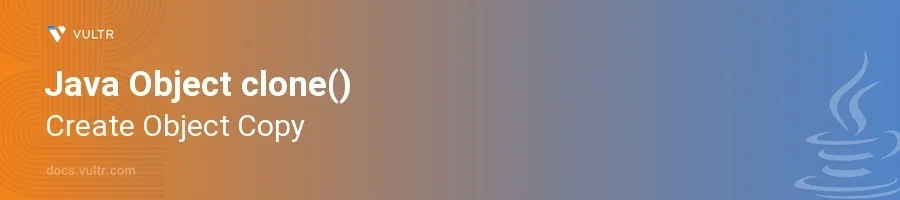
Introduction
In Java, creating a copy of an object often involves using the clone()
method, which is part of the Object
class. This method provides a means to duplicate an existing object, preserving its state. Proper implementation of object copying is crucial in many scenarios, such as when dealing with objects that have numerous properties or complex recursive structures.
In this article, you will learn how to effectively utilize the clone()
method in Java. Explore the requirements for correct implementation, different ways to handle object cloning, and how to manage deep versus shallow copies.
Implementing Cloneable Interface
Understand the Cloneable Interface
Recognize that the
clone()
method is protected in theObject
class and realizes a shallow copy.Note that to use
clone()
, a class must implement theCloneable
interface. Without it, cloning an object will throw aCloneNotSupportedException
.javapublic class Example implements Cloneable { int a; int b; public Object clone() throws CloneNotSupportedException { return super.clone(); } }
This simple example shows how a class should implement the
Cloneable
interface to enable object cloning. Here, callingclone()
creates a shallow copy of the object.
Creating a Test Class
Develop a test class to demonstrate cloning functionality.
Perform cloning and display the copied object properties to verify correctness.
javapublic class TestClone { public static void main(String[] args) { Example ex = new Example(); ex.a = 10; ex.b = 20; try { Example exClone = (Example) ex.clone(); System.out.println("Cloned object values: a = " + exClone.a + ", b = " + exClone.b); } catch (CloneNotSupportedException e) { System.out.println("Clone not supported"); } } }
This code snippet creates an instance of
Example
, sets its properties, and attempts to clone it. It then prints the values of the cloned object to demonstrate that the cloning was successful.
Managing Deep Copies with clone()
Understanding Shallow vs. Deep Copy
- Recognize that a shallow copy duplicates the object's top-level properties, but not the nested or referenced objects.
- Understand that a deep copy duplicates everything, including the objects referenced by the main object, ensuring complete independence of the copies.
Implement Deep Copy Using clone()
Modify the class to include a reference to another object and update the
clone()
method to manage deep copying.javapublic class DeepExample implements Cloneable { int x; Point p; // Assume Point is a class with its own clone method public Object clone() throws CloneNotSupportedException { DeepExample clone = (DeepExample) super.clone(); clone.p = (Point) p.clone(); return clone; } }
This modification ensures that when
DeepExample
is cloned, not only are the primitive fields copied, but the referencedPoint
object is also separately cloned, thus achieving a deep copy.
Conclusion
Using the clone()
function in Java requires a thorough understanding of the type of copy needed: shallow or deep. Implementing the Cloneable
interface and overriding the clone()
method provides control over object duplication processes. Remember, deep copying is essential when working with objects that contain non-primitive fields to prevent changes in the cloned object from affecting the original object. GLsizei the techniques discussed, establish effective, accurate object copy functionality in Java applications.
No comments yet.