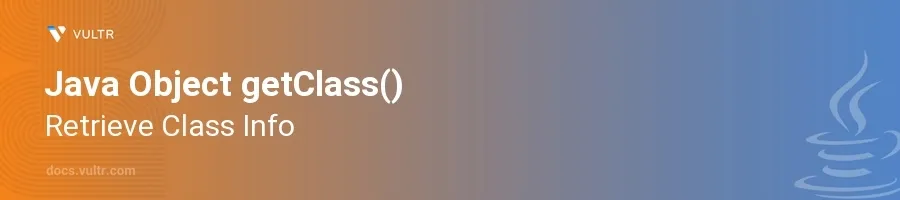
Introduction
The getClass()
method in Java is an integral part of the Java Reflection API, allowing developers to retrieve the runtime class of an object. This method, defined in the java.lang.Object
class, returns a Class
object that is associated with the instance for which it is called. This capability is crucial for dynamic type checking, creating generic factories, and developing frameworks that depend on runtime type modifications.
In this article, you will learn how to effectively leverage the getClass()
method in various practical scenarios. Explore its use in obtaining class information dynamically, making type comparisons, and integrating it within generic programming and frameworks.
Utilizing getClass() for Dynamic Information Retrieval
Retrieve Class Name
Initialize an object of any class.
Invoke the
getClass()
method followed bygetName()
to get the class name.javaObject obj = new String("Hello World!"); String className = obj.getClass().getName(); System.out.println("Class Name: " + className);
This code snippet retrieves the class name of the object
obj
. ThegetClass()
method is called onobj
, and thengetName()
is chained to return the actual class name.
Check if Two Objects are of the Same Class
Compare the class of two different objects to determine if they are instances of the same class.
Use the
.equals()
method on theClass
objects obtained from each instance.javaObject obj1 = new String("Hello"); Object obj2 = new String("World"); boolean sameClass = obj1.getClass().equals(obj2.getClass()); System.out.println("Same Class: " + sameClass);
This code compares the classes of
obj1
andobj2
. By callinggetClass()
on both objects, it retrieves theirClass
objects and checks for equality.
Using getClass() in Generic Methods
Use
getClass()
in a generic method to handle different types based on the class of the object passed.Implement a method that alters behavior based on the class type.
javapublic static void printClassInfo(Object obj) { Class<?> objClass = obj.getClass(); System.out.println("Class Name: " + objClass.getName()); System.out.println("Simple Name: " + objClass.getSimpleName()); System.out.println("Canonical Name: " + objClass.getCanonicalName()); } printClassInfo("A simple test string.");
When this method is called with a
String
object, it prints various reflective information about theString
class, demonstrating howgetClass()
can be used in generic programming to tailor method behavior in accordance with the object's class type.
Conclusion
The getClass()
method in Java is a versatile tool for accessing dynamic class information at runtime. It enables the development of flexible and reusable code, as it allows class specifics to be determined on the fly. This method is indispensable in scenarios involving type introspection and reflection, such as in serialization frameworks, dependency injection engines, and during type-safe cast operations. By integrating the techniques discussed, enhance your Java applications with dynamic capabilities that adapt to varying runtime conditions.
No comments yet.