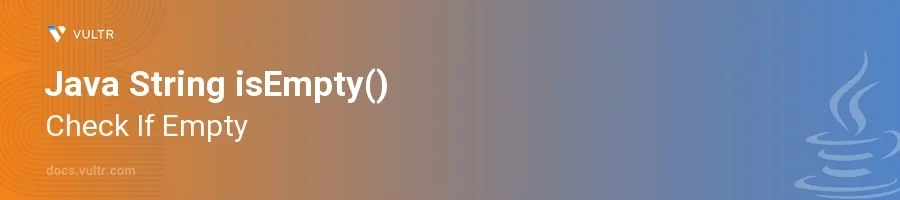
Introduction
The isEmpty()
method in Java is a straightforward and efficient way for checking whether a given string has a length of zero, which means it is an empty string. This method is part of the String class in Java's standard library, making it readily accessible for string manipulations and checks. It plays a critical role in validation processes where determining whether a string is empty can dictate the flow of program logic, such as in form validations or processing user inputs.
In this article, you will learn how to leverage the Java String isEmpty()
method across various scenarios. Discover how to use this function to improve input validation, refine data processing, and simplify condition checks in your Java applications.
Understanding the isEmpty() Method
Before diving deeper into the applications, it's crucial to understand the basic usage and behavior of the isEmpty()
method. This method returns a boolean value, true
if the length of the string is zero, and false
otherwise. It's a non-static method, meaning it is called on an instance of the String class.
Basic Usage
Consider a string and you want to check if it is empty.
Call the
isEmpty()
method on the string instance.javaString myString = ""; boolean result = myString.isEmpty(); System.out.println("Is empty: " + result);
Here,
myString
is an empty string. When theisEmpty()
method is called, it returnstrue
, indicating that the string is indeed empty.
Comparison with Length Method
Note that determining string emptiness can also be done using the
length()
method.Understand that while
isEmpty()
internally checks if the string's length is zero, using it makes the code more readable than checking length explicitly.javaString anotherString = "content"; boolean isEmptyCheck = anotherString.isEmpty(); // More readable boolean isLengthZeroCheck = anotherString.length() == 0; // Less readable System.out.println("Is empty using isEmpty(): " + isEmptyCheck); System.out.println("Is empty using length(): " + isLengthZeroCheck);
In this example,
isEmpty()
makes the code more intuitive and focused on the purpose, enhancing readability, whilelength() == 0
serves the same purpose but might divert attention to string length considerations.
Practical Applications of isEmpty()
Using isEmpty()
can simplify many tasks in Java programming by making condition checks cleaner and clearer. Here are some scenarios where isEmpty()
proves beneficial:
Form Validation
Assume you are validating user input in a form where fields must not be empty.
Use
isEmpty()
to check each input field.javaString username = getUserInput(); if (username.isEmpty()) { System.out.println("Username is required!"); } else { System.out.println("Username accepted."); }
This code snippet checks if the
username
string is empty and provides feedback accordingly, crucial for avoiding processing of invalid or empty inputs.
Decision Making in Data Processing
Handle input data where operations depend on whether strings are empty or not.
Employ
isEmpty()
to conditionally process data only when strings are not empty.javaString data = fetchData(); if (!data.isEmpty()) { processData(data); } else { System.out.println("No data to process."); }
Use
isEmpty()
to check if thedata
string is empty before proceeding with processing, optimizing resources by skipping unnecessary operations.
Streamlining Conditional Structures
Improve readability of conditions involving string checks.
Integrate
isEmpty()
in logical conditions to streamline code and reduce complexity.javaString message = loadMessage(); // Instead of using message.length() > 0 if (!message.isEmpty()) { display(message); } else { display("No new messages."); }
This example demonstrates how
isEmpty()
can replace length checks for simplicity and enhanced code clarity.
Conclusion
The isEmpty()
method in Java is a vital tool for efficiently checking if a string is empty. It not only aids in writing clear and concise code but also ensures that operations involving string manipulations are performed only when meaningful. By incorporating the isEmpty()
method in various common programming tasks, such as data validation, decision-making processes, and enhancing the clarity of conditional structures, you elevate the robustness and readability of your Java applications. Adapt the strategies and examples presented to streamline and improve your coding practices effectively.
No comments yet.