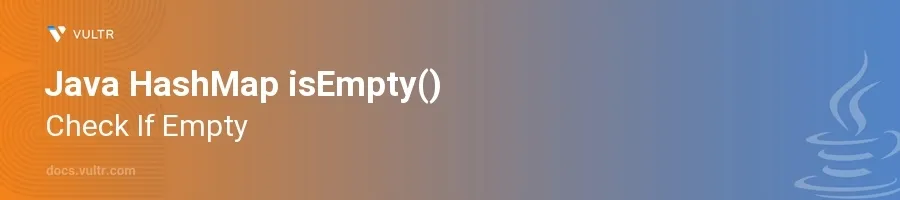
Introduction
The isEmpty()
method in Java's HashMap
class is a straightforward yet powerful tool for checking whether a map contains any key-value pairs. This method becomes especially valuable when you need to ensure that a map is not processed further if it's empty, helping avoid unnecessary computation and potential errors in your code.
In this article, you will learn how to use the isEmpty()
method to check the emptiness of a HashMap
. You will also discover scenarios where this check is particularly useful, reinforcing your ability to manage collections efficiently in Java.
Understanding isEmpty() Method
The isEmpty()
method is a part of the Map
interface and is implemented by the HashMap
class. It returns true
if the map contains no key-value mappings, and false
otherwise.
Basic Usage of isEmpty()
Create an instance of
HashMap
.Use
isEmpty()
to check if the map is empty.javaHashMap<String, Integer> map = new HashMap<>(); boolean result = map.isEmpty(); System.out.println("Is map empty? " + result);
This code snippet sets up an empty
HashMap
and checks its emptiness. Since no key-value pairs are added tomap
,isEmpty()
returnstrue
.
Testing with a Non-Empty Map
Add some elements to your
HashMap
.Again, apply the
isEmpty()
method to see the result.javaHashMap<String, Integer> map = new HashMap<>(); map.put("A", 1); map.put("B", 2); boolean result = map.isEmpty(); System.out.println("Is map empty? " + result);
Here, after inserting elements into the map,
isEmpty()
evaluates tofalse
as there are now key-value pairs within the map.
Practical Scenarios to Use isEmpty()
isEmpty()
finds its importance in scenarios where actions depend on whether the map is populated. Some typical use cases include:
Preventing Errors
Check if data is present before processing.
javaif (map.isEmpty()) { System.out.println("No data to process."); } else { // Process map data }
Avoid process-related errors by confirming the presence of data in your
HashMap
.
Conditional Execution
Vary logic based on the presence of entries.
javaif (!map.isEmpty()) { // Logic when the map is not empty System.out.println("Processing data."); }
This ensures that specific parts of your code execute only when the map contains data, optimizing performance.
Conclusion
The isEmpty()
method in Java's HashMap
is integral to efficiently managing workflows that depend on the presence or absence of data within maps. By using this method to check for emptiness, you prevent unnecessary computations and reduce the chance of errors due to unexpected empty data structures. Integrate this check into your Java applications to enhance reliability and code clarity, particularly when handling large or critical datasets.
No comments yet.