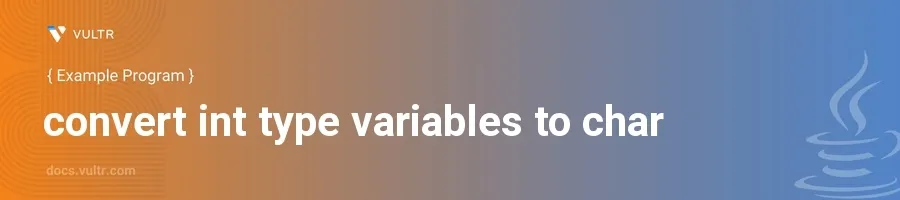
Introduction
Conversion between data types is a common task in programming. In Java, converting data types like integers to characters can be instrumental in scenarios where you need to manipulate data at a more granular level, like dealing with ASCII values or performing character arithmetic.
In this article, you will learn how to efficiently convert int
type variables to char
in Java through practical examples. Understand the nuances of such conversions and see how different methods can be applied in real-world programming tasks.
Direct Conversion Using Casting
Convert an Integer to Character by Casting
Declare an integer variable.
Convert the integer to a char using casting.
javaint numericalValue = 65; char character = (char) numericalValue; System.out.println("The character for ASCII 65 is: " + character);
This example converts the integer
65
, which is the ASCII value forA
, to the corresponding character by using casting. The output will be:The character for ASCII 65 is: A
.
Using Character Methods
Convert Using Character.toString Method
Understand that sometimes you might need to convert the char back to a String for certain operations.
Convert the integer to a char, then to a String.
javaint numericalValue = 77; char character = (char) numericalValue; String charString = Character.toString(character); System.out.println("The character for ASCII 77 is: " + charString);
In this code snippet, the integer
77
is first cast to a char (which corresponds toM
) and then converted to a String usingCharacter.toString()
. The output will be:The character for ASCII 77 is: M
.
Conclusion
Converting integers to characters in Java is a straightforward process that primarily involves casting. This technique is useful when dealing with ASCII values, character manipulation, or when character data representation is required from numeric values. By mastering int to char conversion, you enhance your capability to handle diverse data types and improve your data processing skills in Java applications.
No comments yet.