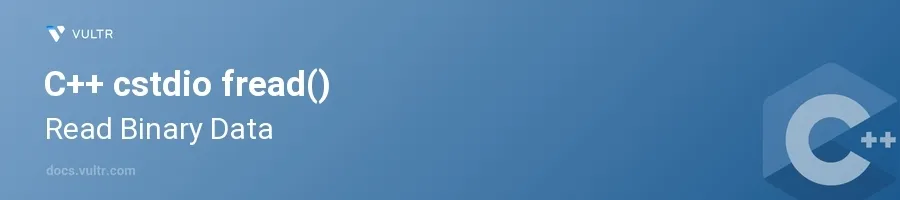
Introduction
The fread()
function in C++ is a standard library function commonly used to read blocks of binary data from a file. This function is indispensable in scenarios where you have to deal with files in binary format, such as images, videos, or custom binary file types. Understanding how to use fread()
effectively is essential for tasks ranging from simple data loading to complex file manipulation operations.
In this article, you will learn how to use the fread()
function to perform binary data reading operations in C++. Explore the function's parameters, its typical use cases, and how to manage various outcomes related to file handling.
Understanding fread() in C++
Basic Usage of fread() in C++
Include the necessary header files.
Open a file in binary mode.
Use
fread()
to read the data.cpp#include <cstdio> #include <iostream> int main() { FILE *filePointer; char buffer[128]; filePointer = fopen("example.bin", "rb"); if (filePointer == nullptr) { std::cerr << "Failed to open file.\n"; return -1; } size_t bytesRead = fread(buffer, sizeof(char), sizeof(buffer), filePointer); fclose(filePointer); std::cout << "Bytes Read: " << bytesRead << std::endl; return 0; }
This code snippet opens a binary file named
example.bin
and reads the binary file up to 128 bytes. It uses thefread()
function, which returns the number of items successfully read. The example checks if the file is not opened successfully and handles the error by displaying a message.
Handling Errors in fread()
Check the return of
fopen()
to ensure the file opened successfully.Use the return value of
fread()
to determine how many bytes were successfully read.Check for end of file (EOF) or error during the reading process.
cppif (feof(filePointer)) { std::cout << "End of file reached." << std::endl; } else if (ferror(filePointer)) { std::cerr << "Error reading the file." << std::endl; }
After reading, it is crucial to determine whether the end of the file has been reached or if an error occurred. The
feof()
function checks for the end-of-file indicator, whereasferror()
checks for errors.
Advanced Binary File Reading Techniques Using fread() in C++
Using fread() to Read Structured Binary Data
Define a struct that matches the binary data structure.
Read the data directly into the struct for easy access.
cppstruct MyData { int id; float value; }; MyData data; size_t result = fread(&data, sizeof(MyData), 1, filePointer);
Here,
fread()
can read directly into an instance ofMyData
, allowing for structured access to the binary data. This approach is particularly useful for reading serialized data structures.
Conclusion
The fread()
function offers a powerful way to read binary data from files in C++. By understanding how to open files, read data, and check for errors, you can handle binary file inputs efficiently in your programs. Ensure consistency in data reads by carefully managing file pointers and data buffers. With the examples and techniques provided, you can implement robust file reading operations in your C++ applications.
No comments yet.