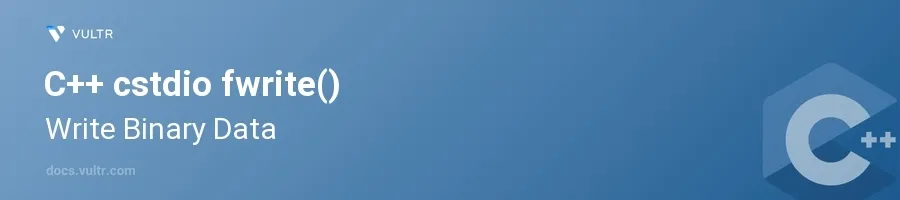
Introduction
The fwrite()
function in C++ is a vital tool for handling binary data writing to files. It's part of the C standard input and output library, and offers a straightforward method for writing blocks of data directly to a file. This functionality is crucial for tasks that require precise control over how data is recorded, such as when dealing with images, custom file formats, or serialized data.
In this article, you will learn how to effectively use the fwrite()
function to write binary data to files in C++. You'll see examples that demonstrate how to setup and use fwrite()
correctly, ensuring data integrity and efficient file handling.
Understanding fwrite()
The fwrite()
function writes a specified number of items, of a designated size, to a given stream. The prototype of fwrite()
is as follows:
size_t fwrite(const void* ptr, size_t size, size_t count, FILE* stream);
ptr
: Pointer to the data array that holds the items to be written.size
: Size of each item in bytes.count
: Number of items to be written.stream
: Pointer to aFILE
object that identifies the output file.
Writing Simple Data Types
Open a file where binary data will be written.
Define data you wish to write.
Use
fwrite()
to write binary data to the file.cpp#include <cstdio> int main() { FILE *file = fopen("example.bin", "wb"); if (file == nullptr) return -1; int numbers[] = {1, 2, 3, 4, 5}; size_t elementsWritten = fwrite(numbers, sizeof(int), 5, file); fclose(file); return 0; }
In this example, an array of integers is written to a binary file named
example.bin
. Thefwrite()
function writes five integers to the file.
Writing Composite Data Structures
Define a struct to represent a complex data type.
Write instances of this struct to a file using
fwrite()
.cpp#include <cstdio> struct Student { char name[50]; int age; float marks; }; int main() { Student student = {"John Doe", 20, 72.5}; FILE *file = fopen("student.bin", "wb"); if (file == nullptr) return -1; fwrite(&student, sizeof(student), 1, file); fclose(file); return 0; }
This snippet writes the data of a
Student
struct to a file. Here,sizeof(student)
ensures that the entire struct is written correctly.
Handling Errors in fwrite()
fwrite()
returns the number of items written successfully. If it's less than the number specified in count
, either an error occurred or the end of the file was reached. Check this return value to ensure data integrity:
Write data to a file.
Check the result to confirm all data has been written.
cppFILE *file = fopen("output.bin", "wb"); if (!file) { perror("File opening failed"); return -1; } int data[] = {10, 20, 30}; size_t result = fwrite(data, sizeof(int), 3, file); if (result != 3) { perror("Failed to write all data"); fclose(file); return -1; } fclose(file);
Here, the code checks if the function wrote all three integers. If not, it handles the error by reporting it and exiting the program.
Conclusion
Using fwrite()
in C++ to write binary data is essential for applications that need direct control over data storage. Whether you're dealing with simple data types or complex structures, fwrite()
provides a reliable way to handle binary file output. By ensuring you check the number of items written, you can maintain data integrity and handle errors effectively in your code. This technique is fundamental for any C++ programmer working with file I/O at a low level.
No comments yet.