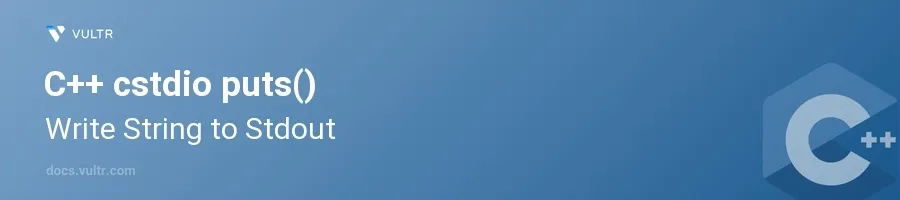
Introduction
The puts()
function from the C++ cstdio library is a straightforward and efficient way to write strings to the standard output (stdout), usually the terminal or console screen. This function automatically appends a newline character at the end of the string, which simplifies the process of printing text with line breaks.
In this article, you will learn how to effectively use the puts()
function in various programming situations. Explore its practical applications, understand its behavior, and discover how it differs from other output functions like printf()
.
Basics of puts()
Simple String Output
Include the cstdio library.
Utilize the
puts()
function to print a string.cpp#include <cstdio> int main() { puts("Hello, world!"); return 0; }
This example demonstrates how to print "Hello, world!" to the console.
puts()
automatically adds a newline after the string.
Comparison with printf()
Recognize that
puts()
is simpler for plain string outputs compared toprintf()
.Implement an example using
printf()
for the same output.cpp#include <cstdio> int main() { printf("Hello, world!\n"); return 0; }
While
printf()
can handle formatted strings and variable substitution,puts()
is more efficient for straightforward string writing because it reduces syntactic overhead.
Error Handling in puts()
Checking for Errors
Understand that
puts()
returns a non-negative number on success and EOF on failure.Write a code snippet that checks for errors when using
puts()
.cpp#include <cstdio> int main() { if (puts("Hello, error checking!") == EOF) { perror("Error writing to stdout"); } return 0; }
This check ensures you handle situations where writing to stdout might fail, perhaps due to redirection errors or when outputting to a closed stream.
Advanced Uses of puts()
Integrating with Control Structures
Use
puts()
within loops or conditions to manage multiple lines of output effectively.Demonstrate outputting multiple lines through a loop.
cpp#include <cstdio> int main() { for(int i = 1; i <= 5; i++) { puts("This is a repeated line."); } return 0; }
This loop prints the string "This is a repeated line." five times, each on a new line, utilizing the inherent newline addition that
puts()
provides.
Conclusion
The puts()
function in C++ is a valuable tool for quickly printing strings to stdout, especially when dealing with simple text outputs that require new lines. Its simplicity offers clear advantages over more complex functions like printf()
when no formatted output is necessary. By incorporating the puts()
function and handling errors appropriately, your C++ code can efficiently manage outputs to the console, maintaining readability and function. Experiment with different scenarios to fully leverage puts()
in your C++ projects.
No comments yet.