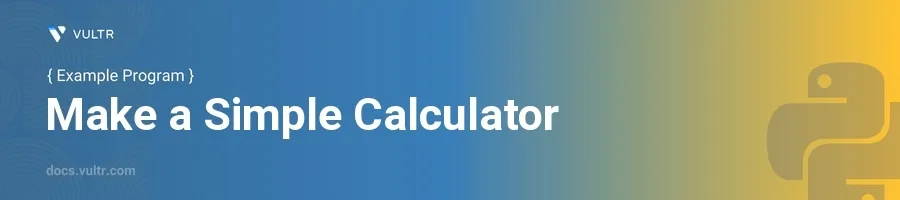
Introduction
A simple calculator is a fundamental project to start with when you are learning a programming language like Python. It allows you to apply basic programming concepts such as functions, loops, and conditional statements, and also provides a clear understanding of handling user input and performing arithmetic operations.
In this article, you will learn how to create a basic calculator using Python. Dive into step-by-step examples that outline a variety of operations such as addition, subtraction, multiplication, and division, along with how to manage user inputs and display outputs.
Building a Simple Calculator
Start with Function Definitions
Define the basic arithmetic functions: addition, subtraction, multiplication, and division. Each function takes two parameters and returns the result after performing the arithmetic operation.
pythondef add(x, y): return x + y def subtract(x, y): return x - y def multiply(x, y): return x * y def divide(x, y): return x / y
These functions are straightforward:
add
function returns the sum ofx
andy
.subtract
function returns the difference betweenx
andy
.multiply
function returns the product ofx
andy
.divide
function returns the quotient ofx
divided byy
.
Handle User Input
Create a function to handle user input. Make sure to convert the inputs to
float
for arithmetic operations and handle any potential exceptions (like dividing by zero or inputting non-numeric values).pythondef get_input(): while True: try: num1 = float(input("Enter first number: ")) num2 = float(input("Enter second number: ")) return num1, num2 except ValueError: print("Please enter valid numbers.")
This loop continues until the user provides valid inputs. The function uses exception handling to catch
ValueError
if the input cannot be converted to a float.
Implementing the Main Loop
Create the main driver function of your calculator that uses a loop to perform operations based on user choice until the user decides to exit.
pythondef calculator(): while True: print("Operations:\n1. Addition\n2. Subtraction\n3. Multiplication\n4. Division\n5. Exit") choice = input("Select operation (1/2/3/4/5): ") if choice == '5': print("Exiting Calculator...") break num1, num2 = get_input() if choice == '1': print("Result: ", add(num1, num2)) elif choice == '2': print("Result: ", subtract(num1, num2)) elif choice == '3': print("Result: ", multiply(num1, num2)) elif choice == '4': if num2 == 0: print("Error: Cannot divide by zero.") else: print("Result: ", divide(num1, num2)) else: print("Invalid Choice! Please select a valid operation.")
- This function allows users to choose an operation by entering a selection number.
- It checks if the user wants to exit the loop when
choice
is '5'. - It uses conditions to determine which arithmetic function to call based on input.
- A division by zero error is specifically checked and handled.
Validator for User Decisions
Optionally, add a validator function to ensure the choices made by the user are acceptable before proceeding with calculations.
pythondef validate_choice(choice): return choice in ['1', '2', '3', '4', '5']
This function ensures that the user's choice is among the predefined options.
Conclusion
Develop a Python program to create a simple calculator that accepts user inputs, performs basic calculations, and outputs the results. Start by defining functions for each arithmetic task. Manage user inputs safely and loop through operations effectively. Implement validation to ensure user choices are correct before computations. This exercise strengthens understanding of Python’s basic constructs and how to apply them in creating functional applications. Enjoy coding and testing your basic calculator to explore more features and enhancements.
No comments yet.