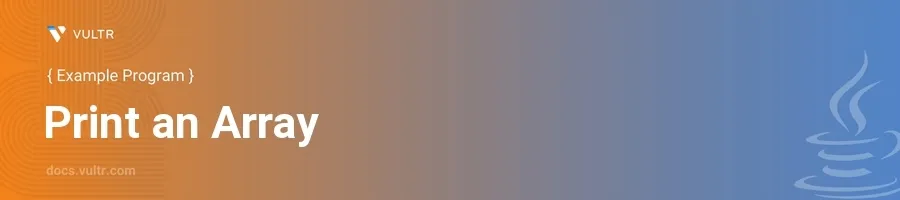
Introduction
In Java, arrays store fixed-size sequential collections of elements of the same type. Printing arrays is a fundamental task that is necessary for debugging and displaying sequential data effectively in applications. Arrays can hold primitives (like int, char, etc.) or objects (like Strings or other Java objects).
In this article, you will learn how to print arrays in Java using different methods. Explore practical examples that include using loops, Java 8 Streams, and the Arrays
utility class. These approaches can be applied to any type of array, enhancing your ability to manipulate and display array data. This guide on Java printing arrays will help you understand efficient techniques for handling array output.
Printing an Array in Java Using Loops
Print an Integer Array
Define an array of integers.
Use a for-loop to iterate through the array and print each element.
javaint[] array = {10, 20, 30, 40, 50}; for (int i = 0; i < array.length; i++) { System.out.print(array[i] + " "); }
This code iterates through each element in the array
array
and prints it followed by a space.
Print a String Array
Create a String array.
Employ an enhanced for-loop (for-each loop) to print each element.
javaString[] colors = {"Red", "Blue", "Green", "Yellow"}; for (String color : colors) { System.out.print(color + " "); }
In this example, the for-each loop simplifies iterating through the
colors
array, printing each color followed by a space.
Utilizing Java 8 Streams for Arrays
Print an Array with Stream
Import the
java.util.Arrays
andjava.util.stream.Stream
classes.Convert the array into a stream and utilize the
forEach
method to print elements.javaimport java.util.Arrays; import java.util.stream.Stream; Integer[] numbers = {1, 2, 3, 4, 5}; Stream.of(numbers).forEach(n -> System.out.print(n + " "));
Here,
Stream.of(numbers)
creates a stream from the array, andforEach
is used to execute a print operation for each element in the stream.
Using Arrays.toString() and Arrays.deepToString()
Print Arrays with Arrays.toString()
Import the
java.util.Arrays
class.Use the
Arrays.toString()
method to convert the array into a printable string format.javaimport java.util.Arrays; int[] numArray = {5, 10, 15, 20}; System.out.println(Arrays.toString(numArray));
Arrays.toString(numArray)
returns a string representation of thenumArray
array enclosed in brackets and separated by commas.
Handle Multidimensional Arrays
For multidimensional arrays, use
Arrays.deepToString()
.javaimport java.util.Arrays; int[][] matrix = {{1, 2}, {3, 4}}; System.out.println(Arrays.deepToString(matrix));
Arrays.deepToString(matrix)
efficiently prints nested arrays, showing the structure and values clearly.
Conclusion
Printing arrays in Java is a versatile skill that aids in debugging and output formatting in a variety of applications. From simple loops for individual display to using Java streams or utility methods from the Arrays
class, the options are robust. Implement these methods to improve the readability and debugging capabilities of your Java applications, ensuring clear and efficient display of array contents. With these techniques in your toolkit, handle arrays with ease, regardless of their complexity or depth.
No comments yet.