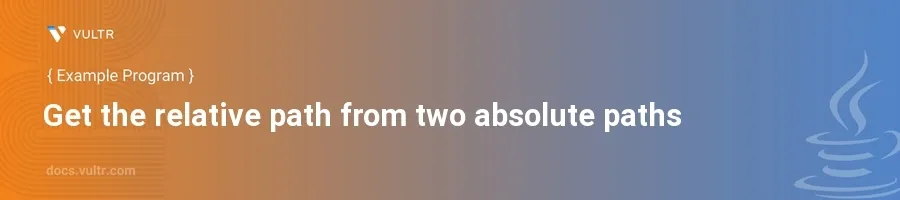
Introduction
Handling file paths efficiently is a critical skill when working with Java file I/O operations. At times you need to find the relative path between two absolute paths, a scenario common in applications managing files across different directories. This involves determining the path you need to traverse from one file location to another.
In this article, you will learn how to derive relative paths from two absolute paths using Java. The following sections explain mapping out common path scenarios and applying Java's Path
and Paths
classes from the java.nio.file
package to implement this.
Understanding the Use of Path and Paths
Overview of Path and Paths Classes
- Recognize the purpose of the
Path
class. - Become familiar with the
Paths
method.
The Path
class represents a file path in the Java File System, which can denote a directory or a file. The Paths
class contains static methods that are used to return a Path
by converting a string or URI.
Example: Create Path Instances
Import needed classes.
javaimport java.nio.file.Path; import java.nio.file.Paths;
Utilize
Paths.get(String)
to createPath
instances.javaPath path1 = Paths.get("/home/user/Documents"); Path path2 = Paths.get("/home/user/Documents/Reports");
This example creates two path instances from string paths. These paths are often the input for further operations, such as finding the relative path.
Calculating Relative Path between Two Paths
Basic Calculation of Relative Path
- Comprehend the concept of a relative path.
- Use the
relativize
method.
The method relativize(Path other)
in the Path
class computes the relative path between the path it’s called on and another path.
Simple Relative Path Example
Implement the
relativize
method to calculate the relative path.javaPath path1 = Paths.get("/home/user/Documents"); Path path2 = Paths.get("/home/user/Documents/Reports"); Path relativePath = path1.relativize(path2); System.out.println("Relative Path from path1 to path2: " + relativePath);
The relative path from
path1
topath2
is determined here. Running the above code outputsReports
, indicating thatReports
is a directory insideDocuments
.
More Complex Example Involving Non-Common Paths
Prepare paths that do not have a direct parent-child relationship.
Apply the
relativize
method for complex paths.javaPath path3 = Paths.get("/home/user/Documents"); Path path4 = Paths.get("/home/user/Photos/2022"); Path relativePath2 = path3.relativize(path4); System.out.println("Relative Path from path3 to path4: " + relativePath2);
This code calculates the relative path where moving from
Documents
toPhotos/2022
involves going up one directory and then down into the Photos directory and further into 2022.When you run this code, it outputs
../Photos/2022
, which shows the necessary directory navigation fromDocuments
to2022
underPhotos
.
Advanced Usage: Handling Errors and Absolute Paths
Potential Errors with Relative Path Calculation
- Understand the importance of using absolute paths.
- Identify possible errors when using relative paths improperly.
Using relative paths incorrectly, especially between paths that do not share a common root, can lead to errors. It's crucial to ensure that the paths are absolute and normalized before calculating their relative paths.
Ensuring Paths are Absolute
Check and convert paths to absolute paths.
Use normalization to clear out any redundant elements.
javaPath absolutePath1 = Paths.get("/home/user/Documents").toAbsolutePath().normalize(); Path absolutePath2 = Paths.get("/home/user/Photos/2022").toAbsolutePath().normalize(); Path robustRelativePath = absolutePath1.relativize(absolutePath2); System.out.println("Robust Relative Path: " + robustRelativePath);
This approach ensures that both paths are absolute and normalized, avoiding potential pitfalls in path calculation.
Conclusion
Deriving a relative path from two absolute paths in Java is a straightforward process when using the Path
class methods provided in the java.nio.file
package. By understanding and applying the relativize
method, you can efficiently compute relative paths, handling even complex directory structures reliably. Ensure paths are absolute and normalized to avoid common errors, and leverage these Java capabilities to manage file directories effectively, enhancing your Java applications' file handling robustness.
No comments yet.