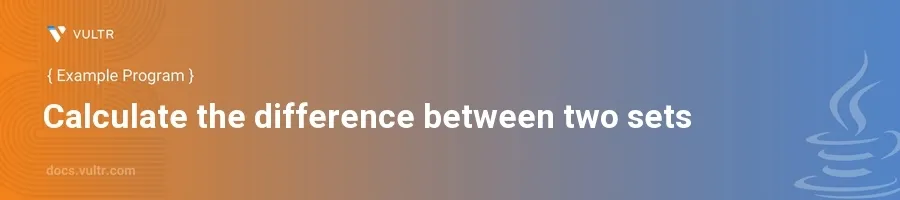
Introduction
Java provides various ways to manipulate sets, including methods to calculate the difference between two sets, also known as the relative complement. Understanding how to perform this operation is crucial for handling collections that require comparison or exclusion of certain elements based on a given criterion.
In this article, you will learn how to calculate the difference between two sets in Java. You will explore different approaches using Java's Set interface and its common implementations like HashSet and TreeSet. Various examples will demonstrate practical applications to help you understand how to implement these techniques efficiently in your Java projects.
Basic Set Difference Using HashSet
Using removeAll()
Method
Initialize two
HashSet
instances with some sample elements.Use the
removeAll()
method to calculate the difference.javaHashSet<Integer> set1 = new HashSet<>(Arrays.asList(1, 2, 3, 4, 5)); HashSet<Integer> set2 = new HashSet<>(Arrays.asList(4, 5, 6, 7, 8)); set1.removeAll(set2); System.out.println("Difference (set1 - set2): " + set1);
This code snippet initializes two sets,
set1
andset2
, with differing elements. ApplyingremoveAll()
toset1
withset2
as the argument removes all elements fromset1
that are also inset2
. The remaining elements inset1
represent the difference betweenset1
andset2
.
Determine Difference in Both Directions
Start by creating two separate
HashSet
objects with different elements.Use the
removeAll()
method for both combinations to get differences in both directions.javaHashSet<Integer> originalSet1 = new HashSet<>(Arrays.asList(1, 2, 3, 4, 5)); HashSet<Integer> originalSet2 = new HashSet<>(Arrays.asList(4, 5, 6, 7, 8)); HashSet<Integer> difference1 = new HashSet<>(originalSet1); difference1.removeAll(originalSet2); HashSet<Integer> difference2 = new HashSet<>(originalSet2); difference2.removeAll(originalSet1); System.out.println("Difference (set1 - set2): " + difference1); System.out.println("Difference (set2 - set1): " + difference2);
This variation demonstrates calculating the set difference in both directions:
set1 - set2
andset2 - set1
. This is useful for cases where the relationship between the two sets needs full evaluation.
Set Difference Using TreeSet
Ordered Set Difference
Initialize
TreeSet
instances to benefit from sorted order.Apply the
removeAll()
method similarly to how it's done withHashSet
.javaTreeSet<Integer> set1 = new TreeSet<>(Arrays.asList(10, 20, 30, 40, 50)); TreeSet<Integer> set2 = new TreeSet<>(Arrays.asList(30, 40, 60, 70, 80)); set1.removeAll(set2); System.out.println("Difference (set1 - set2): " + set1);
Using a
TreeSet
instead ofHashSet
ensures that the elements in the resulting set are sorted. This can be especially useful when order matters, such as when preparing a dataset for presentation or further ordered operations.
Bi-directional Ordered Differences
Create
TreeSet
objects for both sets.Calculate the differences in both directions using the
removeAll()
method.javaTreeSet<Integer> treeSet1 = new TreeSet<>(Arrays.asList(10, 20, 30, 40, 50)); TreeSet<Integer> treeSet2 = new TreeSet<>(Arrays.asList(30, 40, 60, 70, 80)); TreeSet<Integer> treeDiff1 = new TreeSet<>(treeSet1); treeDiff1.removeAll(treeSet2); TreeSet<Integer> treeDiff2 = new TreeSet<>(treeSet2); treeDiff2.removeAll(treeSet1); System.out.println("Difference (set1 - set2): " + treeDiff1); System.out.println("Difference (set2 - set1): " + treeDiff2);
This example illustrates how to perform the operation in a way that keeps results ordered using
TreeSet
. This helps when working with data that benefits from natural ordering, such as numeric or alphabetical sets.
Conclusion
Calculating the difference between two sets in Java can be performed cleanly using Java's powerful collection framework, particularly the Set interface. Whether choosing HashSet
for speed and uniqueness without order or TreeSet
for maintaining a natural order, the removeAll()
method offers a straightforward approach to determine elements unique to one set. By mastering these techniques, ensure that data manipulation involving sets is both efficient and adaptable to various application requirements.
No comments yet.