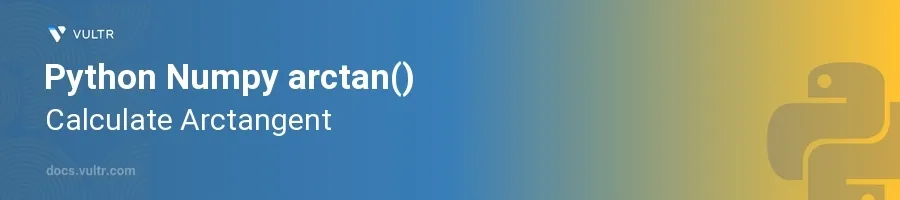
Introduction
The arctan()
function in the NumPy library is a mathematical tool used to calculate the arctangent of an element-wise manner in arrays. Arctangent is one of the trigonometric functions that gives the angle whose tangent is the input value. This function proves very useful in fields like engineering, physics, and computer graphics, where angle determination is essential in computations.
In this article, you will learn how to utilize NumPy's arctan()
function to compute the arctangent of both single numbers and arrays. Techniques for handling real-world data types and understanding the output in terms of radians will also be explored.
Basic Usage of arctan()
Calculating the Arctangent of a Single Value
Import the NumPy library.
Pass a numeric value to
np.arctan()
.pythonimport numpy as np angle_rad = np.arctan(1) print("Arctangent of 1 is:", angle_rad)
This code calculates the arctangent of 1, which is π/4 radians, or approximately 0.785 radians.
Applying arctan() to a Range of Values
Utilize NumPy's array creation facility to generate an array of values.
Apply the
arctan()
function to the entire array.pythonimport numpy as np values = np.array([-1, 0, 1]) angles_rad = np.arctan(values) print("Arctangent of array values:", angles_rad)
The function computes the arctangent of each element in the array
values
, returning an array of radians.
Advanced Applications
Angle Conversion from Radians to Degrees
Compute the arctangent which results in radians.
Convert the result from radians to degrees using
np.degrees()
.pythonimport numpy as np value = 1 angle_rad = np.arctan(value) angle_deg = np.degrees(angle_rad) print("Angle in degrees:", angle_deg)
After calculating the arctangent of the value, which gives radians, convert this result to degrees for more intuitive angle measurements often used in various applications.
Handling Complex Numbers
Pass a complex number to the
arctan()
function.Analyze the output, noting how NumPy handles complex inputs.
pythonimport numpy as np complex_value = 1 + 1j angle_rad = np.arctan(complex_value) print("Arctangent of complex number:", angle_rad)
When a complex number is provided,
arctan()
gracefully handles the computation, extending its applicability beyond simple real number operations.
Conclusion
NumPy's arctan()
function is a versatile tool for computing the arctangent of numbers and arrays in Python. By transforming these values into radians (or degrees), it supports various scientific, engineering, and graphical computations. Whether dealing with simple scenarios or complex numbers, mastering arctan()
enhances your mathematical toolkit in Python, making it easier to handle trigonometric calculations effectively and efficiently.
No comments yet.