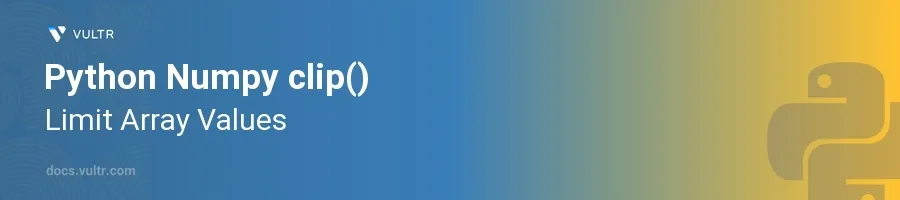
Introduction
The clip()
function in Python's NumPy library is an essential tool for managing numerical arrays, particularly when you need to limit the range of values to a specific minimum and maximum. This function ensures that all elements in an array fall within a given interval, effectively clipping outliers. It's widely utilized in data preprocessing, image processing, and anywhere data normalization is required.
In this article, you will learn how to leverage the clip()
function to control the range of your data arrays. Explore different scenarios where this function can be particularly useful, including handling outliers and maintaining data within defined boundaries for further analysis or visualization.
Understanding the clip() Function
Basic Usage of clip()
Import NumPy and create an array.
Apply the
clip()
method to restrict its values within a specified range.pythonimport numpy as np data = np.array([1, 2, 3, 10, 20, 30]) clipped_data = data.clip(2, 10) print(clipped_data)
This code sets a lower limit of
2
and an upper limit of10
. Values less than2
are set to2
, and those greater than10
are set to10
. The output array will be[2, 2, 3, 10, 10, 10]
.
Clipping Data Based on Dynamic Thresholds
Sometimes, you need thresholds that aren't static but are derived from the data itself, such as using percentiles.
Use the
percentile()
function from NumPy to determine dynamic clipping thresholds.pythondata = np.random.randn(100) # Generate 100 random numbers lo, hi = np.percentile(data, [5, 95]) # Get 5th and 95th percentiles clipped_data = data.clip(lo, hi) print(clipped_data)
This snippet calculates the 5th and 95th percentiles of a dataset and uses these as the bounds for the
clip()
method. This method is effective in ensuring that extreme outliers are mitigated.
Using clip() in Multidimensional Arrays
Clipping Values in 2D Arrays
Apply
clip()
to each element in multidimensional arrays, such as matrices used in image processing.Initialize a 2D array and apply the
clip()
function.pythonimport numpy as np matrix = np.array([[1, 20, 3], [4, 5, 60], [70, 8, 9]]) clipped_matrix = matrix.clip(3, 9) print(clipped_matrix)
Here, every element less than
3
becomes3
, and those greater than9
become9
, resulting in a new clipped matrix. Such operations are crucial, for example, in adjusting image pixel values.
Conclusion
Utilizing the clip()
function in NumPy effectively limits the range of your numerical data, ensuring values stay within a desired boundary. This capability is particularly beneficial for data normalization, preprocessing before machine learning, or even adjusting image data for better visualization. By experimenting with both static and dynamic clipping thresholds, as well as applying clipping to multidimensional arrays, you can maintain control over your data's consistency and quality. Adapt these techniques to ensure your data fulfills its intended analysis or visualization criteria while strengthening the robustness of your computational logic.
No comments yet.