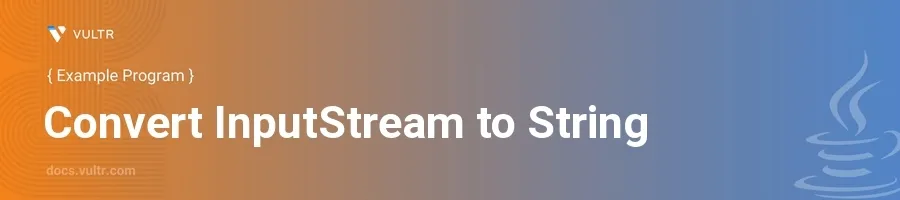
Introduction
When working with Java, a common task you might encounter is converting an InputStream
to a String
. This occurs frequently in scenarios involving file input/output operations, web data fetching, or reading from resources. The InputStream
class is a fundamental part of Java’s IO package and provides a means to read bytes from a source. However, handling these bytes as a string requires some specific steps to convert inputstream to string java effectively.
In this article, you will learn how to convert an InputStream
to a String
using different methods in Java. These will include using standard libraries such as IOUtils
from Apache Commons, Java’s own Scanner
class, and the newer java.nio
package. These inputstream to string methods allow for flexibility depending on your project requirements and the version of Java you are using.
Utilizing the Standard Library
Convert Using Java 8's Standard Charset
Begin by obtaining an
InputStream
. For example, read from a file or a network resource.Use
java.nio.charset.StandardCharsets
andjava.util.Scanner
to convert theInputStream
to aString
.javaInputStream inputStream = new FileInputStream("example.txt"); Scanner scanner = new Scanner(inputStream, StandardCharsets.UTF_8.name()); String text = scanner.useDelimiter("\\A").next(); scanner.close();
This code creates an
InputStream
from a file named "example.txt". TheScanner
reads the entire content of the stream using a delimiter that matches the beginning of the input, effectively reading the whole stream into a singleString
. The stream and scanner are then closed to free resources.
Using Apache Commons IO
Add Apache Commons IO to your project dependencies.
Use
IOUtils.toString
to read the entireInputStream
into aString
.javaInputStream inputStream = new FileInputStream("example.txt"); String result = IOUtils.toString(inputStream, StandardCharsets.UTF_8); inputStream.close();
Here,
IOUtils.toString
method is directly used to convert theInputStream
into aString
specifying the character encoding explicitly. It's crucial to close the stream afterwards to avoid memory leaks.
Java 11 and Above Approach
Utilizing java.nio.file Files Class
With Java 11, leverage the
Files
andPath
classes for a more modern approach.Convert the
InputStream
to aString
using theFiles.readString
after converting the stream to aPath
.javaPath path = Paths.get("example.txt"); String content = Files.readString(path);
This snippet directly reads the contents of a file into a
String
. This method is straightforward and handles the character encoding automatically, making it suitable for simple file-reading scenarios involving input stream to string java conversions.
Conclusion
Converting an InputStream
into a String
in Java can be accomplished through multiple methods, each suitable for different use cases. Whether by utilizing Java's built-in capabilities with Scanner
and java.nio.file
or using external libraries like Apache Commons IO, you now have the tools to select the appropriate approach based on your specific conditions, such as Java version or project requirements. These java inputstream to string techniques can be adapted to fit your needs,, ensuring efficient and effective data handling within your Java applications.
No comments yet.