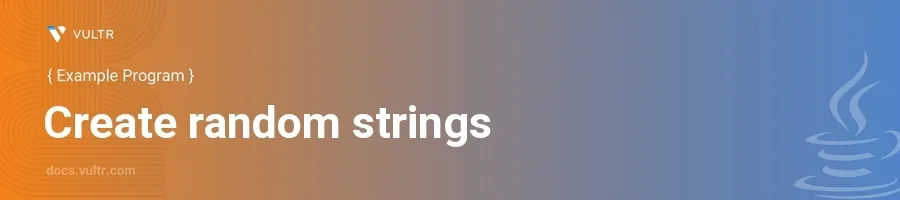
Introduction
Generating random strings is a common requirement in software development for scenarios such as unique identifiers for objects, random test data, or even placeholder text in UI mockups. Java provides several built-in methods and libraries to accomplish this task effectively, adapting to different requirements in terms of string length, character sets, and unpredictability.
In this article, you will learn how to create random strings in Java using various methods. Discover how to leverage the standard Java libraries as well as external libraries to generate strings that fit your specific software development needs. Each method is explained with practical code examples to help you understand the implementation details effectively.
Using Java's Built-in Features
Generate Random Strings with java.util.Random
Import the necessary Java utilities.
Create a method that leverages
java.util.Random
to generate random strings from a specified set of characters.javaimport java.util.Random; public class RandomStringGenerator { public String generateRandomString(int length) { String characterSet = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789"; StringBuilder sb = new StringBuilder(); Random random = new Random(); for (int i = 0; i < length; i++) { int index = random.nextInt(characterSet.length()); sb.append(characterSet.charAt(index)); } return sb.toString(); } }
This code initializes a
StringBuilder
and aRandom
object. It builds a string of the specified length by randomly selecting characters from the predefinedcharacterSet
. The use ofStringBuilder
ensures that the string construction is efficient.
Using Java 8 Streams for Random String Generation
Use Java 8's Stream API in combination with
Random
to create a one-liner method for random string generation.Define a set of characters and generate a random string using functional-style programming.
javaimport java.util.Random; import java.util.stream.Collectors; import java.util.stream.IntStream; public class StreamRandomString { public String generate(int length) { String chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789"; Random rnd = new Random(); return IntStream.generate(() -> chars.charAt(rnd.nextInt(chars.length()))) .limit(length) .collect(StringBuilder::new, StringBuilder::appendCodePoint, StringBuilder::append) .toString(); } }
This approach uses
IntStream.generate()
to produce an infinite stream of random integers, mapping each integer to a character in a set. The stream is then limited to the desired length and collected into aStringBuilder
.
Leveraging External Libraries
Using Apache Commons Lang
Incorporate the Apache Commons Lang library, which includes utilities for generating random strings easily.
Utilize the
RandomStringUtils
class which provides methods to create randomized strings.javaimport org.apache.commons.lang3.RandomStringUtils; public class ExternalLibRandomString { public String generateRandomString(int length) { return RandomStringUtils.randomAlphanumeric(length); } }
The
RandomStringUtils.randomAlphanumeric(int)
method from Apache Commons Lang generates a random string of letters and digits. It's a straightforward method for simple needs where only alphanumeric characters are required. This library handles all the randomness and character selection internally.
Using Google Guava
Add Google Guava to your project dependencies.
Create strings with Guava's
BaseEncoding
to handle more complex string encoding requirements.javaimport com.google.common.io.BaseEncoding; public class GuavaRandomString { public String generateRandomBytesBase64(int byteLength) { byte[] randomBytes = new byte[byteLength]; new Random().nextBytes(randomBytes); return BaseEncoding.base64().encode(randomBytes); } }
This example utilizes Guava's
BaseEncoding
class to encode a byte array into a Base64 string. This method is particularly useful when you need a URL-safe randomly generated string.
Conclusion
Generating random strings in Java can be accomplished through a variety of methods, from simple JDK capabilities to more elaborate solutions using external libraries. Each method serves different purposes and offers different levels of control and flexibility over the output. By selecting the appropriate technique for your needs, you ensure that your software can generate random strings effectively, whether for identifiers, tokens, or test data. Employ these strategies in your Java applications to enhance functionality and maintain robustness.
No comments yet.