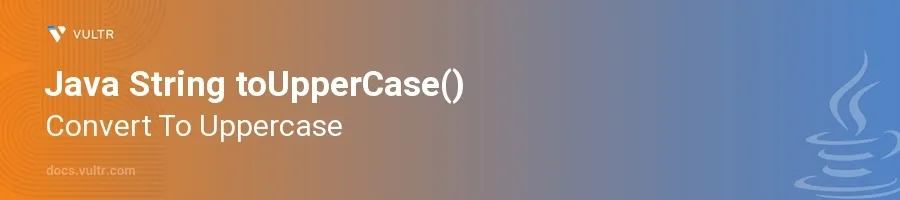
Introduction
The toUpperCase()
method in Java is a fundamental tool for string manipulation, allowing you to convert all characters in a string to uppercase. This method is especially useful in scenarios where consistent case formatting is required, such as user inputs in applications or data preprocessing in software development.
In this article, you will learn how to effectively use the toUpperCase()
method in various contexts. Explore the basic usage of converting strings to uppercase in Java, handling locale-specific conversions, and integrating this method in real-world Java applications. This guide covers everything you need to know about using toUpperCase in Java.
Basic Usage of toUpperCase() in Java
Simple Conversion to Uppercase in java
Start by defining a basic string before using the
toUpperCase()
method to convert the string to uppercase in Java..Apply the
toUpperCase()
method to convert all characters to uppercase.Print the result to see the transformation.
javaString original = "hello world"; String modified = original.toUpperCase(); System.out.println(modified);
This example converts the string
"hello world"
to"HELLO WORLD"
. It demonstrates the straightforward application oftoUpperCase()
to convert any lowercase letters to their uppercase counterparts.
Understanding Case Conversion with Special Characters
Recognize that
toUpperCase()
only affects alphabetic characters.Define a string that includes numbers and symbols along with alphabets.
Convert the string to uppercase and observe the outcome.
javaString mixedContent = "java 123!@#"; String upperCaseContent = mixedContent.toUpperCase(); System.out.println(upperCaseContent);
In this code, alphabetic characters in
"java 123!@#"
are converted to"JAVA 123!@#"
. The numbers and symbols remain unchanged, demonstrating thattoUpperCase()
specifically targets alphabetic elements.
Locale-Specific Uppercase Conversion
Why Locale Matters in toUpperCase()
- Understand that certain languages have specific rules for character casing.
- Using the default
toUpperCase()
might not always adhere to local linguistic rules. - Recognize the importance of using a locale parameter for accuracy in international applications.
Converting with Locale
Import the
Locale
class from thejava.util
package.Create a string in Turkish that includes the dotless 'i'.
Apply
toUpperCase()
with the Turkish locale to correctly convert the string.javaimport java.util.Locale; String strTurkish = "için"; String upperTurkish = strTurkish.toUpperCase(new Locale("tr", "TR")); System.out.println(upperTurkish);
This snippet demonstrates converting
"için"
to"İÇİN"
using the Turkish locale. Notice the uppercase letter 'İ', which adheres to the unique rules of the Turkish alphabet, highlighting the importance of locale intoUpperCase()
conversions.
Practical Applications in Java Projects
Normalizing User Input
Capture user input using a Scanner.
Convert the input string to uppercase to standardize before processing.
Use the converted string for further application logic.
javaimport java.util.Scanner; Scanner scanner = new Scanner(System.in); System.out.println("Enter your name:"); String userName = scanner.nextLine(); String normalizedInput = userName.toUpperCase(); System.out.println("Processed Name: " + normalizedInput);
This example takes user input, converts it to uppercase, and then processes it. This is common in applications where case consistency is needed, such as database entries or user authentication systems.
Making Case-Insensitive Comparisons
Assume the requirement to match strings irrespective of their cases.
Convert both strings involved in the comparison to uppercase.
Compare the uppercase versions for equality.
javaString a = "Java"; String b = "java"; boolean equal = a.toUpperCase().equals(b.toUpperCase()); System.out.println("Are the strings equal? " + equal);
Converting both
"Java"
and"java"
to uppercase results in"JAVA"
for both, facilitating case-insensitive comparison which returnstrue
.
Conclusion
Utilize the toUpperCase()
method in Java to convert strings to uppercase effectively, ensuring your applications handle string cases consistently and correctly. Whether for simple modifications, locale-specific requirements, or practical applications, toUpperCase()
serves as an essential tool in the Java developer's toolkit. By leveraging this method as demonstrated, you help ensure that your Java applications are robust, user-friendly, and adaptable to various language environments.
No comments yet.