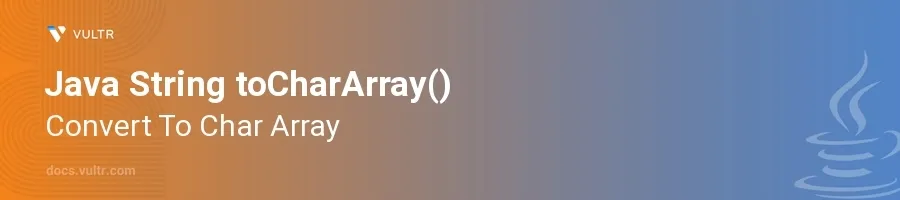
Introduction
In Java, the toCharArray()
method is a quick and convenient way to convert a string into an array of characters. This method is particularly useful when you need to manipulate individual characters of the string or perform operations that require direct access to the underlying character array. For instance, algorithms that process or analyze text often benefit from this conversion.
In this article, you will learn how to effectively utilize the toCharArray()
method to convert strings into character arrays in Java. You will explore different scenarios where this technique is applicable, enhancing your understanding and ability to manipulate string data proficiently.
Understanding toCharArray() Method
The toCharArray()
method belongs to the String
class in Java and is used to convert a given string into a new character array. This method does not take any parameters and returns a char[]
that represents the characters of the string.
Basic Usage of toCharArray()
Define a string you wish to convert.
Use the
toCharArray()
method to convert the string to a character array.javaString greeting = "Hello, World!"; char[] chars = greeting.toCharArray();
This code snippet takes the string
greeting
and converts it into a character arraychars
. Each element of the array represents a single character from the original string.
Analyzing the Returned Character Array
After conversion, iterate through the character array to understand its structure and contents.
javafor (char c : chars) { System.out.println(c); }
This loop prints each character in the
chars
array on a new line, allowing you to see the individual characters that make up the string.
Practical Applications of toCharArray()
The toCharArray()
method is highly useful in scenarios where string manipulation and character inspection are required. Here are several practical applications:
Character Counting
Convert the string to a character array using
toCharArray()
.Use a loop to count occurrences of a specific character.
javaString sentence = "Look for the character frequency."; char[] sentenceChars = sentence.toCharArray(); int count = 0; char toFind = 'e'; for (char c : sentenceChars) { if (c == toFind) { count++; } } System.out.println("Number of '" + toFind + "' in the sentence: " + count);
This example counts how many times the character 'e' appears in the string
sentence
.
Reversing a String
Convert the string to a character array.
Reverse the array manually or using a utility method.
Convert the reversed character array back to a string if needed.
javaString original = "Java"; char[] originalChars = original.toCharArray(); for(int i = 0, j = originalChars.length - 1; i < j; i++, j--) { char temp = originalChars[i]; originalChars[i] = originalChars[j]; originalChars[j] = temp; } String reversed = new String(originalChars); System.out.println("Reversed: " + reversed);
This code takes the string
original
, converts it to an array, reverses the array, and constructs a new string from the reversed array.
Conclusion
The toCharArray()
method is a versatile and powerful tool in Java for string manipulation and analysis. Whether counting specific characters, reversing strings, or performing custom modifications, converting strings to character arrays simplifies many operations on string data. By mastering the toCharArray()
method and related string manipulation techniques, you enhance your capacity to tackle more complex problems in Java programming.
No comments yet.