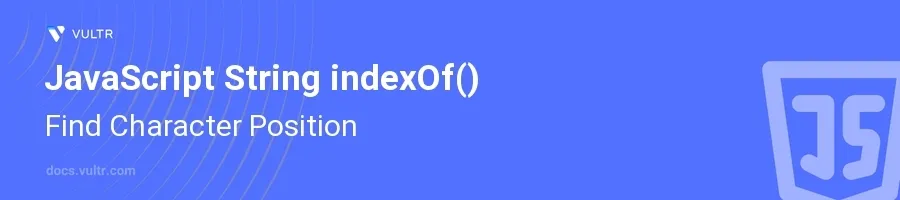
Introduction
The indexOf()
method in JavaScript is a critical tool for finding the position of a substring within a string. This method returns the index of the first occurrence of the specified value, or -1 if the value isn't found. Its straightforward utility makes it essential for tasks like checking the presence of substrings, parsing data, and formatting user input.
In this article, you will learn how to effectively use the indexOf()
method in various scenarios. Discover how to locate a character or substring within a string, handle cases where the substring is not found, and use this method to check the presence of multiple occurrences.
Basic Usage of indexOf()
Finding the position of a single character
Declare a variable containing the string to search.
Use
indexOf()
to find the position of a character.javascriptconst message = "Hello, world!"; const index = message.indexOf('w'); console.log(index);
This code checks for the position of the character
'w'
in the string stored inmessage
. The index7
is returned and logged to the console because 'w' is the eighth character, and index positions start at 0.
Search for Substrings
Define the string and the substring to locate.
Use
indexOf()
to find the starting index of the substring.javascriptconst phrase = "Searching in JavaScript"; const substringIndex = phrase.indexOf("in"); console.log(substringIndex);
Here, the
indexOf()
looks for the substring "in" in thephrase
. It returns12
, which is the index where the substring begins.
Handling Substrings Not Found
Check if a substring does not exist
Perform a search where the substring is not within the string.
Evaluate the result to manage cases where the substring is missing.
javascriptconst text = "No such substring here"; const searchResult = text.indexOf("absent"); console.log(searchResult);
In this snippet, since the substring "absent" is not in the
text
,indexOf()
returns-1
.
Using indexOf() to Avoid Errors
Ensuring substring existence before further processing
Use the return value of
indexOf()
to guard subsequent logic that requires the presence of the substring.javascriptconst content = "Important data: extract me"; const startIndex = content.indexOf("data:"); if(startIndex !== -1) { const data = content.slice(startIndex + 5); console.log(data.trim()); } else { console.error("Data marker not found!"); }
This code looks for the
'data:'
marker. If found, it extracts and logs the information following this marker. If not found, it logs an error message, preventing further erroneous processing.
Conclusion
The indexOf()
function in JavaScript is a versatile tool for identifying the position of characters or substratches within strings. By returning a numeric index or -1
when not found, it provides a robust way to handle string search operations efficiently. Use this method in diverse situations, from validating input to processing text data, to ensure your JavaScript code remains precise and error-free. By mastering indexOf()
, you enhance your string manipulation capabilities in JavaScript programming.
No comments yet.