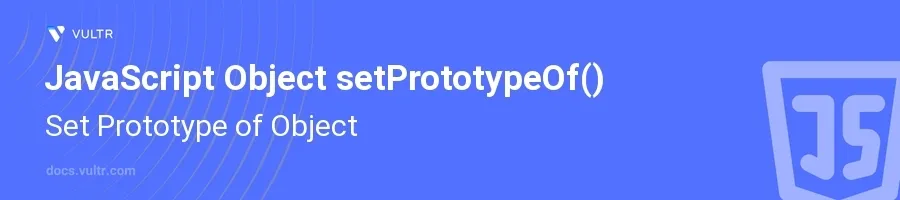
Introduction
The Object.setPrototypeOf()
method in JavaScript is a powerful tool for changing the prototype (i.e., the internal [[Prototype]]
slot) of a specified object to another object or null
. This method is part of the ECMAScript 2015 (ES6) specification and is used primarily for dynamic inheritance restructuring and in cases where the prototype needs to be switched after the object's creation.
In this article, you will learn how to effectively use the Object.setPrototypeOf()
method. Explore various practical examples to understand how to change an object’s prototype, consider performance implications, and learn best practices in applying this method in your JavaScript projects.
Understanding Object.setPrototypeOf()
Basic Usage of setPrototypeOf
Determine the object for which you want to change the prototype.
Specify the new prototype object.
javascriptlet animal = { isAlive: true }; let dog = { barks: true }; Object.setPrototypeOf(dog, animal); console.log(dog.isAlive); // Outputs: true
This example demonstrates setting
animal
as the prototype ofdog
. Now,dog
inherits properties fromanimal
.
Handling null
as Prototype
Set the prototype of an object to
null
to remove any prototype linkage.javascriptlet item = { name: 'Widget' }; Object.setPrototypeOf(item, null); console.log('toString' in item); // Outputs: false
Here, after setting the prototype to
null
,item
loses access to theObject.prototype
methods such astoString()
.
Performance Considerations
Object Creation with Direct Prototype Assignment
Recognize that altering an object's prototype after creation can lead to performance drawbacks.
javascriptlet animal = { isAlive: true }; let dog = Object.create(animal); dog.barks = true; console.log(dog.isAlive); // Outputs: true
Using
Object.create()
for setting the prototype at the time of object creation is generally more performant and recommended over usingObject.setPrototypeOf()
.
Impact on Engine Optimizations
- Understand that modern JavaScript engines optimize based on the assumption that prototypes are rarely changed.
- Accept that changing an object’s prototype dynamically using
Object.setPrototypeOf()
may cause deoptimizations.
Best Practices
- Prefer setting the prototype at the time of object creation using
Object.create()
or other mechanisms avoiding dynamic changes. - Limit the use of
Object.setPrototypeOf()
to cases where dynamic prototype changes are unavoidable or necessary for the functionality. - Test for performance impacts if using
Object.setPrototypeOf()
in performance-critical sections of code.
Conclusion
The Object.setPrototypeOf()
function in JavaScript offers the capability to dynamically change the prototype of objects, facilitating flexibility in object inheritance structures. However, due to potential performance penalties, use this method judiciously and prefer alternative approaches like Object.create()
where feasible. By understanding both the powers and limitations of Object.setPrototypeOf()
, you can make more informed decisions about its deployment in your JavaScript coding projects.
No comments yet.