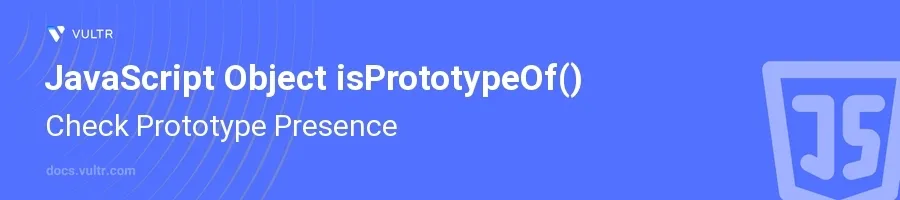
Introduction
The JavaScript method isPrototypeOf()
is crucial for understanding the prototype chain in object-oriented JavaScript. It allows you to verify whether a specific object exists within the prototype chain of another object, which is fundamental in the realm of JavaScript inheritance where prototype chains provide a mechanism for object to inherit features from others.
In this article, you will learn how to utilize the isPrototypeOf()
method effectively across different scenarios. Explore its role in confirming object relationships and in maintaining the integrity of prototype chains, which is a cornerstone for efficiently managing inheritance in JavaScript applications.
Understanding isPrototypeOf()
Basic Usage of isPrototypeOf()
Define a prototype object.
Create another object that inherits from the prototype.
Use
isPrototypeOf()
to check the relationship.javascriptconst vehicle = { start() { return "Starting the engine!"; } }; const car = Object.create(vehicle); console.log(vehicle.isPrototypeOf(car));
This example creates
car
as an object that inherits fromvehicle
. TheisPrototypeOf()
method confirms thatvehicle
is indeed a prototype ofcar
, returningtrue
.
Checking Deeper Inheritance
Expand on basic usage by creating a multi-level inheritance chain.
Use
isPrototypeOf()
to check for indirect prototype relationships.javascriptconst vehicle = { start() { return "Starting the engine!"; } }; const car = Object.create(vehicle); const myCar = Object.create(car); console.log(vehicle.isPrototypeOf(myCar));
In this scenario,
vehicle
is the prototype ofcar
, andcar
is the prototype ofmyCar
. UsingisPrototypeOf()
checks whethervehicle
is indirectly the prototype ofmyCar
, thus returningtrue
.
Common Mistakes to Avoid
Beware of reversed arguments.
Do not confuse
isPrototypeOf()
with theinstanceof
operator.javascriptconst vehicle = { start() { return "Starting the engine!"; } }; const car = Object.create(vehicle); // Incorrect usage console.log(car.isPrototypeOf(vehicle)); // false
In the incorrect example, the code mistakenly checks if
car
is a prototype ofvehicle
, which is not the case, hence it returnsfalse
.
Practical Use Cases
Validating Prototype Integrity
Ensure that modifications do not break expected prototype relationships.
Regularly check prototypes in development, especially after dynamic changes.
javascriptconst vehicle = { start() { return "Starting the engine!"; } }; const car = Object.create(vehicle); // Assume modification might happen car.start = function() { return "Car starts differently!"; }; console.log(vehicle.isPrototypeOf(car)); // still true
Despite
car
having a modified method,vehicle
remains its prototype. This is critical for maintaining behaviors expected from the original prototype chain.
Frameworks and Libraries
Ensure custom objects or components correctly integrate with framework expectations.
Verify object structures where prototype chains are important, like in React or Angular components.
javascriptconst component = { render() {} }; const customComponent = Object.create(component); console.log(component.isPrototypeOf(customComponent)); // true
Here, ensuring that
customComponent
correctly inherits fromcomponent
might be critical for integration with other parts of a web application framework, keeping inheritance and component behavior intact.
Conclusion
The isPrototypeOf()
function in JavaScript offers a robust way to trace and confirm the relationships in prototype chains, which is indispensable for managing inheritance and object behavior in complex applications. Whether you're working on maintaining legacy code, developing robust APIs, or integrating JavaScript objects with frameworks, understanding and applying isPrototypeOf()
helps in verifying and safeguarding the intended object behaviors across your projects. Implement this method to ensure that your objects interact and function as expected within their respective hierarchies.
No comments yet.