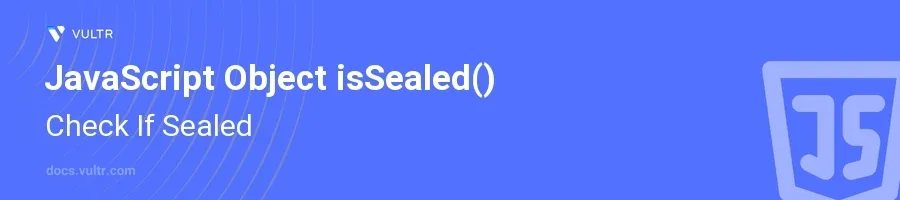
Introduction
The JavaScript Object.isSealed()
method is a direct way to check if an object is sealed. Sealing an object prevents new properties from being added to it and marks all existing properties as non-configurable. This means that properties cannot be deleted or reconfigured, though their values can still be modified if they are writable. Understanding how to check if an object is sealed is crucial for debugging and managing object configurations in JavaScript applications.
In this article, you will learn how to use the Object.isSealed()
method in various scenarios. Explore practical examples that illustrate checking if objects are sealed, understanding the impact on object properties, and how sealing differs from freezing objects.
Working with Object.isSealed()
Check If an Object is Sealed
Declare an object.
Seal the object using
Object.seal()
.Verify if the object is sealed by using
Object.isSealed()
.javascriptconst obj = { property1: 42 }; Object.seal(obj); const sealedStatus = Object.isSealed(obj); console.log(sealedStatus); // Output: true
This code demonstrates sealing an object and then checking if it is sealed. It outputs
true
, confirming that the object is indeed sealed.
Modifying Properties of a Sealed Object
Understand that while you cannot add new properties or reconfigure existing ones, you can still modify the values of writable properties in a sealed object.
Modify the property value of a sealed object.
Check the updated value.
javascriptobj.property1 = 100; console.log(obj.property1); // Output: 100
This code snippet changes the value of
property1
in the already sealed objectobj
. Despite the sealing, the modification of the value is valid and the new value is reflected.
Attempting to Add Properties to a Sealed Object
Try adding a new property to a sealed object.
Confirm that the addition does not take effect.
javascriptobj.newProperty = 'new'; console.log(obj.newProperty); // Output: undefined
In this example, when attempting to add a new property
newProperty
to the sealed objectobj
, the operation fails silently in non-strict mode, resulting inundefined
when trying to access the new property. This illustrates the restrictive nature of sealed objects regarding the addition of new properties.
Comparing Sealed and Frozen Objects
Exploring Differences
Recognize that while a sealed object cannot have new properties added and cannot have its existing properties reconfigured, its properties' values (if writable) can still be changed.
Understand that a frozen object is more restrictive: no new properties can be added, no existing properties can be changed, and no properties' values can be modified.
javascriptconst frozenObj = Object.freeze({ property1: 42 }); console.log(Object.isSealed(frozenObj)); // Output: true console.log(Object.isFrozen(frozenObj)); // Output: true
This example shows that a frozen object is also considered sealed. However, the reverse is not true: a sealed object is not necessarily frozen, demonstrated by the writable property values in a sealed object.
Conclusion
The Object.isSealed()
function in JavaScript is instrumental for verifying if an object is sealed. By mastering this check, you can manage object stability and configuration effectively in your coding projects. Use sealing to protect object structures from changes while maintaining flexibility in modifying writable property values, contrasting with freezing, which locks the object's state entirely. Apply the methods and examples discussed in this article to enhance security and predictability in your JavaScript applications.
No comments yet.