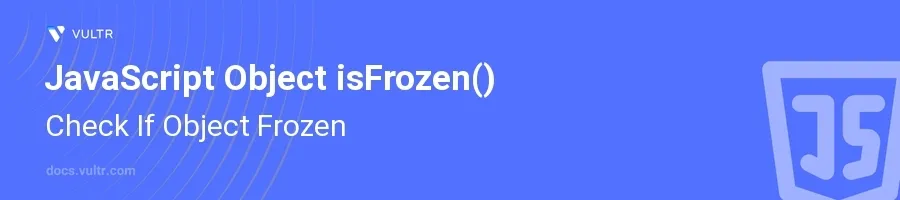
Introduction
The Object.isFrozen()
method in JavaScript is an invaluable tool for developers to verify the immutability of an object. It allows you to check if an object is frozen, meaning that its properties cannot be modified, properties cannot be added, and they cannot be removed — a state achieved using Object.freeze()
. Ensuring the immutability of an object can be critical in the development of secure and predictable JavaScript applications, especially in contexts where state management is key.
In this article, you will learn how to utilize the Object.isFrozen()
method in your JavaScript projects. This guide will demonstrate practical scenarios for checking an object's frozen status and discuss the importance of such operations in maintaining the integrity of your application states.
Checking the Frozen Status of an Object
Basic Usage of isFrozen()
Create an object in your JavaScript code.
Freeze the object using
Object.freeze()
.Check if the object is frozen with
Object.isFrozen()
.javascriptconst obj = { name: "Example" }; Object.freeze(obj); const isFrozen = Object.isFrozen(obj); console.log(isFrozen); // outputs: true
This code first creates an object with one property, freezes it, and then checks whether it is frozen. The console logs
true
because the object is indeed frozen after theObject.freeze()
method is applied.
Confirming Behavior on Unfrozen Objects
Verify how
isFrozen()
behaves when applied to objects that have not been frozen.javascriptconst obj = { name: "Not frozen" }; const isFrozen = Object.isFrozen(obj); console.log(isFrozen); // outputs: false
In this example, checking an unfrozen object returns
false
, verifying thatObject.isFrozen()
correctly identifies that the object has not been subjected toObject.freeze()
.
Practical Considerations
- Be mindful of the mutable or immutable condition of objects when working with methods like
Object.freeze()
. UseObject.isFrozen()
to systematically check an object's status, especially before running operations that rely on the object state not changing. - Remember that freezing an object ensures no new properties can be added, existing properties cannot be removed, and existing properties' values can't be changed, making it perfect for creating truly constant objects.
Conclusion
The Object.isFrozen()
method in JavaScript provides an effective way to check if an object is immune to future changes, which can be especially important when data integrity needs to be guaranteed. By incorporating the Object.isFrozen()
check in your JavaScript coding practices, you help ensure that objects maintain their intended state throughout the execution of your program. Whether you're confirming the immutability of configuration objects or setting up foolproof conditions for your application's core functionalities, understanding and using Object.isFrozen()
empowers you to build more reliable and robust applications.
No comments yet.