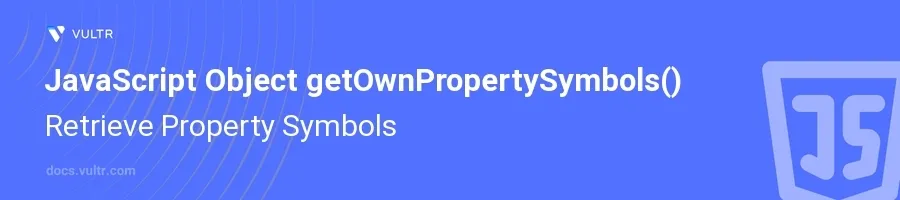
Introduction
In JavaScript, symbols are a primitive data type introduced in ECMAScript 2015 (ES6) that enable the creation of unique identifiers for object properties. Unlike strings, each symbol value is distinct, which helps avoid name clashes in complex applications and libraries. The getOwnPropertySymbols()
method is a static function on the Object
constructor that retrieves all symbol properties from a specified object.
In this article, you will learn how to use Object.getOwnPropertySymbols()
to access symbol-based property keys from objects. Dive into various examples to understand how this method can be part of your coding toolkit, especially when dealing with non-traditional property identifiers that enhance privacy and control over object attributes.
Retrieving Symbol Properties from Objects
Basic Usage of getOwnPropertySymbols()
Initialize an object with symbol properties.
Use
Object.getOwnPropertySymbols()
to retrieve the symbols.javascriptconst sym1 = Symbol('alpha'); const sym2 = Symbol('beta'); let obj = { [sym1]: 'Value of alpha', [sym2]: 'Value of beta' }; let symbols = Object.getOwnPropertySymbols(obj); console.log(symbols); // Outputs: [ Symbol(alpha), Symbol(beta) ]
This example defines two symbols and adds them as keys to an object
obj
.Object.getOwnPropertySymbols(obj)
returns an array of the symbol keys used in the object.
Accessing Symbol-based Property Values
Retrieve the symbols from the object using
Object.getOwnPropertySymbols()
.Iterate over the symbols to access the corresponding property values.
javascriptsymbols.forEach((symbol) => { console.log(`${String(symbol)}: ${obj[symbol]}`); });
Using the
forEach()
method, you can iterate over each symbol returned byObject.getOwnPropertySymbols()
and access the respective property values in the object by using the symbol as a key.
Combining Symbol and String Properties
Combine both string and symbol properties in a single object.
Use both
Object.getOwnPropertySymbols()
andObject.keys()
to list all properties.javascriptlet objMixed = { [sym1]: 'Symbol-based value', normalKey: 'String-based value' }; let symbolKeys = Object.getOwnPropertySymbols(objMixed); let stringKeys = Object.keys(objMixed); console.log('Symbol keys:', symbolKeys); console.log('String keys:', stringKeys);
This snippet shows how to handle objects containing both symbol and traditional string keys.
Object.keys()
retrieves normal (string-keyed) properties, whereasObject.getOwnPropertySymbols()
extracts those that are keyed with a symbol.
Conclusion
The getOwnPropertySymbols()
method is an essential tool for manipulating and accessing symbol-keyed properties in JavaScript objects. It allows developers to fully leverage symbols to manage property visibility and ensure uniqueness, avoiding potential overlaps in property names that are common in larger or modular applications. By mastering Object.getOwnPropertySymbols()
, you enhance your ability to work with modern JavaScript applications that utilize metadata and internally tracked properties effectively.
No comments yet.