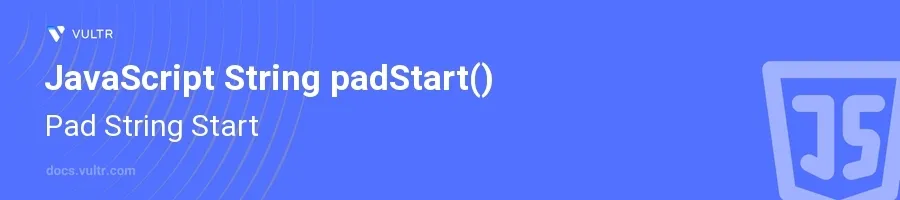
Introduction
In JavaScript, the padStart()
method is a handy tool for manipulating strings, specifically for adding padding to the start of a string until it reaches a certain length. This method is particularly useful in formatting tasks, such as ensuring that all numbers display with the same width in a UI, or in preparing data for tabular display where alignment is key.
In this article, you will learn how to use the padStart()
method to add padding to strings for various purposes. Explore how to specify both the length of the resulting string and the string used for padding, and see how these capabilities can be applied in real-world scenarios to enhance data readability and presentation.
Using padStart() in Common Scenarios
Ensuring Fixed Width for Numbers
Consider when numerical data needs to appear in a fixed width, such as in a financial report.
Convert the number to a string and use
padStart()
to pad it.javascriptconst number = 42; const paddedNumber = number.toString().padStart(5, '0'); console.log(paddedNumber);
This converts the number
42
to a string and pads it with zeros on the left, resulting in the string"00042"
.
Formatting Date and Time Components
In applications where date and time need uniform formatting, like in log files.
Use
padStart()
to format hours, minutes, or seconds.javascriptconst hours = '9'; const formattedHours = hours.padStart(2, '0'); console.log(formattedHours);
This pads the hour
9
to"09"
, ensuring all hour values are uniformly formatted with two digits.
Aligning Text for Display
When displaying text in a UI where alignment enhances readability, such as in a menu or a table.
Apply
padStart()
to align text entries uniformly by their left edges.javascriptconst text = 'apple'; const maxLength = 10; const paddedText = text.padStart(maxLength); console.log(paddedText);
This code pads the word
"apple"
with spaces at the start, making it right-aligned in a field of 10 characters.
Conclusion
The padStart()
method in JavaScript provides an efficient and simple way to manipulate string length by adding padding to the start of strings. Its primary use cases include aligning text, standardizing data presentation, and ensuring uniformity in visual displays such as tables and reports. By incorporating padStart()
into string handling routines, developers can enhance the clarity and aesthetics of data presentation, benefiting user interfaces and data processing tasks alike. Utilize this method to maintain consistent formatting in your applications, ensuring a professional appearance and easy readability.
No comments yet.