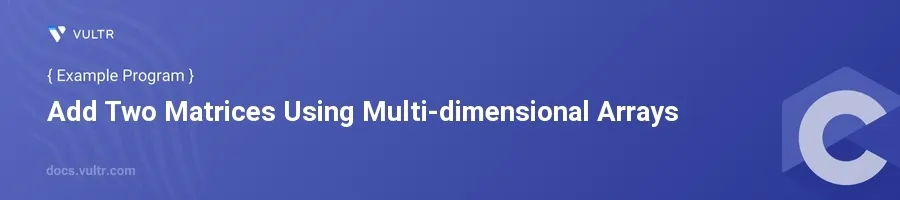
Introduction
Adding two matrices in programming is a fundamental operation that can be achieved through the use of multi-dimensional arrays in C. This operation involves element-wise addition of two matrices of the same size, which results in a new matrix where each element is the sum of the elements from the two matrices at the same position.
In this article, you will learn how to create a C program that adds two matrices using multi-dimensional arrays. Explore how multi-dimensional arrays are used to represent matrices and follow through practical examples that demonstrate the process of matrix addition.
Matrix Addition in C
Initializing the Matrices
Define the dimensions of the matrices which must be the same for both matrices.
Initialize two matrices as multi-dimensional arrays, and a third matrix to store the result of the addition.
c#include <stdio.h> int main() { int matrix1[2][2] = {{1, 2}, {3, 4}}; int matrix2[2][2] = {{5, 6}, {7, 8}}; int resultMatrix[2][2]; int i, j; }
Here,
matrix1
andmatrix2
are initialized with predefined values, andresultMatrix
is used to store the result.
Adding the Matrices
Loop through each element of the matrices using nested loops, one for rows and another for columns.
Add the corresponding elements of
matrix1
andmatrix2
, and store the result inresultMatrix
.cfor (i = 0; i < 2; i++) { for (j = 0; j < 2; j++) { resultMatrix[i][j] = matrix1[i][j] + matrix2[i][j]; } }
This nested loop structure allows accessing each element of the matrices. The elements at the same indices from
matrix1
andmatrix2
are added and stored in the corresponding index ofresultMatrix
.
Displaying the Result
Use another nested loop to print out the contents of the result matrix.
cprintf("Resultant Matrix:\n"); for (i = 0; i < 2; i++) { for (j = 0; j < 2; j++) { printf("%d ", resultMatrix[i][j]); } printf("\n"); } return 0;
This loop iterates through
resultMatrix
and prints each element. Theprintf("\n");
ensures that each row of the matrix is printed on a new line.
Conclusion
Creating a C program to add two matrices using multi-dimensional arrays involves initializing the matrices, performing element-wise addition, and displaying the result. Multi-dimensional arrays serve as an efficient way to handle operations on matrices in C. By understanding and implementing these steps, you leverage multi-dimensional arrays effectively, offering a clear and structured approach to matrix operations. Use this knowledge to extend to more complex matrix operations and other applications in scientific computing, data analysis, and more.
No comments yet.