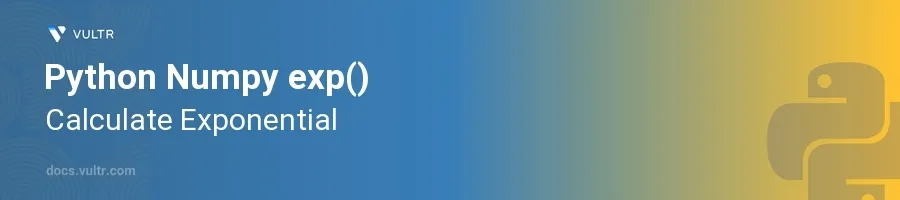
Introduction
The exp()
function from the NumPy library in Python is essential for performing exponential calculations, which is the process of raising a number to the power of e (Euler's number, approximately 2.71828). This function is particularly useful in fields like data science, economics, and engineering, where exponential growth models or natural logarithm calculations are common.
In this article, you will learn how to efficiently utilize the exp()
function to perform exponential calculations on arrays and individual numbers. Explore the application of this function in practical programming scenarios and learn how to handle different data types and structures.
Using exp() with Single Numbers
Calculate the Exponential of a Number
Import the NumPy library.
Apply the
exp()
function to a single number.pythonimport numpy as np number = 2 result = np.exp(number) print(result)
This code computes e raised to the power of 2 (e²). The result is approximately 7.389056, showcasing how
exp()
calculates exponential values.
Explore Precision with Floating Point Numbers
Recognize that Python's floating point numbers have limitations in precision.
Perform exponential calculations on a floating point number.
pythonfloat_number = 1.5 result = np.exp(float_number) print(result)
This snippet calculates e raised to the power of 1.5, yielding about 4.48169, demonstrating the precision capabilities of the
exp()
function for floating point numbers.
Using exp() with Arrays
Calculate Exponential Values for Each Element in an Array
Define a NumPy array with several numbers.
Use the
exp()
function to compute the exponential for each element.pythonarr = np.array([1, 2, 3]) results = np.exp(arr) print(results)
This code demonstrates calculating the exponential of each element in an array. The resulting array,
[2.71828183, 7.3890561 , 20.08553692]
, illustrates thatexp()
efficiently applies the operation across the array.
Handling Large Arrays
Understand that operations on large arrays may consume significant computing resources.
Apply the
exp()
function to a large array and consider the implications.pythonlarge_arr = np.random.rand(1000) * 10 # creating a large array with random numbers from 0 to 10 large_results = np.exp(large_arr) print(large_results)
Applying
exp()
on a large array showcases its capability to efficiently handle bulk calculations, which is useful in data analysis and scientific computing.
Conclusion
The exp()
function in NumPy is a powerful tool for calculating exponential values in Python. Its application ranges from simple number calculations to comprehensive arrays, supporting various technical and scientific computing needs. By implementing the techniques discussed, you ensure your computational routines are efficient and effective, whether tackling small-scale or large-scale data operations. Remember to utilize this function to streamline and optimize your exponential calculations within your Python projects.
No comments yet.