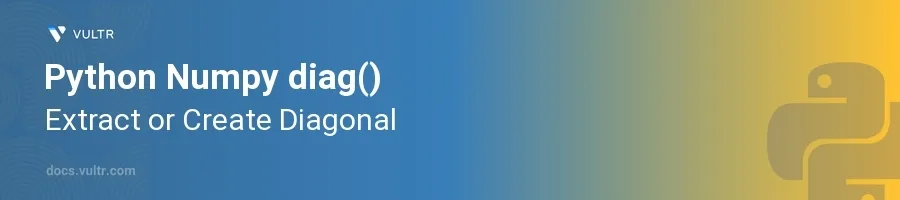
Introduction
The numpy.diag()
function in Python plays a crucial role in matrix operations, either extracting the diagonal elements from a given array or creating a diagonal array from a given input sequence. This function is an essential tool for scientific and mathematical computing, often used in linear algebra and data processing tasks.
In this article, you will learn how to harness the power of the numpy.diag()
function to both extract diagonals from existing arrays and construct diagonal arrays efficiently. Explore practical examples to understand different usage scenarios, and enhance your capability in handling array manipulations with NumPy.
Extracting Diagonal Elements
Extract the Main Diagonal from a Matrix
Import the NumPy library.
Create a square or rectangular matrix.
Utilize the
numpy.diag()
to retrieve the main diagonal.pythonimport numpy as np matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) diagonal = np.diag(matrix) print(diagonal)
This example extracts the main diagonal
[1, 5, 9]
from the matrix. Thenumpy.diag()
function retrieves elements where the indices are equal (i.e.,[0,0]
,[1,1]
,[2,2]
).
Extract Off-center Diagonals
Specify the offset for the diagonal relative to the main diagonal.
Apply the
numpy.diag()
function with thek
parameter to extract the desired diagonal.pythonupper_diagonal = np.diag(matrix, k=1) lower_diagonal = np.diag(matrix, k=-1) print("Upper diagonal: ", upper_diagonal) print("Lower diagonal: ", lower_diagonal)
In this case,
upper_diagonal
will output[2, 6]
andlower_diagonal
will output[4, 8]
, showing elements above and below the main diagonal, respectively.
Creating Diagonal Arrays
Create a Matrix with Diagonal Entries
Start with a 1D array that represents the diagonal.
Use
numpy.diag()
with this array to construct a 2D diagonal matrix.pythondiagonal_elements = np.array([1, 2, 3]) diagonal_matrix = np.diag(diagonal_elements) print(diagonal_matrix)
The output matrix will have the input array as its main diagonal, while all off-diagonal elements are zeroes, forming a square matrix.
Generate a Matrix with an Off-center Diagonal
Define the diagonal elements and the offset using the
k
parameter.Construct a diagonal matrix with these specifications.
pythonoff_center_diagonal = np.diag(diagonal_elements, k=-1) print(off_center_diagonal)
This produces a matrix with the diagonal elements shifted one row downwards, as specified by
k=-1
.
Conclusion
Utilizing the numpy.diag()
function effectively allows for sophisticated manipulations related to diagonal elements in matrices, making it a valuable tool in a variety of scientific computing and data analysis applications. With the ability to easily extract or create diagonal matrices, optimize various tasks involving matrix operations. Implement these techniques in your projects to maximize data handling efficiency and improve computation strategies.
No comments yet.