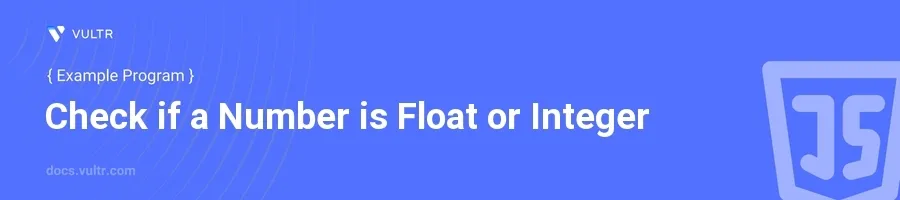
Introduction
Determining whether a number is a floating point or an integer is a common task in JavaScript, especially when working with numerical data input and validation. This kind of check helps in ensuring that data conforms to expected formats before processing it further in applications like financial calculators, data analysis tools, or when interacting with APIs that require specific numerical types.
In this article, you will learn how to differentiate between floating point numbers and integers in JavaScript through practical examples. You'll explore different methods to achieve this, enhancing your ability to handle and validate numerical data efficiently in your JavaScript programs.
Checking Number Type in JavaScript
Convert and Compare Method
Use the
Number()
function to ensure the value is treated as a numerical data type.Compare the original number with its integer-part obtained using
Math.floor()
.javascriptfunction isInteger(num) { return num === Math.floor(num); } console.log(isInteger(10)); // true console.log(isInteger(10.5)); // false
This function checks if a number is an integer by comparing it to its floored version. If they are the same, the number is an integer; otherwise, it is a float.
Using the Modulus Operator
Apply the modulus operator to determine if there is any remainder when divided by 1.
A remainder of 0 indicates the number is an integer.
javascriptfunction isInteger(num) { return num % 1 === 0; } console.log(isInteger(5)); // true console.log(isInteger(5.5)); // false
Here, the modulus operation finds out if there is a fractional part in the number.
Utilizing ECMAScript 6 Features
Use the
Number.isInteger()
method introduced in ECMAScript 6 for a straightforward approach.javascriptconsole.log(Number.isInteger(23)); // true console.log(Number.isInteger(4.6)); // false
Number.isInteger()
directly checks if the provided value is an integer, offering an efficient and ready-to-use solution without additional coding.
Conclusion
Determining the type of a numerical value as float or integer in JavaScript can be achieved through various methods, each suited for different situations. Whether using ES6 features for straightforward checks or applying traditional JavaScript techniques like modulus operations or manual comparisons, these methods equip you with the flexibility to handle numbers effectively in your applications. Integrate these techniques into your validation processes to ensure robust data handling capabilities in your JavaScript projects.
No comments yet.