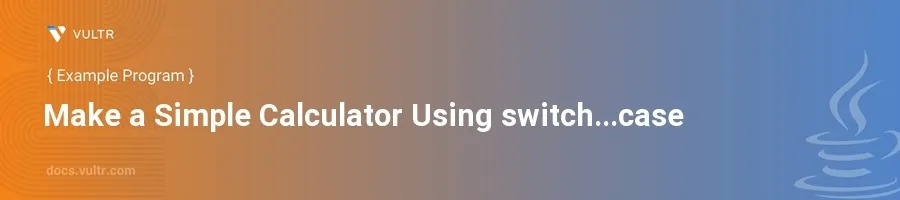
Introduction
A simple calculator is a great beginner project for anyone learning Java, as it encompasses fundamental programming concepts such as conditional statements, user inputs, and arithmetic operations. The use of switch...case
in a Java calculator program makes it especially educational because it introduces control flow mechanisms that are essential in many Java applications.
In this article, you will learn how to create a basic calculator program in Java using switch...case
. The examples provided will guide you through handling different arithmetic operations: addition, subtraction, multiplication, and division. This walkthrough not only bolsters your understanding of Java syntax but also demonstrates practical application of the concepts.
Building a Simple Calculator
Setup the Basic Structure
Start by setting up your Java environment.
Create a new Java file named
SimpleCalculator.java
.Import the necessary packages and declare the main class and method.
javaimport java.util.Scanner; public class SimpleCalculator { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Enter first number: "); double firstNumber = scanner.nextDouble(); System.out.println("Enter second number: "); double secondNumber = scanner.nextDouble(); System.out.println("Choose an operation (+, -, *, /): "); char operation = scanner.next().charAt(0); double result; switch (operation) { case '+': result = firstNumber + secondNumber; break; case '-': result = firstNumber - secondNumber; break; case '*': result = firstNumber * secondNumber; break; case '/': result = secondNumber != 0 ? firstNumber / secondNumber : Double.NaN; break; default: System.out.println("Invalid operation"); return; } System.out.printf("Result: %.2f%n", result); scanner.close(); } }
This code snippet sets up a simple calculator. It prompts the user to input two numbers and choose an arithmetic operation. The
switch
statement then determines which operation to perform based on the user's input.
Understanding the switch...case
Structure
Examine the
switch
block in the code.Note how each case corresponds to an arithmetic operation.
Observe the use of
break
to exit the switch once a match is found, and how division checks for division by zero.The use of
switch...case
in this context efficiently handles multiple potential operations. Each case in the structure is clearly devoted to a particular arithmetic operation, which makes the program easy to extend and maintain.
Running Your Calculator
- Compile your Java file from your command line or IDE:
console
javac SimpleCalculator.java
- Run the compiled Java program:
console
java SimpleCalculator
- Follow the prompts in your terminal to input numbers and an operation. Observe the result.
Conclusion
Implementing a simple calculator in Java using switch...case
provides a robust introduction to basic Java programming concepts. You've seen how to handle user input, perform arithmetic operations, and use conditional logic through switch...case
. The simplicity of switch...case
makes your code organized and easily understandable, which is particularly beneficial for debugging and enhancing the calculator's functionality. Utilize this structure in other areas of your Java development to manage multiple execution paths cleanly and efficiently.
No comments yet.