How to Manage Node Applications with PM2
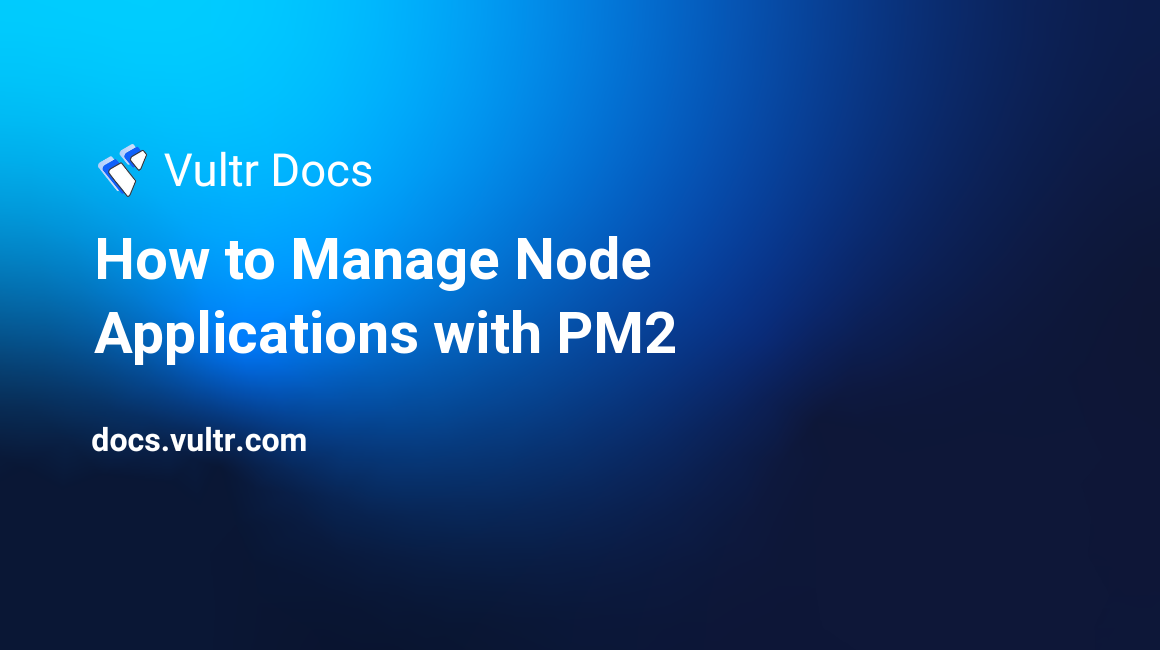
Introduction
Node.js is a server-side JavaScript environment that can be used to create high-performance, real-time applications for the web and mobile devices. Managing Node applications can require additional considerations beyond those needed when running standard web servers like Apache or Nginx.
PM2 is a process management system for Node applications designed for production usage. This article explains how to install and use PM2 to manage applications in production, along with the best practices.
Prerequisites
- You have a system (macOS, Linux, or Windows) with Node and NPM already installed.
- Log in to your server as the root user.
1. Install PM2 Using NPM
Install PM2 via the NPM package manager.
# npm install pm2 -g
2. Create an Example Node.js Application (optional)
If you already have a Node.js application that you want to run, you can skip this step.
Edit a new file named app.js
in your favorite text editor.
Paste the following contents into the file.
const http = require('http');
const hostname = '0.0.0.0';
const port = 80;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World');
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
Exit and save the file.
The app.js
file is an example Node.js web application. It listens on the TCP port 80. When it receives an HTTP request, it sends back a Hello World response. Once the web server bounds to listen on the port, it logs a message into the console.
3. Start the Application Using PM2
Start a Node.js application with PM2. Replace app.js
in the next command with your own application's initial file if you want to start an existing Node.js application rather than the example.
# pm2 start app.js
You may use several additional parameters when starting an application:
--name <app_name>
- specify an application name--watch
- restart your application automatically when a file changes--max-memory--restart <100MB>
- a memory limit that triggers an automatic restart when reached--log <log_file>
- specify a log file-- arg1 arg2
- pass extra arguments to the script--restart-delay <2000ms>
- delay between automatic restarts in milliseconds--time
- prefix logs with time--no-autorestart
- turn off automatic application restarts--cron
- a cron job pattern for automatic application restarts--no-daemon
- do not run the application in a daemon process
Visit the official documentation to view all available parameters.
4. Manage the Application
You may manage a PM2 application with the restart, reload, stop and delete operations.
Restart an application.
# pm2 restart app
Reload an application.
# pm2 reload app
Stop an application.
# pm2 stop app
Delete an application.
# pm2 delete app
5. List All Applications
Retrieve a list of all applications.
# pm2 list
6. Display Logs
View application logs in real-time.
# pm2 logs
View older logs. Specify the line amount with the --lines
parameter.
# pm2 logs --lines 200
7. Monitor the Resource Usage
You can monitor memory and CPU usage with the next command.
# pm2 monit
8. Set up a Web-Based Dashboard
Configure a web-based PM2 dashboard.
# pm2 plus
The command asks you whether you have a PM2 account. If you have one, answer yes and provide the login credentials. Otherwise, answer no and fill in the required information to create one. Accept the license agreement.
After the setup completes, the tool prints a web address to the dashboard. Open a web browser and navigate to the address.
Log in with your created PM2 account and use the dashboard.
9. Configure PM2 to Start on Server Boot
Generate a startup script and configure PM2 to start on server boot.
# pm2 startup
If you want to stop PM2 from starting on server boot, use the next command.
# pm2 unstartup systemd
10. Configure Applications to Start on Server Boot
You may configure PM2 applications to start automatically on server boot by saving them into an application list.
Save the running applications to the list.
# pm2 save
If you need to restore the saved applications manually from the list, use the next command.
# pm2 resurrect
11. Check for Updates
Print a list of outdated NPM packages on your system.
# npm outdated -g
If the list contains the PM2 package, a newer version is available.
12. Update PM2
Update the PM2 package via NPM.
The update stops all your running PM2 applications and starts them again after the update. Your applications will be unavailable until the update process is complete.
# npm install pm2@latest -g
Perform an in-memory update of PM2.
# pm2 update
Best Practices and Security Considerations
Prevent Downtime with Cluster Mode
Your applications will restart many times during their lifespan. A restart may cause a downtime period until the application is started again.
You may use the cluster mode feature in PM2 to avoid downtime.
Cluster mode runs multiple instances of your application. When a restart is required, only one instance at a time is stopped and restarted, while the other instances continue to serve your application.
Stop the application before switching to cluster mode.
# pm2 stop app
Start the application in cluster mode. Specify the number of instances with the `-i' parameter.
# pm2 start app.js -i 3
Scale an application. Specify the new amount of instances as the last parameter.
# pm2 scale app 4
Eliminate Failed Requests with Graceful Shutdown
Graceful shutdowns allow your application to finish all tasks before it exits. For example, your application may need to complete HTTP requests that are being handled or close the database connection before it exits.
A graceful shutdown in PM2 is executed as follows:
- Before the shutdown, PM2 sends an
SIGINT
signal to your application - The signal is intercepted by your application
- Your application finishes all requests and closes all database connections
- Your application exits
You may add the following code to enable graceful shutdown in the example application you've created, for instance. The code intercepts the SIGINT
signal, logs a message to the console, closes the HTTP server, and exits the process.
process.on('SIGINT', function() {
// sigint signal received, log a message to the console
console.log('Shutdown signal intercepted');
// close the http server
server.close();
// exit the process
process.exit();
});
Conclusion
In this article, you have learned how to set up PM2 and use it to manage Node.js applications.
You have also learned to configure advanced features such as cluster mode or graceful shutdowns to eliminate downtime from your application.
More Information
To learn more about PM2, visit the official documentation.