How to Setup a Koa.js Node Application on Ubuntu 20.04 with Nginx
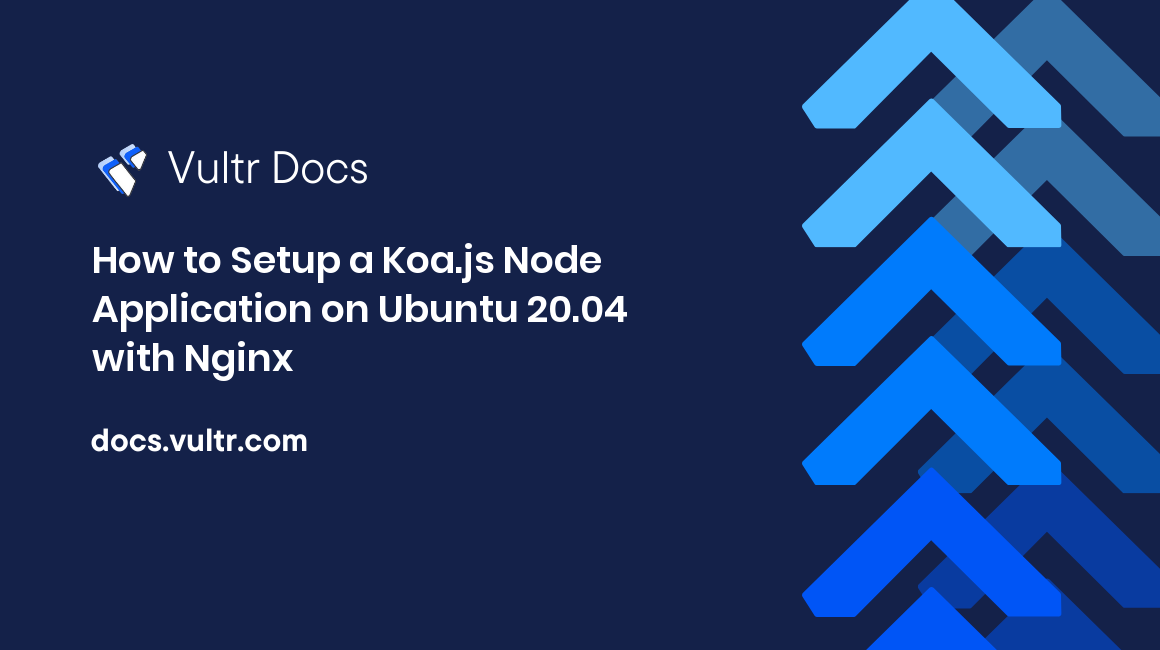
Overview
Koa.js is a modern web framework built on Node.js. It allows developers to build high-performace and scalable web applications by focusing on a concise and extendible API built on asynchronous functionality in Node.js.
Prerequisites
- Deploy a new Ubuntu 20.04 Vultr cloud server.
- Set up a non-root user with sudo privileges.
- Verify your server is up to date.
Install Nodejs & Yarn
Koa requires Node.js version v7.6.0 or higher.
Install the latest LTS version of Nodejs.
$ curl -fsSL https://deb.nodesource.com/setup_lts.x | sudo -E bash - $ sudo apt-get install nodejs
Verify successful Nodejs installation.
$ node -v v16.xx.x
Install Yarn.
$ curl -fsSL https://dl.yarnpkg.com/debian/pubkey.gpg | sudo apt-key add - $ echo "deb https://dl.yarnpkg.com/debian/ stable main" | sudo tee /etc/apt/sources.list.d/yarn.list $ sudo apt-get update && sudo apt-get install yarn
Verify successful Yarn installation.
$ yarn -v 1.2x.xx
Setup Application
Create the project root directory.
$ cd ~ $ mkdir koaserver && cd koaserver
Initialize the Node.js project environment through
yarn
and answer the question prompts according to your project.$ yarn init
Install Koa.
$ yarn add koa
Create
index.js
.$ touch index.js
Add sample code to
index.js
.const Koa = require("koa"); const app = new Koa(); app.use(async (ctx) => { ctx.body = "Hello World"; }) app.listen(3000);
Setup PM2
PM2 will manage the Node.js application to run in the background as a system process. PM2 will automatically restart the server if it crashes, handle logs, and monitor performance.
Install PM2.
$ sudo yarn global add pm2
Confirm successful installation.
$ pm2 -V 5.x.x
Navigate to the project folder and start the Koa server through PM2.
$ cd ~/koaserver $ pm2 start index.js --name koaserver
Set PM2 to run on startup.
$ eval "$(pm2 startup | tail -1)"
Save PM2 configuration.
$ pm2 save
Setup Reverse Proxy through Nginx
Install Nginx.
$ sudo apt-get install nginx
Delete default Nginx server block.
$ sudo rm /etc/nginx/sites-enabled/default
Create a new Nginx server block.
$ sudo touch /etc/nginx/sites-enabled/koaserver.conf
Add the following text to the file.
server { listen 80; listen [::]:80; server_name _; location / { proxy_pass http://127.0.0.1:3000; } }
Restart Nginx.
$ sudo systemctl nginx restart
Verify a working server response by navigating to your server's IP address in a browser. You should see a
Hello World
message.
Setup Firewall
For added security, configure a firewall to restrict access to internal service ports.
Setup UFW rules.
$ sudo ufw allow 22,80,443/tcp
Add any other ports according to your application and needs.
Enable UFW.
$ sudo ufw enable
Verify UFW working.
$ sudo ufw status Status: active
Conclusion
For more information, refer to the official Koa.js documentation.