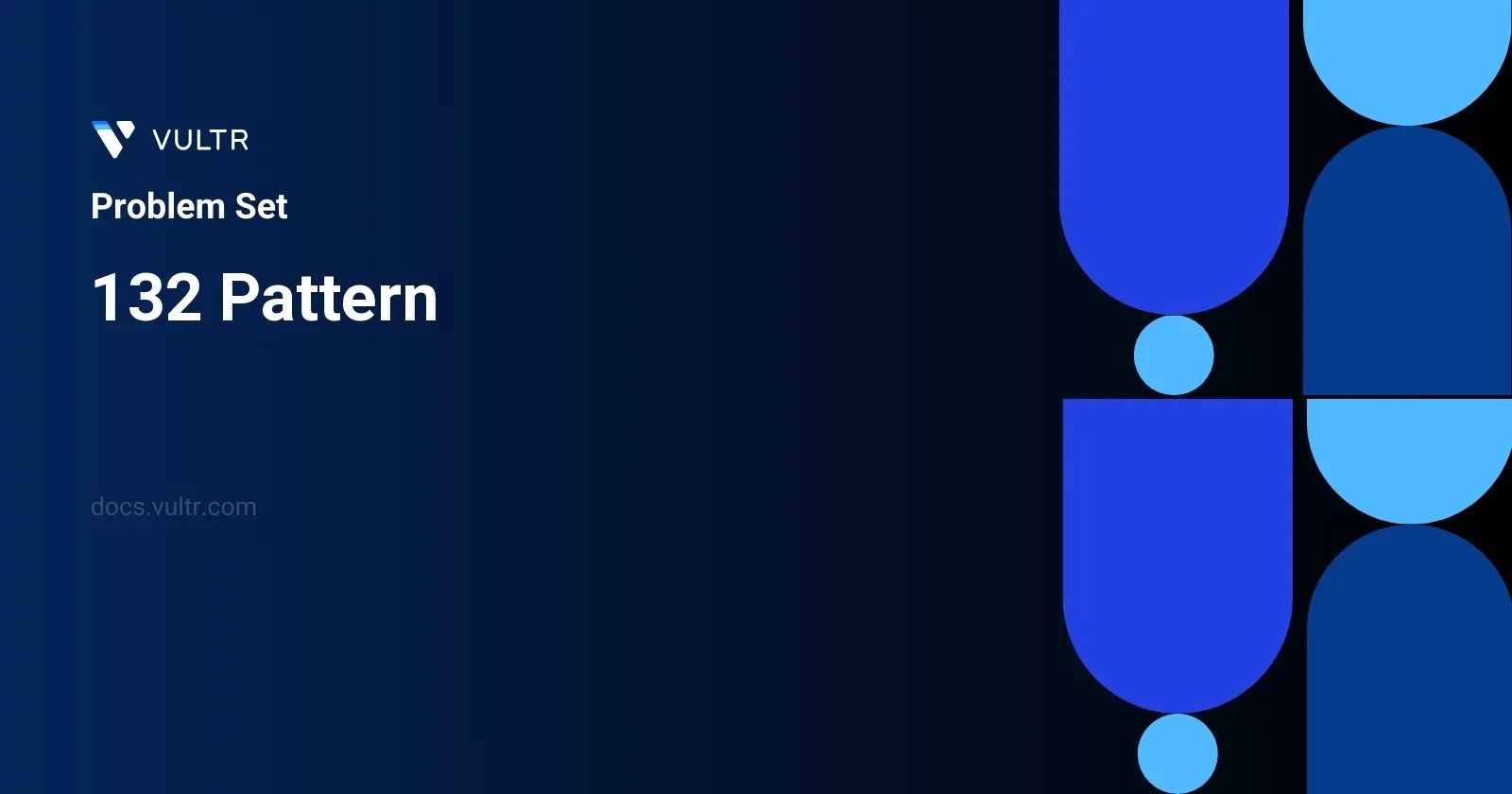
Problem Statement
In this problem, you are given an array of integers named nums
. Your task is to determine if there exists at least one subsequence that follows the 132 pattern. A subsequence in this context is defined by three integers from the given array: nums[i], nums[j], nums[k]
, where the indices meet the condition i < j < k
. The values at these indices must satisfy the pattern nums[i] < nums[k] < nums[j]
. If such a subsequence exists, your function should return true
; otherwise, it returns false
.
Examples
Example 1
Input:
nums = [1,2,3,4]
Output:
false
Explanation:
There is no 132 pattern in the sequence.
Example 2
Input:
nums = [3,1,4,2]
Output:
true
Explanation:
There is a 132 pattern in the sequence: [1, 4, 2].
Example 3
Input:
nums = [-1,3,2,0]
Output:
true
Explanation:
There are three 132 patterns in the sequence: [-1, 3, 2], [-1, 3, 0] and [-1, 2, 0].
Constraints
n == nums.length
1 <= n <= 2 * 105
-109 <= nums[i] <= 109
Approach and Intuition
Understanding the 132 pattern requires visualizing or tracing through potential subsequences within the array, adhering to the index and value conditions. Our objective is to identify three integers where the first one is less than the third, and the third is less than the second, forming a peak-like structure. To clarify this approach, consider the examples provided:
Breakdown of Examples
Example 1
- Input:
nums = [1,2,3,4]
- Output:
false
- Explanation: Sequentially increasing or decreasing numbers cannot form a valid 132 pattern since no middle value can be both greater and lesser between two other values.
Example 2
- Input:
nums = [3,1,4,2]
- Output:
true
- Explanation: The subsequence
[1, 4, 2]
adheres to the 132 pattern because1 < 2 < 4
. Here1
isnums[i]
,4
isnums[j]
, and2
isnums[k]
fulfillingi < j < k
.
Example 3
- Input:
nums = [-1,3,2,0]
- Output:
true
- Explanation: Several subsequences here meet the 132 criteria:
[-1, 3, 2]
fits because-1 < 2 < 3
[-1, 3, 0]
fits because-1 < 0 < 3
[-1, 2, 0]
also fits since-1 < 0 < 2
Intuition behind Solution
To solve this problem efficiently:
- Use a structure to maintain a record of possible values for
nums[k]
. You can use a stack, which keeps track of potential candidates fornums[k]
as you iterate the array from right to left. - Continuously update the minimum possible value for
nums[i]
as you go through array elements. - For each element, check against the potential
nums[k]
values in the stack — ensuring that the current element can serve asnums[j]
, checking if it can form a valid pattern with some values behind it consideringnums[i]
andnums[k]
.
This combination of scanning and stack-based approach leverages past computations efficiently, attempting to optimize the nested iteration scenario, particularly under constraints where n
can be very large.
This approach effectively manages complexity, navigating through numerous possible index combinations efficiently, which otherwise could escalate into a time-intensive process given large array inputs as stated in the constraints.
Solutions
- C++
- Java
- Python
class Solution {
public:
bool check132pattern(vector<int>& elements) {
int n = elements.size();
if (n < 3) {
return false;
}
vector<int> leftMin(n);
leftMin[0] = elements[0];
for (int i = 1; i < n; i++) {
leftMin[i] = min(leftMin[i - 1], elements[i]);
}
for (int j = n - 1, k = n; j > 0; j--) {
if (elements[j] <= leftMin[j]) continue;
while (k < n && elements[k] <= leftMin[j]) k++;
if (k < n && elements[k] < elements[j]) {
return true;
}
elements[--k] = elements[j];
}
return false;
}
};
The given C++ code defines a method check132pattern
within a class named Solution
. This method aims to determine if there exists a subsequence of at least three numbers in the given array elements
that can form a pattern of 132. Here's how the solution works:
- The method starts by determining the size of the input vector
elements
. If the size is less than three, it immediately returnsfalse
as no triplet can exist. - An auxiliary vector
leftMin
is created to keep track of the minimum values from the left up to the current position. The first element is initialized to the first element ofelements
. - A loop fills the
leftMin
vector where each position holds the minimum value found so far from the start of the array to that position. - Another loop starts from the end of the array and uses variables
j
andk
to check for the existence of the 132 pattern. The variablej
traverses the array from the last but one element to the start, andk
is used as a reference to scan for a suitable third element of the pattern.- The loop checks if the current element
elements[j]
is greater than the smallest element to its leftleftMin[j]
. If not, it skips the current iteration. - A nested while loop increments
k
to find an element larger than theleftMin[j]
but less thanelements[j]
. - If an element fulfilling the 132 condition is found (
elements[k] < elements[j]
), the function returnstrue
.
- The loop checks if the current element
- If the loops complete without finding a suitable triplet, the method returns
false
.
Thus, the function efficiently checks for the 132 pattern in the array by minimizing redundant checks and optimizing element comparisons with the help of the leftMin
vector and careful placement of the iterators j
and k
.
public class Solution {
public boolean checkPattern(int[] arr) {
if (arr.length < 3) return false;
int[] minValues = new int[arr.length];
minValues[0] = arr[0];
for (int i = 1; i < arr.length; i++) {
minValues[i] = Math.min(minValues[i - 1], arr[i]);
}
for (int j = arr.length - 1, k = arr.length; j >= 0; j--) {
if (arr[j] > minValues[j]) {
while (k < arr.length && arr[k] <= minValues[j]) k++;
if (k < arr.length && arr[k] < arr[j]) return true;
arr[--k] = arr[j];
}
}
return false;
}
}
In this Java solution, you will check for the presence of a 132 pattern in a given array of integers. The 132 pattern is identified where an element n1
is followed by n2
, which is followed by n3
, and n1 < n3 < n2
. This can be approached systematically:
- First, verify if the array contains at least three elements to form a valid pattern. If not, immediately return
false
. - Create an array
minValues
to store the minimum value found up to each index of the original array. Initialize the first element ofminValues
with the first element of the input array. - Populate the
minValues
array by maintaining the minimum value seen so far as you iterate through the array. - Then, traverse the array from the last element towards the first. Use two pointers:
j
for the current position being checked in the original array andk
initialized beyond the end of the array. - For each element, compare the current element with the minimum value at that index. If the current element is greater:
- Check if there exists a suitable
n3
(middle element in the 132 pattern). Movek
such that it points to the potentialn3
while ensuringn3
remains greater than the minimum value atj
. - If a valid
n3
is found that is also less than the current elementarr[j]
, immediately returntrue
. - If not found, update the index
k
to the current indexj
, effectively moving backwards to check for other potentialn3
values.
- Check if there exists a suitable
- If no valid pattern is detected through the entire array, conclude by returning
false
.
This algorithm smartly checks the conditions for the 132 pattern using a backward iteration combined with a dynamic update of minimum values and a controlled search for the middle pattern element, leveraging both space and time efficiencies inherent in the process. Ensure to understand the conditions correctly and implement careful index management when adapting or expanding this solution.
class Solution:
def findPattern(self, values: List[int]) -> bool:
if len(values) < 3:
return False
lowest = [-1] * len(values)
lowest[0] = values[0]
for index in range(1, len(values)):
lowest[index] = min(lowest[index - 1], values[index])
right = len(values)
for middle in range(len(values) - 1, -1, -1):
if values[middle] <= lowest[middle]:
continue
while right < len(values) and values[right] <= lowest[middle]:
right += 1
if right < len(values) and values[right] < values[middle]:
return True
right -= 1
values[right] = values[middle]
return False
The provided Python solution efficiently determines if there exists a 132 pattern in a given list of integers. This pattern exists if there is a subsequence of three integers i, j, and k such that i < k < j. The implementation encapsulates the logic within a class named Solution
using the method findPattern
.
Here's how the algorithm operates:
- First, check if the input list contains fewer than three elements. If true, immediately return
False
as it's impossible to find a valid subsequence with less than three numbers. - Initialize an auxiliary list
lowest[]
that stores the minimum value encountered up to each index of the array. - Iterate through the values to populate the
lowest
array while comparing each element and maintaining the lowest value found up to the current position. - Use a stack-like approach with a pointer
right
set to the end of the list and iterate backward through the list, which allows efficient checking against the conditions of the 132 pattern. - For each "middle" element in the reversed loop:
- Skip over elements that do not satisfy the 132 middle value condition (if they are equal or less than their corresponding
lowest
value). - While maintaining the desired order, adjust the
right
pointer and update values to find a suitable "k". - If conditions for i, j, and k are met, return
True
.
- Skip over elements that do not satisfy the 132 middle value condition (if they are equal or less than their corresponding
- If no patterns are found across all iterations, return
False
.
The dual scan approach—forward to create the lowest
array and backward to check for pattern conditions—ensures that the method is efficient. Handling elements in this way exploits the nested nature of the pattern, streamlining detection by minimizing unnecessary comparisons.
No comments yet.