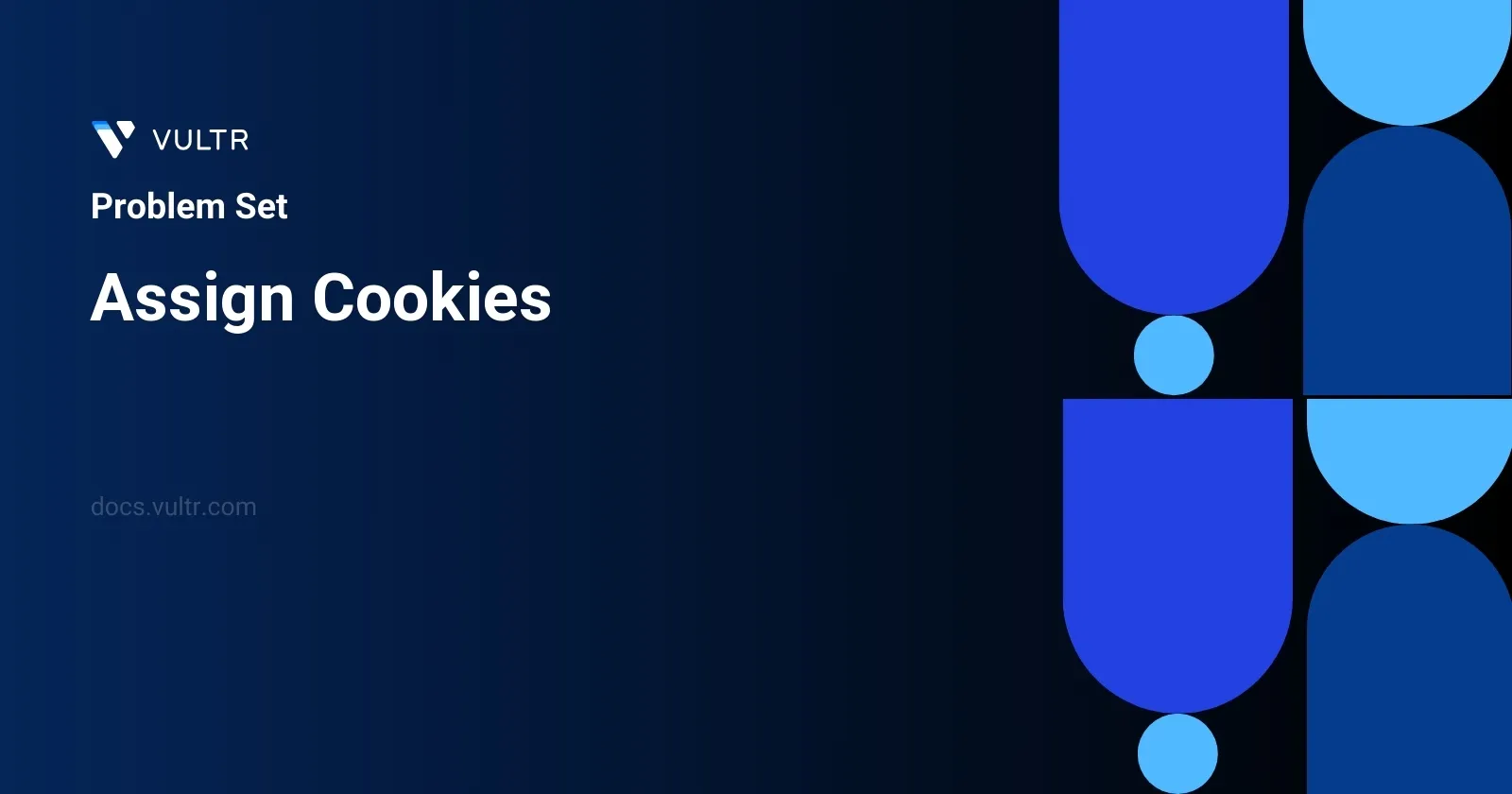
Problem Statement
In this task, imagine being a considerate parent who wants to distribute cookies to your children. Every child has what is referred to as a "greed factor"; this factor represents the minimum size of a cookie that will make them happy. With each child allowed just one cookie, your goal is to maximize the number of satisfied children by strategically distributing the available cookies. Each cookie has a specific size, and a child is satisfied if the size of the cookie they receive is greater than or equal to their greed factor. You should determine the maximum number of children that can be content with the cookies you have.
Examples
Example 1
Input:
g = [1,2,3], s = [1,1]
Output:
1
Explanation:
You have 3 children and 2 cookies. The greed factors of 3 children are 1, 2, 3. And even though you have 2 cookies, since their size is both 1, you could only make the child whose greed factor is 1 content. You need to output 1.
Example 2
Input:
g = [1,2], s = [1,2,3]
Output:
2
Explanation:
You have 2 children and 3 cookies. The greed factors of 2 children are 1, 2. You have 3 cookies and their sizes are big enough to gratify all of the children, You need to output 2.
Constraints
1 <= g.length <= 3 * 104
0 <= s.length <= 3 * 104
1 <= g[i], s[j] <= 231 - 1
Approach and Intuition
Sorting for Strategy: To efficiently assign cookies that satisfy as many children as possible, first think about sorting. By sorting both the children by their greed factors and the cookies by their sizes, you can implement a in-progress strategy.
Two-pointer Technique:
- Use two pointers: one (
i
) iterating through the children's greed factors, and the other (j
) iterating through the cookie sizes. - Start with the least greedy child and the smallest cookie. Move through the array of children and try to satisfy each child with the smallest available cookie that meets or exceeds the child’s greed factor.
- If a cookie satisfies a child, move both pointers to the next child and the next cookie, respectively. If it does not satisfy the child, move to the next bigger cookie while keeping the child pointer constant.
- This method ensures that each child gets the smallest possible cookie that they would be content with, leaving bigger cookies available for potentially greedier children.
- Use two pointers: one (
Optimization Insight:
- Sorting both arrays initially allows us to efficiently try the smallest available cookie for each child, optimizing our chances of satisfying a greater number of children.
The approach leverages a logical, incremental assignment of resources (cookies) to demands (children’s greed) in a manner that seeks to maximize efficiency (number of satisfied children) without wastage. By attending to the least greedy child first, this method ensures that we utilize the smallest appropriate resources, thus potentially leaving room for accommodating the needs of greedier children.
Solutions
- C++
- Java
- Python
class Solution {
public:
int satisfyChildren(std::vector<int>& children, std::vector<int>& cookies) {
std::sort(children.begin(), children.end());
std::sort(cookies.begin(), cookies.end());
int satisfied = 0;
int index = 0;
while (index < cookies.size() && satisfied < children.size()) {
if (cookies[index] >= children[satisfied]) {
satisfied++;
}
index++;
}
return satisfied;
}
};
The solution provided addresses the problem of assigning cookies to children in a way that maximizes the number of satisfied children. Each child has a minimum size of cookie they need in order to be satisfied. The approach taken here involves sorting both the list of children and cookies by size, which allows for a more efficient way of satisfying the maximum number of children with the available cookies.
In the given C++ code, you'll find a function named satisfyChildren
that accepts two vectors of integers: one representing the greed factors of the children (children
) and the other representing the sizes of the cookies (cookies
). Both vectors are sorted in ascending order to facilitate comparison.
The solution uses a simple while loop to iterate through the cookies, attempting to satisfy each child in sequence. The variable satisfied
counts the number of children that have been successfully given a cookie that meets or exceeds their greed factor. The index
is used to iterate over the cookies
array.
The function returns the total number of satisfied children once all possible comparisons are exhausted. The key to the efficiency of this algorithm lies in the sorting step, which ensures that the smallest available cookie that can satisfy a child is always tried first, minimizing the waste of larger cookies on children with smaller requirements. This greedy algorithm is effective for this type of matching problem and helps achieve an optimal solution under the constraints given.
class Solution {
public int distributeCookies(int[] children, int[] cookies) {
Arrays.sort(children);
Arrays.sort(cookies);
int satisfiedChildren = 0;
int index = 0;
while (index < cookies.length && satisfiedChildren < children.length) {
if (cookies[index] >= children[satisfiedChildren]) {
satisfiedChildren++;
}
index++;
}
return satisfiedChildren;
}
}
In the given Java solution, the goal is to determine how many children can be satisfied with the given cookies based on size preferences. Each child can be satisfied with a single cookie that meets or exceeds their size preference. Below is a summary of how the solution works:
- Start by sorting both the
children
andcookies
arrays to facilitate the greedy allocation where each child gets the smallest available cookie that meets their need. - Initialize variables to keep track of the number of satisfied children (
satisfiedChildren
) and the current index in the cookies array (index
). - Utilize a loop that continues as long as there are cookies left and not all children are satisfied. Within the loop:
- Compare the current cookie with the current child's size preference.
- If the size of the cookie satisfies the child's preference, increment the count of satisfied children and move to the next child.
- Always increment the cookie index to move to the next cookie after each check.
- Return the count of satisfied children as the result of the function.
This approach ensures that the maximum number of children are satisfied using the minimum necessary cookies through efficient comparison facilitated by sorting.
class Solution:
def countSatisfiedChildren(self, greed: List[int], cookies: List[int]) -> int:
greed.sort()
cookies.sort()
satisfied_count = 0
cookie_idx = 0
while cookie_idx < len(cookies) and satisfied_count < len(greed):
if cookies[cookie_idx] >= greed[satisfied_count]:
satisfied_count += 1
cookie_idx += 1
return satisfied_count
This solution tackles the problem of assigning cookies to children based on their greed factor. The objective is to maximize the number of satisfied children given arrays of greed factors and cookie sizes.
Here's how the code works:
- First, sort the lists for greed and cookies to streamline the comparison process.
- Initialize a counter
satisfied_count
to keep track of how many children have been satisfied with a cookie. - Use a while loop to traverse the list of cookies and attempt to satisfy each child in order of their greed. The loop continues as long as there are cookies available and there are still unsatisfied children.
- For each cookie, if it meets or exceeds the current child’s need (greed factor), increment the
satisfied_count
and move to the next child. - Return the total count of satisfied children.
This approach efficiently matches cookies to children with minimal waste, ensuring each child only receives a cookie if it satisfies their minimum requirement.
No comments yet.