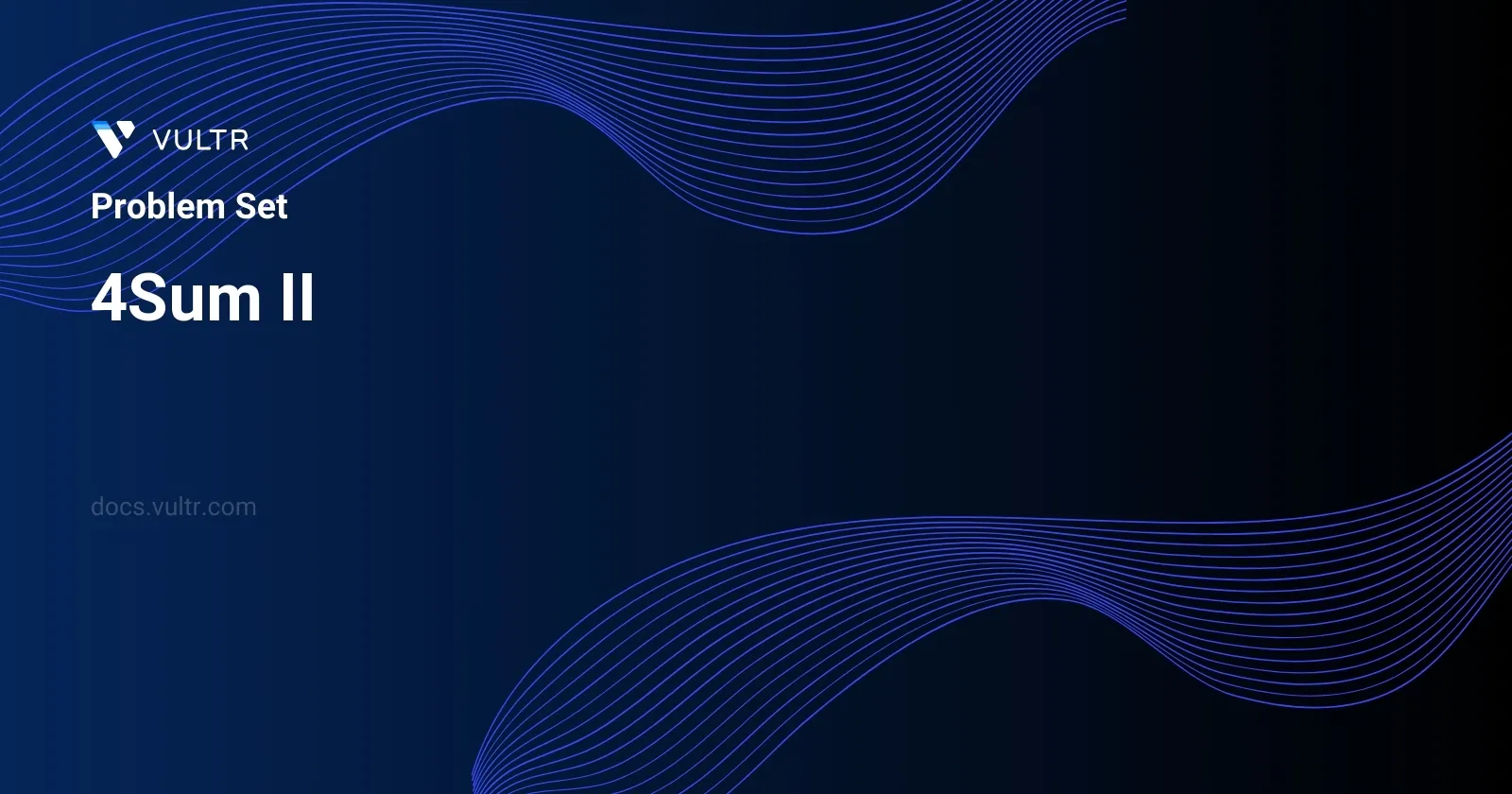
Problem Statement
In this problem, you are given four integer arrays: nums1
, nums2
, nums3
, and nums4
, each with the same length n
. Your task is to determine the number of tuples (i, j, k, l)
such that the following conditions are met:
- Each index value (
i
,j
,k
,l
) is within the range of0
ton-1
. - The sum of the elements at these indexes from each array equals zero, that is,
nums1[i] + nums2[j] + nums3[k] + nums4[l] == 0
.
The challenge lies in efficiently counting these tuples given the possible large size of each array (up to 200 elements).
Examples
Example 1
Input:
nums1 = [1,2], nums2 = [-2,-1], nums3 = [-1,2], nums4 = [0,2]
Output:
2
Explanation:
The two tuples are: 1. (0, 0, 0, 1) -> nums1[0] + nums2[0] + nums3[0] + nums4[1] = 1 + (-2) + (-1) + 2 = 0 2. (1, 1, 0, 0) -> nums1[1] + nums2[1] + nums3[0] + nums4[0] = 2 + (-1) + (-1) + 0 = 0
Example 2
Input:
nums1 = [0], nums2 = [0], nums3 = [0], nums4 = [0]
Output:
1
Constraints
n == nums1.length
n == nums2.length
n == nums3.length
n == nums4.length
1 <= n <= 200
-228 <= nums1[i], nums2[i], nums3[i], nums4[i] <= 228
Approach and Intuition
To tackle the problem of finding the tuples where the sum of elements from four different arrays equals zero, we consider the combinatorial nature of the problem and constraints:
A direct approach would involve generating all possible tuples
(i, j, k, l)
, computing their sums, and counting how many of those sums equal zero. However, this would result in an O(n^4) time complexity, which is impractical givenn
can be as large as 200.A more efficient approach utilizes hashing to reduce the time complexity. By noticing that
nums1[i] + nums2[j] = -(nums3[k] + nums4[l])
, we can separate the problem into two parts:- Compute all possible sums of pairs between
nums1
andnums2
, and store these sums in a hash map, where the key is the sum and the value is the count of how often this sum occurs. - Similarly, compute all possible sums of pairs between
nums3
andnums4
. For each sum, check if the negation of this sum exists in our previously constructed hash map fromnums1
andnums2
. If it does, it means there are pairs from the first two arrays and pairs from the last two arrays that together sum to zero. The number of such tuples is the product of the occurrences of these sums from the two different parts.
- Compute all possible sums of pairs between
This approach leveraging hash maps allows us to count the qualifying tuples in O(n^2) time, which is a significant improvement over the naïve method. This technique effectively reduces the problem complexity by handling two arrays at a time rather than dealing with all four simultaneously.
Solutions
- C++
- Java
- Python
class Solution {
public:
int sumOfFour(vector<int>& A, vector<int>& B, vector<int>& C, vector<int>& D) {
vector<vector<int>> listGroups = {A, B, C, D};
int n = int(listGroups.size());
auto computeCounts = [&](int startIdx, int endIdx) {
map<int, int> counts({{0, 1}});
for (int i = startIdx; i < endIdx; i++) {
map<int, int> tempCounts;
for (int element : listGroups[i]) {
for (auto it = counts.begin(); it != counts.end(); ++it) {
tempCounts[element + it->first] += it->second;
}
}
counts = tempCounts;
}
return counts;
};
map<int, int> half1Counts = computeCounts(0, n / 2);
map<int, int> half2Counts = computeCounts(n / 2, n);
int totalCount = 0;
for (auto it = half1Counts.begin(); it != half1Counts.end(); ++it) {
totalCount += it->second * half2Counts[-it->first];
}
return totalCount;
}
};
The provided C++ solution effectively solves the "4Sum II" problem by calculating the total number of tuples (i, j, k, l) from four lists A, B, C, and D such that the sum A[i] + B[j] + C[k] + D[l] is zero. The approach used in the solution involves dividing the problem into two parts using a two-point technique and leveraging the map
data structure to store intermediary sums and their counts, which significantly reduces the complexity compared to a naive approach.
The solution uses a nested function, computeCounts
, defined within sumOfFour
to facilitate the computation of sum counts between given index ranges in subarrays. Here's an overview of how the code accomplishes this:
- Split the lists into two halves; compute sum counts for each half.
computeCounts
operates by iterating over the selected subarray elements and updatesmap
entries where keys are the possible sums and values are their occurrence counts.- This function uses nested loops to iterate over the elements of the lists and the current sums in the
map
to generate new possible sums, updating the count each time a new sum is generated. - For combining the results, iterate through the sums computed from the first half of the lists. For each sum, find the corresponding negative sum in the second half's computed results to check how many ways the total sum can be zero. Multiply the occurrence counts from both halves for each valid sum pair to get the total number of tuples that sum to zero.
In summary, the code effectively reduces the computational complexity by breaking down the problem into manageable parts, utilizing maps for efficient sum lookup and aggregation. This approach ensures a much faster execution time compared to a brute-force method. The separation of concerns and clear logical flow also aids in understanding and maintaining the code.
import java.util.HashMap;
class Solver {
private int[][] numbersArray;
public int computeFourSumCount(int[] A, int[] B, int[] C, int[] D) {
numbersArray = new int[][]{A, B, C, D};
int length = numbersArray.length;
Map<Integer, Integer> firstHalf = calculateSums(0, length / 2);
Map<Integer, Integer> secondHalf = calculateSums(length / 2, length);
int result = 0;
for (int sum : firstHalf.keySet())
result += firstHalf.get(sum) * secondHalf.getOrDefault(-sum, 0);
return result;
}
private Map<Integer, Integer> calculateSums(int start, int end) {
Map<Integer, Integer> countMap = new HashMap<>();
countMap.put(0, 1);
for (int i = start; i < end; i++) {
Map<Integer, Integer> tempMap = new HashMap<>();
for (int element : numbersArray[i]) {
for (int sum : countMap.keySet()) {
tempMap.put(sum + element, tempMap.getOrDefault(sum + element, 0) + countMap.get(sum));
}
}
countMap = tempMap;
}
return countMap;
}
}
The given Java code presents a method to solve the "4Sum II" problem, which involves finding tuples from four separate arrays (A, B, C, D) such that their sum totals to zero. The solution utilizes a hashmap-based approach to efficiently count combinations of sums in two divided half segments of the combined arrays.
- Create a private 2D integer array
numbersArray
to store input arrays A, B, C, and D. - Define a method
computeFourSumCount
which:- Initializes a
Map
namedfirstHalf
built bycalculateSums
for the first two arrays. - Initializes a
Map
namedsecondHalf
built bycalculateSums
for the last two arrays. - Combines results from these maps, adding product of matched sums from
firstHalf
against their negatives insecondHalf
(reflecting the required zero sum).
- Initializes a
- Implement
calculateSums
, a helper method which:- Initializes a hashmap
countMap
counting ways to achieve various sums. - Iteratively explores possible sum combinations through nested loops and updates
countMap
using another temporary maptempMap
.
- Initializes a hashmap
By decomposing the problem into two parts and using hashmaps to store intermediate results, this solution improves efficiency and manages complexity compared to a straightforward four-array nested loop approach, significantly optimizing the calculation of four sum combinations to zero.
from collections import Counter
class FourNumberSum:
def computeFourSumCount(self, nums1: List[int], nums2: List[int], nums3: List[int], nums4: List[int]) -> int:
def computeSumCounts(lists: List[List[int]]) -> Counter:
result = Counter({0: 1})
for group in lists:
temp_counter = Counter()
for value in group:
for existing_sum in result:
temp_counter[existing_sum + value] += result[existing_sum]
result = temp_counter
return result
grouped_lists = [nums1, nums2, nums3, nums4]
half = len(grouped_lists) // 2
left_part, right_part = computeSumCounts(grouped_lists[:half]), computeSumCounts(grouped_lists[half:])
return sum(left_part[sum_val] * right_part[-sum_val] for sum_val in left_part)
The given Python3 code defines a class FourNumberSum
which is used to solve the problem of finding the count of tuples that can be formed from four given lists of integers (nums1
, nums2
, nums3
, nums4
). Each tuple's sum must be zero. The solution employs a divide and conquer approach along with the use of hash tables to efficiently sum combinations and count occurrences.
The class contains a method
computeFourSumCount
, which is the main function to compute the count of valid four-number tuples.Inside this method,
computeSumCounts
is a helper function used to compute hash maps (Counter
from thecollections
module) representing all possible sums of subdivided list combinations and their respective counts.The initial list of numbers is divided into two halves (
grouped_lists[:half]
andgrouped_lists[half:]
). Each half is processed separately to create two counters (left_part
andright_part
) that store all possible sums and their counts.Finally, a comprehensive count is determined by traversing through each possible sum in the
left_part
and checking if the negative of that sum exists in theright_part
. The result is the multiplication of corresponding counts (number of ways the sum from the first half can be matched with sums from the second half to result in zero).
This efficient combination of hash tables and divide-and-conquer reduces the complexity compared to the straightforward method (O(n^4)). Here, handling subdivided tasks separately and only focusing on matching sums greatly improves performance.
No comments yet.