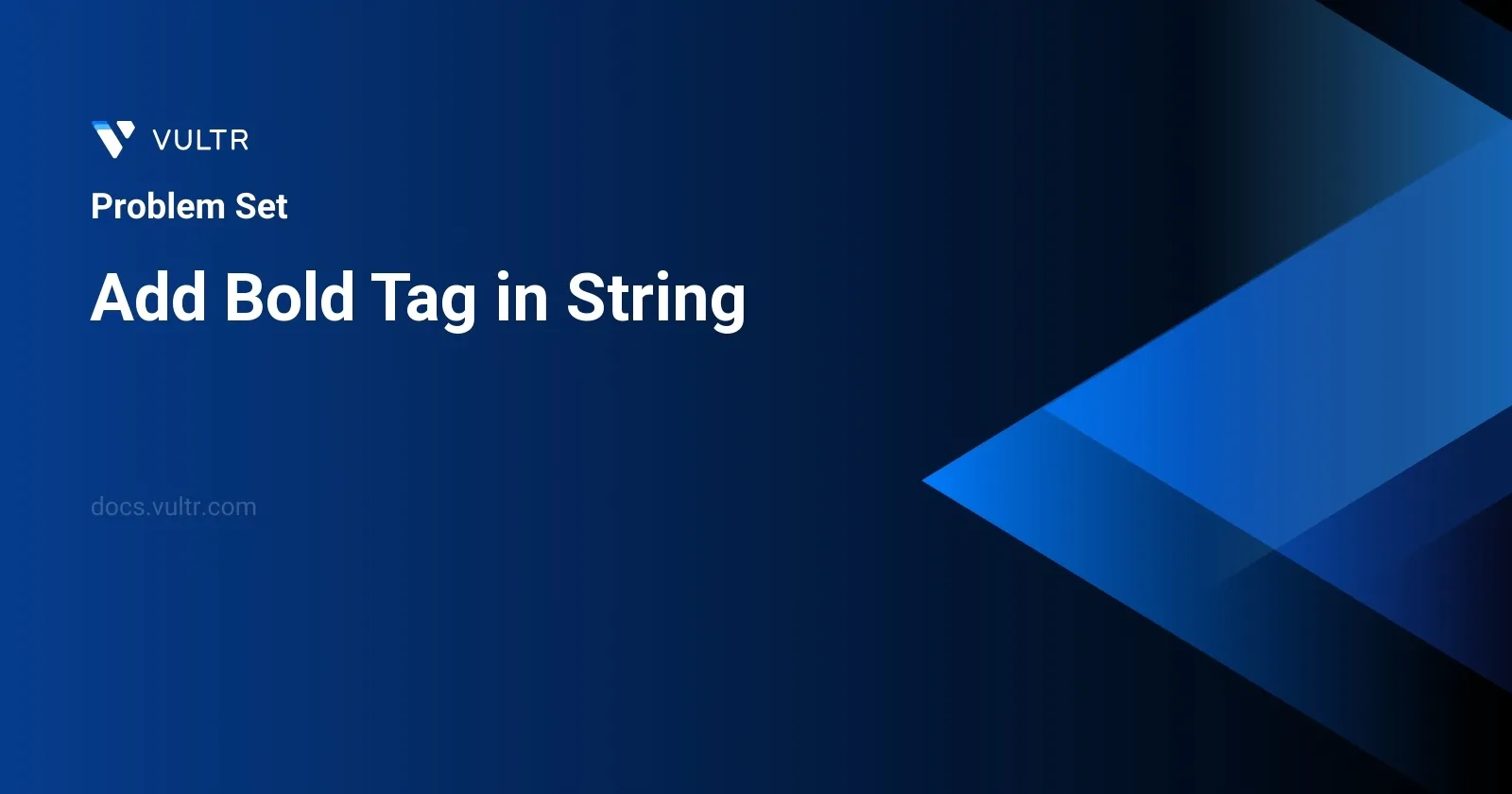
Problem Statement
Given a string s
and a list of substrings named words
, the task is to wrap certain substrings of s
that appear in words
with HTML bold tags (<b>
and </b>
). This wrapping must respect a few conditions: if two substrings from words
that are present in s
overlap, they should be wrapped together under a single pair of tags. Furthermore, if two such substrings are directly adjacent in s
, their wrapping should also be merged into one continuous bold section. The desired outcome is a new version of the string s
with these modifications applied where appropriate.
Examples
Example 1
Input:
s = "abcxyz123", words = ["abc","123"]
Output:
"abcxyz123"
Explanation:
The two strings of words are substrings of s as following: "abcxyz123". We add before each substring and after each substring.
Example 2
Input:
s = "aaabbb", words = ["aa","b"]
Output:
"aaabbb"
Explanation:
"aa" appears as a substring two times: "aaabbb" and "aaabbb". "b" appears as a substring three times: "aaabbb", "aaabbb", and "aaabbb". We add before each substring and after each substring: "aaabbb". Since the first two 's overlap, we merge them: "aaabbb". Since now the four 's are consecutive, we merge them: "aaabbb".
Constraints
1 <= s.length <= 1000
0 <= words.length <= 100
1 <= words[i].length <= 1000
s
andwords[i]
consist of English letters and digits.- All the values of
words
are unique.
Approach and Intuition
To effectively add bold tags around substrings in s
which are found in words
while considering overlaps and adjacent positions, you can utilize the following steps:
- Identify each substring in
s
that matches any string inwords
and record their start and end indices ins
. - Sort these index ranges ensuring order by start index, and, if start indices are the same, by end indices (descending).
- Initialize a merged range list and iteratively merge overlapping or adjacent ranges:
- For each sorted index range, determine if it can be merged with the last range in the merged list (i.e., if they overlap or touch).
- If they can be merged, update the last range in the list to encompass both.
- Otherwise, append the new range to the list.
- Finally, utilizing the merged index ranges, construct the resulting string:
- Traverse through
s
, inserting<b>
at the start of each range and</b>
at the end. Adjust indices for insertion points accordingly as you modify the string length with tags.
- Traverse through
By implementing the above algorithm, the completed string s
will have substrings correctly wrapped in bold tags, ensuring that all specified conditions (overlapping and adjacency) are respected. The approach ensures an efficient traversal and merging of index ranges, avoiding excessive recomputation or unnecessary string concatenations.
Solutions
- C++
- Java
- Python
class Solution {
public:
string encloseBold(string str, vector<string>& dict) {
int len = str.length();
vector<bool> isBold(len);
for (string word : dict) {
int initPos = str.find(word);
while (initPos != -1) {
for (int j = initPos; j < initPos + word.length(); j++) {
isBold[j] = true;
}
initPos = str.find(word, initPos + 1);
}
}
string openTag = "<b>";
string closeTag = "</b>";
string result = "";
for (int k = 0; k < len; k++) {
if (isBold[k] && (k == 0 || !isBold[k - 1])) {
result += openTag;
}
result += str[k];
if (isBold[k] && (k == len - 1 || !isBold[k + 1])) {
result += closeTag;
}
}
return result;
}
};
The provided C++ solution involves wrapping specified substrings (as listed in a dictionary) with bold HTML tags in a given string. Here's an overview of how this solution functions:
- Initially, the solution defines a function
encloseBold
that takes a string and a vector of strings (dictionary) as its parameters. The primary purpose here is to return the string with specified substrings highlighted in bold using HTML tags. - A boolean vector named
isBold
tracks which characters in the original string need to be bold. This vector's size is equal to the length of the input string, initially set to false, indicating no characters are yet marked for bolding. - The process iterates through each word in the dictionary and locates its occurrence(s) in the input string using the
find
method. For each start position (initPos
) found for a word, the corresponding indices inisBold
are set to true, representing these characters should be bolded. - As each position in the input string is checked, if a switch from non-bold to bold or vice versa is detected, an appropriate HTML tag (
<b>
for starting bold and</b>
for ending bold) is added to the result string. - This bold marking continues, with the function appending each character of the original string to the result, adding close tags as necessary based on the
isBold
vector values.
This code effectively handles edge cases, such as overlapping bold sections and instances where the string starts or ends with a bold section. The use of a boolean vector provides a clear and efficient way to manage the placement of HTML tags within the string.
class Solution {
public String wrapWithBold(String text, String[] dictionary) {
int length = text.length();
boolean[] isBold = new boolean[length];
for (String dictWord: dictionary) {
int idx = text.indexOf(dictWord);
while (idx != -1) {
for (int j = idx; j < idx + dictWord.length(); j++) {
isBold[j] = true;
}
idx = text.indexOf(dictWord, idx + 1);
}
}
String startTag = "<b>";
String endTag = "</b>";
StringBuilder finalResult = new StringBuilder();
for (int i = 0; i < length; i++) {
if (isBold[i] && (i == 0 || !isBold[i - 1])) {
finalResult.append(startTag);
}
finalResult.append(text.charAt(i));
if (isBold[i] && (i == length - 1 || !isBold[i + 1])) {
finalResult.append(endTag);
}
}
return finalResult.toString();
}
}
The provided Java code defines a method called wrapWithBold
in the Solution
class, which implements functionality to add HTML bold tags <b>...</b>
around substrings of a given text. These substrings match any of the words in the provided dictionary array. The solution efficiently identifies and wraps the relevant parts of the text using boolean marking and builds the final string with the bold tags appropriately inserted.
- Begin with creating an array
isBold
to mark characters in the inputtext
that should be bolded. The length of this array is set to the length of thetext
. - Iterate over each word in the
dictionary
to determine if it exists intext
. For each found word, mark its characters as bold in theisBold
array. - Utilize a loop to construct the resultant string using
StringBuilder
. Check theisBold
array to determine when to insert<b>
and</b>
. - If the current character needs to start a bold sequence (
<b>
), and either it's the first character or the previous one was not bold, append<b>
tofinalResult
. - Add the current character from the text to
finalResult
. - If the current character ends a bold sequence (
</b>
), and either it's the last character or the next one is not to be bold, append</b>
tofinalResult
. - Return the string representation of
finalResult
which includes the text with bold tags added around the specified substrings.
This code offers a precise method for dynamically adding bold formatting around specific dictionary words found within a string, making it very useful in scenarios where text formatting based on content is required.
class Formatter:
def applyBold(self, text: str, keywords: List[str]) -> str:
length = len(text)
is_bold = [False] * length
for keyword in keywords:
position = text.find(keyword)
while position != -1:
for index in range(position, position + len(keyword)):
is_bold[index] = True
position = text.find(keyword, position + 1)
bold_start = "<b>"
bold_end = "</b>"
result = []
for idx in range(length):
if is_bold[idx] and (idx == 0 or not is_bold[idx - 1]):
result.append(bold_start)
result.append(text[idx])
if is_bold[idx] and (idx == length - 1 or not is_bold[idx + 1]):
result.append(bold_end)
return "".join(result)
This solution involves a Python class named Formatter
that includes a method applyBold
designed to add HTML bold tags around parts of a string based on specified keywords. This function processes the input string and list of keywords to determine which segments should be bolded. Here’s how it does this:
- Initialize a boolean list
is_bold
to keep track of which characters in the input string should be in bold. - Loop through each keyword and mark the appropriate positions in the
is_bold
list asTrue
for the length of each keyword found in the text. - Use two string variables,
bold_start
andbold_end
, to represent the HTML tags for starting and ending bold text. - Create a result list that will build the final string. As you iterate over each character in the input string, check the
is_bold
state to decide whether to append a bold start or end tag before or after the character. - Finally, join the elements of the result list into a single string which now contains the HTML-bolded terms as specified, and return this string.
Key points:
- Utilization of
find
function helps in efficiently locating the positions of each keyword in the text. - Careful handling of edge cases where bold tags should start or end, ensuring no overlapping tags or unnecessary tags are added.
- The final result combines text and HTML tags appropriately, creating a visually formatted string as per the requirements given through the keywords list.
No comments yet.