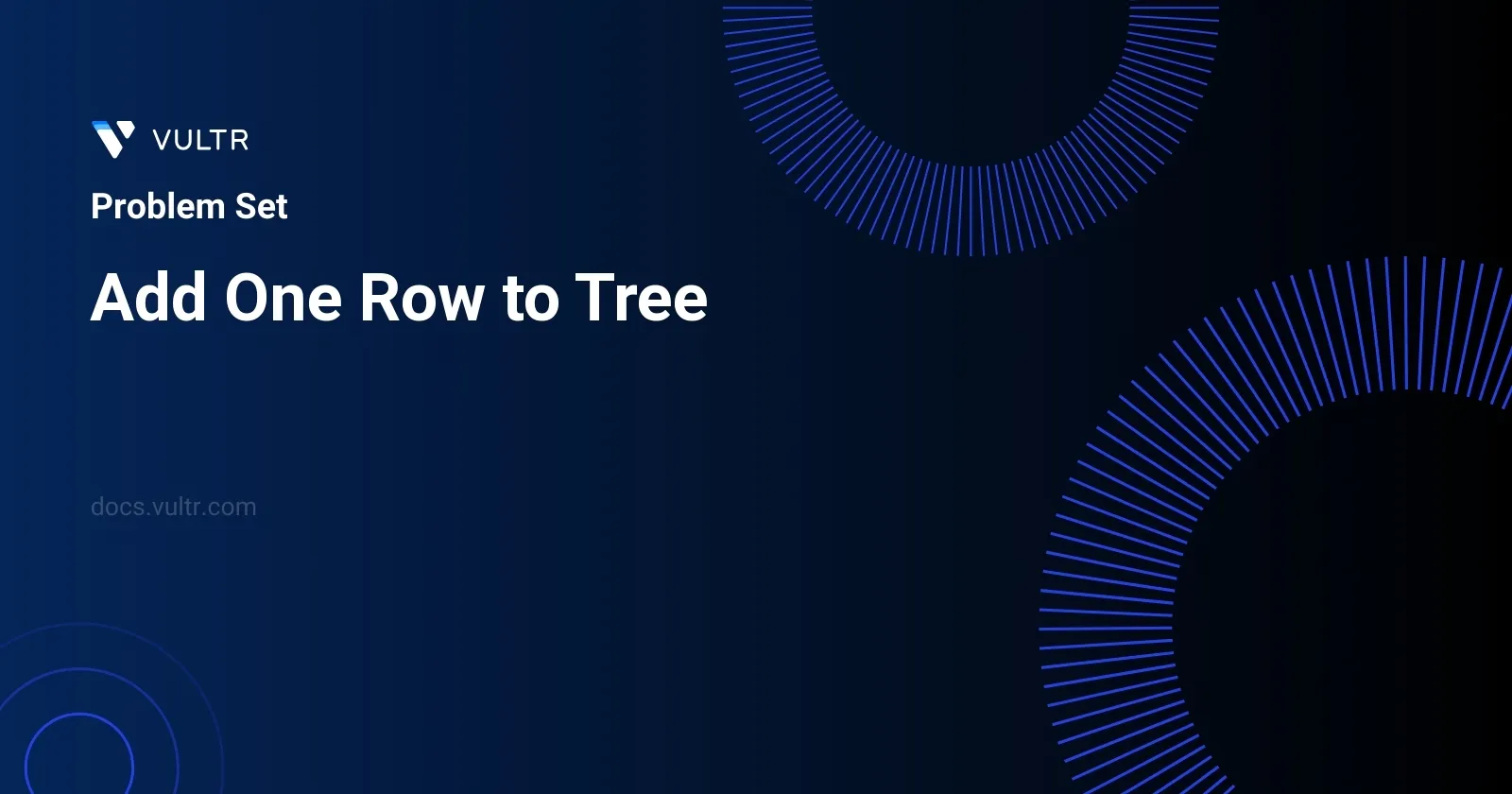
Problem Statement
Given a binary tree's root node and two integers, val
and depth
, the task is to insert a row of nodes into the tree such that each node in the new row has the value val
and is positioned at the specified depth
. Remember, the depth of the root node is 1.
To accomplish this, follow these rules:
- For each existing node
cur
at the depthdepth - 1
, insert two new tree nodes, both having the valueval
. - Set these new nodes as the left and right children of
cur
. The original left subtree ofcur
should now branch off the new left node, and similarly, the original right subtree should attach to the new right node. - In a special case where
depth
is 1, meaning the new nodes should directly follow the root, transform the current root into the left child of a new root node that holds the valueval
.
Examples
Example 1
Input:
root = [4,2,6,3,1,5], val = 1, depth = 2
Output:
[4,1,1,2,null,null,6,3,1,5]
Example 2
Input:
root = [4,2,null,3,1], val = 1, depth = 3
Output:
[4,2,null,1,1,3,null,null,1]
Constraints
- The number of nodes in the tree is in the range
[1, 104]
. - The depth of the tree is in the range
[1, 104]
. -100 <= Node.val <= 100
-105 <= val <= 105
1 <= depth <= the depth of tree + 1
Approach and Intuition
To successfully add a new row of nodes to a binary tree at a specified depth
and with a specific val
, let's break down the steps based on whether the new row is to be added at the root or at a deeper level:
Determine if the new depth is at the root level:
- If
depth
equals 1, create a new root node with the valueval
. Make the existing tree the left subtree of this new node. - This handles cases where inserting a row of nodes directly beneath the very top of the tree, i.e., beneath the
root
, is required, effectively pushing the entire tree down.
- If
Insert the row at a depth other than the root:
- Employ a breadth-first search (BFS) traversal up to
depth - 1
. - For each node encountered:
- Initiate two new nodes, both with the value
val
. - Attach the original left child of the current node to the new left node.
- Attach the original right child of the current node to the new right node.
- Set these new nodes as the left and right children of the current node, respectively.
- Initiate two new nodes, both with the value
- This approach ensures that we efficiently navigate through the tree to precisely the level right before where the insertion is supposed to occur.
- Employ a breadth-first search (BFS) traversal up to
These operations adhere to the constraints and ensure that we are modifying the tree's structure by inserting nodes only where specified, without affecting other portions of the tree's structure or properties.
Solutions
- Java
public class Solution {
public TreeNode insertNewRow(TreeNode root, int value, int depth) {
if (depth == 1) {
TreeNode newNode = new TreeNode(value);
newNode.left = root;
return newNode;
}
Queue<TreeNode> currentLevel = new LinkedList<>();
currentLevel.add(root);
int currentDepth = 1;
while (currentDepth < depth - 1) {
Queue<TreeNode> nextLevel = new LinkedList<>();
while (!currentLevel.isEmpty()) {
TreeNode currNode = currentLevel.poll();
if (currNode.left != null) nextLevel.add(currNode.left);
if (currNode.right != null) nextLevel.add(currNode.right);
}
currentLevel = nextLevel;
currentDepth++;
}
while (!currentLevel.isEmpty()) {
TreeNode currNode = currentLevel.poll();
TreeNode leftNode = currNode.left;
currNode.left = new TreeNode(value);
currNode.left.left = leftNode;
leftNode = currNode.right;
currNode.right = new TreeNode(value);
currNode.right.right = leftNode;
}
return root;
}
}
The Java solution provided offers a method to add a new row of nodes with a specified value at a given depth in a binary tree. The method insertNewRow
takes three parameters: the root of the binary tree, the integer value for the new nodes, and the depth at which the new row should be inserted.
Here's a breakdown of how the method works:
If the specified depth is 1, the method creates a new node with the given value, placing the existing tree under this new node, and returns this node as the new root.
To reach the desired level just before where the new row will be inserted, it utilises a Breadth-First Search approach utilizing a Queue to traverse the tree level by level.
The tree traversal continues until reaching the level just above the target depth. At this level, the following operations take place:
- It iterates over each node at this level.
- For each node, it temporarily stores its current left and right children.
- It then creates new nodes (with the specified value) that become the new left and right children of the current node.
- The original children are reattached to the newly created left and right nodes appropriately.
Finally, it returns the potentially new root of the tree (if the depth was 1), effectively adding a new row at the required depth.
This implementation is efficient because each node in the tree is processed exactly once, making the time complexity proportional to the number of nodes in the tree. The space complexity is also optimized for the breadth of the tree, due to storage requirements for the queue during traversal.
No comments yet.