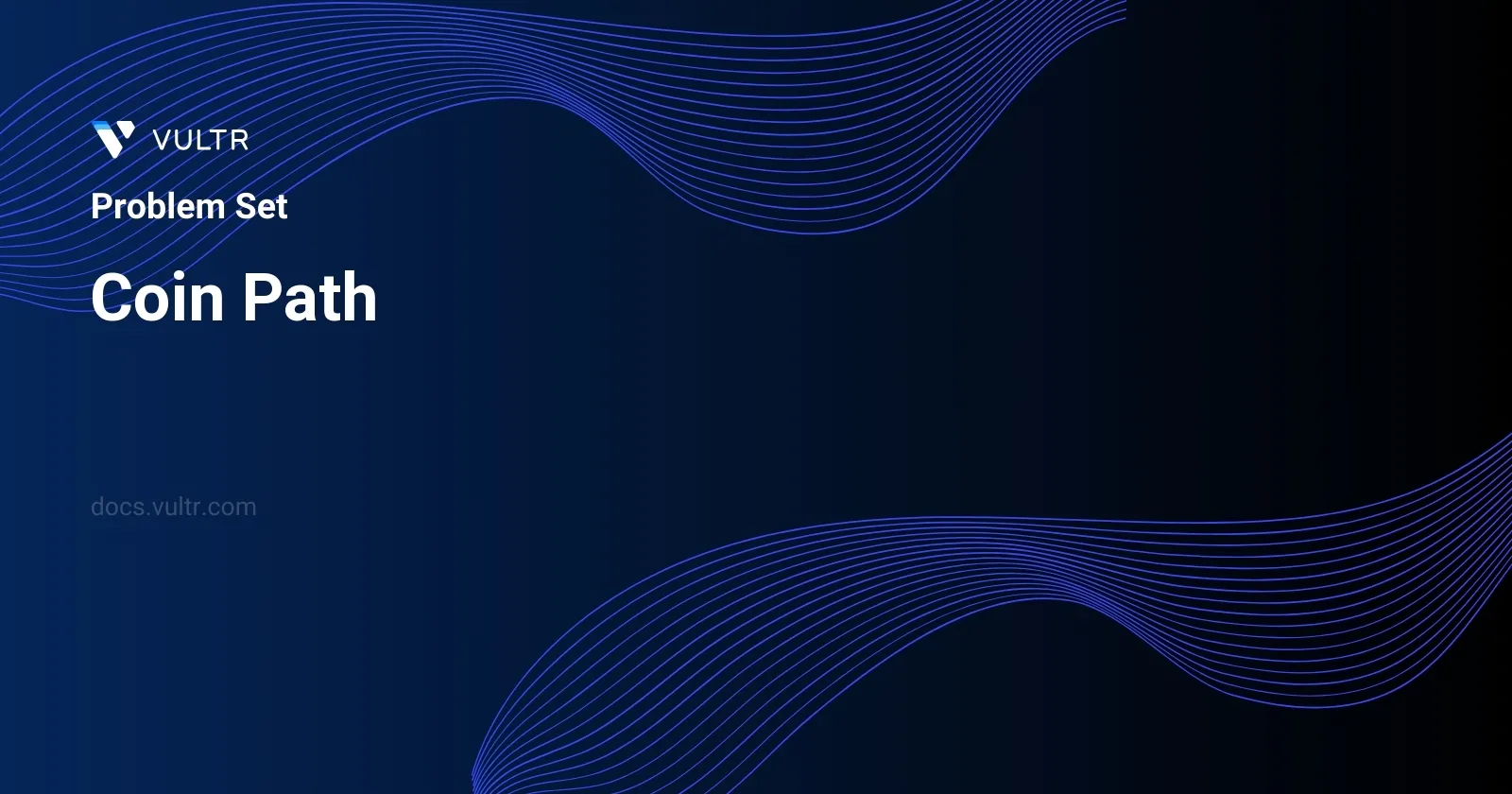
Problem Statement
In this problem, you are given an integer array named coins
, which is 1-indexed and represents the cost of landing on each index. Additionally, you are provided with an integer maxJump
which determines the maximum range of indices you can jump forward from your current position. You start your journey from the index 1, which is guaranteed to be accessible (coins[1] != -1
).
Your goal is to determine the sequence of indices you can follow to reach the last index (n
) of the array with the minimum possible cost. It is crucial to note that you can only jump to an index if its value is not -1
, as this represents an inaccessible position.
The result should be the path of indices, denoted as an integer array, that reflects the minimal cost route to index n
. If multiple paths yield the same cost, you must return the sequence that is lexicographically smallest. In case reaching the final index is impossible, the function should return an empty array.
Examples
Example 1
Input:
coins = [1,2,4,-1,2], maxJump = 2
Output:
[1,3,5]
Example 2
Input:
coins = [1,2,4,-1,2], maxJump = 1
Output:
[]
Constraints
1 <= coins.length <= 1000
-1 <= coins[i] <= 100
coins[1] != -1
1 <= maxJump <= 100
Approach and Intuition
To solve this problem, an understanding of dynamic programming in the context of path-finding and cost minimization is crucial. Additionally, the preference for lexicographically smallest paths when costs are equivalent introduces a layer of complexity that must be carefully managed. Here’s a step-by-step breakdown of the approach:
Understand the problem and constraints:
- You start at index 1 and aim to reach the last index with minimum cost.
- You can jump forward from an index
i
toi+k
, where1 ≤ k ≤ maxJump
ifi+k
is within bounds andcoins[i+k] != -1
. - You need to ensure the path obtained is the lexicographically smallest one in cases with similar costs.
Initial Setup:
- Use a dynamic programming array
dp
wheredp[i]
keeps track of the minimum cost to reach indexi
from index 1. - Use another array
path
to store the actual path (sequence of indices) taken to reach every index.
- Use a dynamic programming array
Dynamic Programming Transition:
- For each index
i
, if it's accessible (i.e.,coins[i] != -1
), updatedp[i+k]
for all1 ≤ k ≤ maxJump
, ensuring that you're not going out of bounds. - Update the cost and path based on comparisons: if moving to
i+k
gives a lesser cost or if the costs are equal but the new path is lexicographically smaller.
- For each index
Lexicographical Consideration:
- While updating paths, always ensure to choose the path that sorts lexicographically smaller in cases where multiple paths result in the same cost.
Result Compilation:
- After filling the
dp
table, the minimum cost to reach the last index will be atdp[n]
. The path leading to this result will be stored inpath[n]
.
- After filling the
This approach essentially maps out optimal paths while keeping track of costs and ensures that in case of ties in cost, the path chosen favors lexicographical precedence. This kind of problem is typical in route optimization scenarios often found in network routing, logistics, and even game development.
Solutions
- Java
public class Solution {
public List<Integer> findCheapestPath(int[] costs, int maxStep) {
int[] nextStep = new int[costs.length];
long[] minCosts = new long[costs.length];
Arrays.fill(nextStep, -1);
List<Integer> path = new ArrayList<>();
for (int i = costs.length - 2; i >= 0; i--) {
long globalMin = Integer.MAX_VALUE;
for (int j = i + 1; j <= Math.min(i + maxStep, costs.length - 1); j++) {
if (costs[j] >= 0) {
long currentCost = costs[i] + minCosts[j];
if (currentCost < globalMin) {
globalMin = currentCost;
nextStep[i] = j;
}
}
}
minCosts[i] = globalMin;
}
int pos;
for (pos = 0; pos < costs.length && nextStep[pos] > 0; pos = nextStep[pos])
path.add(pos + 1);
if (pos == costs.length - 1 && costs[pos] >= 0)
path.add(costs.length);
else
return new ArrayList<Integer>();
return path;
}
}
The provided Java code defines a method findCheapestPath
within a class named Solution
. This method is designed to determine the most cost-effective path for a coin game where each position in the array costs
represents the cost of stepping on that position, and maxStep
specifies the maximum number of steps that can be taken in one move. The goal is to move from the start of the array to the end, minimizing the total cost.
- Initialize two arrays:
nextStep
, which keeps track of the next step index that leads to a minimum cost path from each position.minCosts
, which stores the minimum costs accumulated to reach each position from the start.
- Start from the second last element of the array and compute the minimum cost for each position, considering all possible steps within the range defined by
maxStep
. - For each viable position j within the range from the current position i:
- Calculate the total cost if moved from i to j.
- Update
minCosts[i]
if this calculated cost is lesser than the previously found global minimum, and record the step innextStep[i]
.
- Construct the path:
- Start from the first position, and navigate through positions recorded in
nextStep
array. Add the position to the result listpath
. - Continue this until the last position is reached or no further steps are possible.
- Start from the first position, and navigate through positions recorded in
- If the last position is part of the path and its cost is non-negative, conclude the path. If not, return an empty list.
- Return the path, where each element in the list represents a position index (1-indexed).
This solution optimally finds a way to traverse the costs
array with a constraint of maximum allowable steps, and ensures that the path is both feasible (exists within given conditions) and cost-effective.
No comments yet.