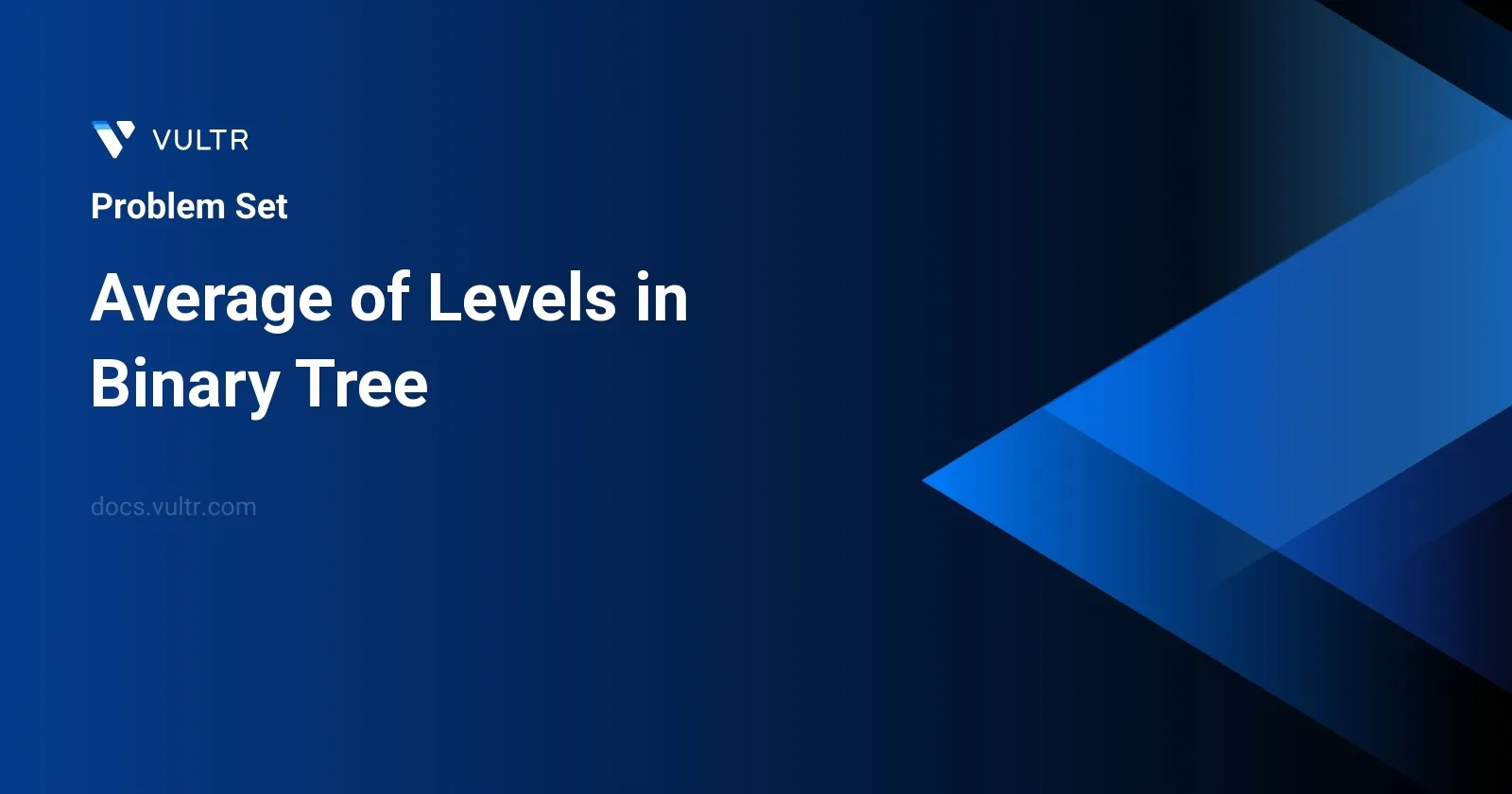
Problem Statement
In this task, you are given the root of a binary tree. Your goal is to compute the average value of the nodes at each level of the tree and return these averages as an array. The average for each level should be accurately calculated to a precision where deviations of up to 10^-5
from the actual average are considered acceptable. This problem tests your understanding of tree traversal techniques and how to manage data at different tree levels.
Examples
Example 1
Input:
root = [3,9,20,null,null,15,7]
Output:
[3.00000,14.50000,11.00000] Explanation: The average value of nodes on level 0 is 3, on level 1 is 14.5, and on level 2 is 11. Hence return [3, 14.5, 11].
Example 2
Input:
root = [3,9,20,15,7]
Output:
[3.00000,14.50000,11.00000]
Constraints
- The number of nodes in the tree is in the range
[1, 104]
. -231 <= Node.val <= 231 - 1
Approach and Intuition
To solve this problem, you’ll need to traverse through each level of the binary tree. The best strategy for this is using the Breadth-First Search (BFS) technique. This approach involves using a queue to keep track of nodes at the current level and to access their children.
Here's the plan:
- Start with the root node and initialize a queue.
- For each level:
- Calculate the total of the node values and count the nodes to derive the average for that level.
- Append all children of the nodes at the current level to the queue.
- Proceed to compute the average and move to the next level.
This method ensures that each node and its children are visited in level order, making the computation of averages straightforward.
The idea is simple but efficient, leveraging the nature of BFS to access each level's nodes in sequence and performing the necessary calculations for the average. Despite the large range of possible node values and tree sizes as highlighted in the constraints section, the BFS approach efficiently handles the tree level by level, ensuring that memory and computation are managed properly.
Solutions
- Java
public class Solution {
public List<Double> levelAverages(TreeNode rootNode) {
List<Double> averages = new ArrayList<>();
Queue<TreeNode> currentLevel = new LinkedList<>();
currentLevel.add(rootNode);
while (!currentLevel.isEmpty()) {
long levelSum = 0, levelCount = 0;
Queue<TreeNode> nextLevel = new LinkedList<>();
while (!currentLevel.isEmpty()) {
TreeNode node = currentLevel.poll();
levelSum += node.val;
levelCount++;
if (node.left != null)
nextLevel.add(node.left);
if (node.right != null)
nextLevel.add(node.right);
}
currentLevel = nextLevel;
averages.add((double) levelSum / levelCount);
}
return averages;
}
}
The provided Java code defines a method levelAverages
which calculates the average values of each level in a binary tree. Here's how the method accomplishes this task:
- Initialize a list
averages
to store the average of values at each level of the tree. - Use a queue
currentLevel
to manage nodes at the current level, starting with the root node. - A
while
loop continues as long as there are nodes at the current level to process.- Declare variables
levelSum
andlevelCount
to keep track of the sum of node values and the count of nodes at the current level. - Use a temporary queue
nextLevel
to hold the children of nodes being processed. - Inside another
while
loop, nodes are dequeued fromcurrentLevel
:- Add the node value to
levelSum
. - Increment
levelCount
. - Add non-null left and right children to
nextLevel
.
- Add the node value to
- After processing all nodes at the current level, calculate the average and add it to the
averages
list. - Assign
nextLevel
tocurrentLevel
to move to the next level of the tree.
- Declare variables
The method finishes by returning the averages
list, which now contains the average of node values for each tree level.
No comments yet.