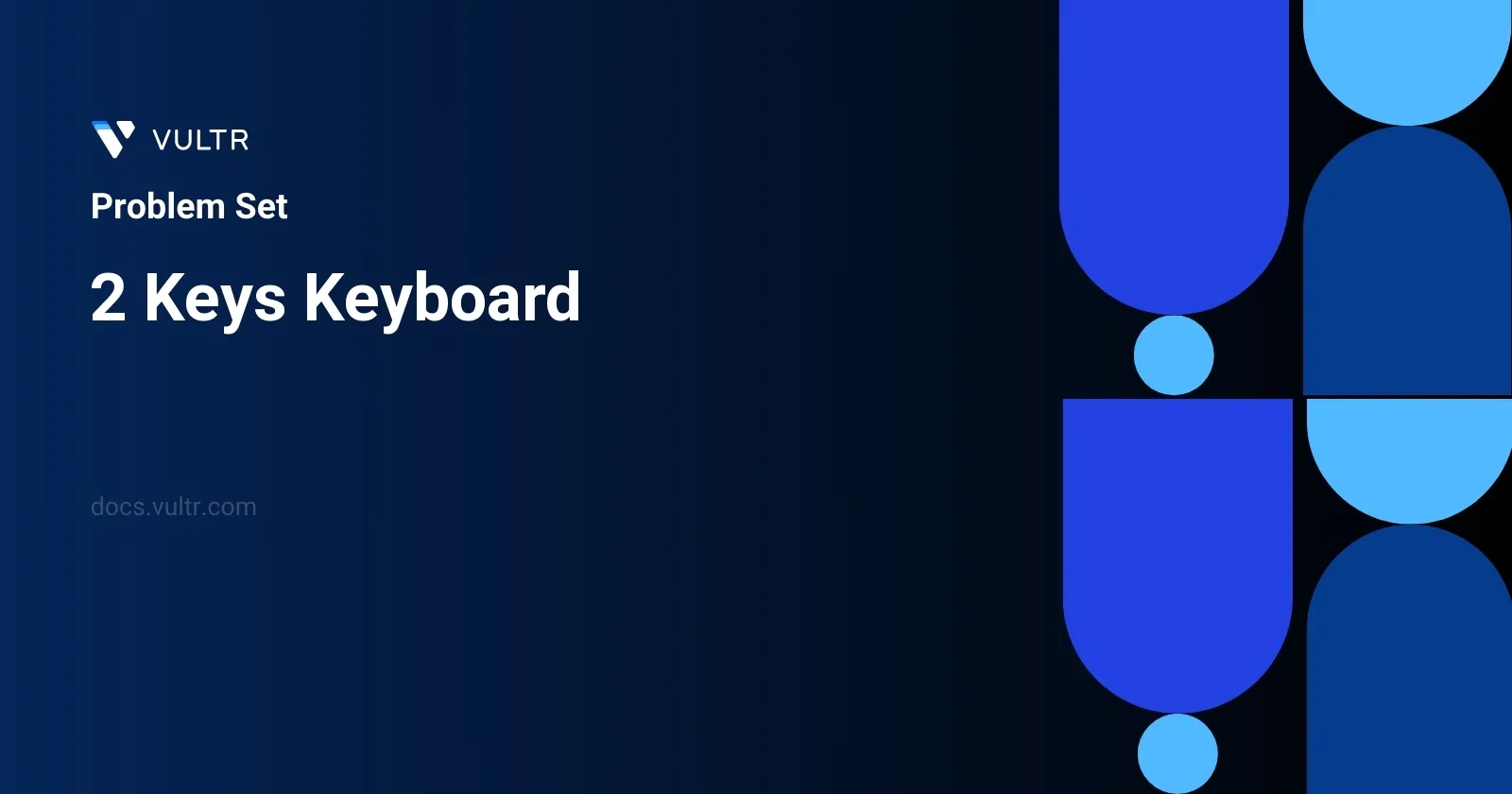
Problem Statement
In this problem, we start with a single occurrence of the character 'A' displayed on a notepad. To manipulate this notepad, we have two operations at our disposal:
- Copy All: This operation allows you to copy all characters currently visible on the screen. It must be noted that only a full copy of all characters is allowed, not just a partial selection.
- Paste: This operation pastes the last copied set of characters onto the screen.
Given a target integer n
, the challenge is to find the minimum number of operations required to have n
instances of 'A' on the screen. The operations should be optimally chosen to meet the goal with as few steps as possible.
Examples
Example 1
Input:
n = 3
Output:
3
Explanation:
Initially, we have one character 'A'. In step 1, we use Copy All operation. In step 2, we use Paste operation to get 'AA'. In step 3, we use Paste operation to get 'AAA'.
Example 2
Input:
n = 1
Output:
0
Constraints
1 <= n <= 1000
Approach and Intuition
To understand the minimum number of operations required to replicate 'A' on the notepad to reach exactly n
iterations, we can examine a few scenarios and develop an intuition based on the constraints:
If
n
is 1, you start with one 'A'. No operations are needed since you're already at the desired state.If
n
is greater than 1, explore the factorization ofn
:- Every factor of
n
represents a potential breakpoint where a Copy All + Paste operation sequence might be optimized. For instance, ifn
is 6, one of the optimal ways is to get to 3 'A's through minimal operations and then just duplicate that twice. - Specifically, for any factor
f
ofn
wheref
is less thann
, one can split this into replicatingf
'A's and then using the operations to double, triple, etc., until reachingn
by multiplyingf
. The number of operations this requires corresponds to the number of timesf
must be pasted (which isn / f
) plus the operations needed to reachf
initially.
- Every factor of
To determine the fewest operations, iterate through potential factors and determine the sequence size based often on smaller sub-problems — this aligns closely with dynamic programming or a greedy approach where at each step, the problem is broken down into smaller sub-problems.
The essence of the solution is derived from the fact that efficient replication (via pasting) hinges on the factors of n
. Voyager into deeper, endeavor into efficient algorithm design perhaps could look into progressively building up the number of 'A's and utilizing each step most advantageous, considering the number operations it necessitates.
Solutions
- C++
- Java
- Python
class Solution {
public:
int calculateMinSteps(int number) {
int result = 0;
int divisor = 2;
while (number > 1) {
while (number % divisor == 0) {
result += divisor;
number /= divisor;
}
divisor++;
}
return result;
}
};
This summary explains a C++ solution for the "2 Keys Keyboard" problem, where the goal is to compute the minimum number of steps to create a given number of characters using only two possible operations: copy all and paste.
Begin with a function
calculateMinSteps
which takes an integernumber
as input, representing the total number of characters to be formed.Initialize two integers,
result
to store the cumulative number of steps, anddivisor
starting at 2, to factorize thenumber
.Use a nested
while
loop:- The outer
while
loop continues untilnumber
is greater than 1. - The inner
while
loop executes whilenumber
can be evenly divided bydivisor
. Each time this condition is met:- Add the value of
divisor
toresult
. - Divide
number
bydivisor
to reduce it.
- Add the value of
- The outer
Increment
divisor
after the inner loop finishes, moving to the next potential factor.The function returns
result
as the final count of minimum steps needed to reach the targetnumber
of characters.
The approach leverages prime factorization, noticing that the least costly way to multiply the pasted characters is by repeatedly using the most efficient multiplication represented by the prime factors of the target number.
class Solution {
public int minOperations(int number) {
int result = 0;
int divisor = 2;
while (number > 1) {
while (number % divisor == 0) {
result += divisor;
number /= divisor;
}
divisor++;
}
return result;
}
}
The provided Java solution addresses the problem of determining the minimum number of operations required to get n
characters starting from the first character on a keyboard, using operations that allow copying and pasting. The core idea is to decompose the target number n
into its prime factors. The strategy involves dividing n
repeatedly by its smallest divisor (starting from 2) while adding that divisor to a cumulative result until n
becomes 1.
Key highlights of the code:
- Initialize
result
to store the total count of operations. - Use a while loop to handle the division of the number by its divisors, starting from 2.
- Nested within this, another while loop checks if the divisor divides
number
completely without remainder; in such cases, the divisor is added toresult
and the number is divided by the divisor. - Increment divisor by 1 after exploring all multiple divisibility by the current divisor.
- Return the value of
result
which will provide the minimum operations needed.
This code effectively uses factorization to determine the most efficient way (minimum operations) to reach the desired number of characters via strategic copy-paste operations modeled in a mathematical way.
class Solution:
def minimumSteps(self, number: int) -> int:
result = 0
divisor = 2
while number > 1:
while number % divisor == 0:
result += divisor
number //= divisor
divisor += 1
return result
The "2 Keys Keyboard" problem involves finding the minimum number of steps to produce a specific number of 'A's on a screen using only two operations: copy all and paste. The solution provided uses Python to functionally determine the minimum steps.
Here’s how the solution works:
- Initialize the
result
variable to zero to accumulate the total number of operations. - Start with the initial
divisor
value of 2, which signifies the first possible factorization other than 1. - Use a while loop to check if the input number is greater than 1.
- Inside this while loop, the inner loop checks if the number is divisible by the current divisor without a remainder.
- If so, the
result
accumulates the divisor's value (simulating the operations needed), and the author divides the number by the divisor (simulating the reduction of problem size). - Increment the divisor after processing to check the next possible factor.
- The process repeats until the number cannot be reduced further, implying the optimal number of operations is achieved.
Return result
, which holds the minimized operation count needed. The use of factorization here cleverly reduces the problem size by breaking down the number into its constituent factors, optimizing the number of operations needed compared to if trying to handle it count incrementally from 1. This approach efficiently addresses the problem in logarithmic time complexity related to the number's prime factors.
No comments yet.