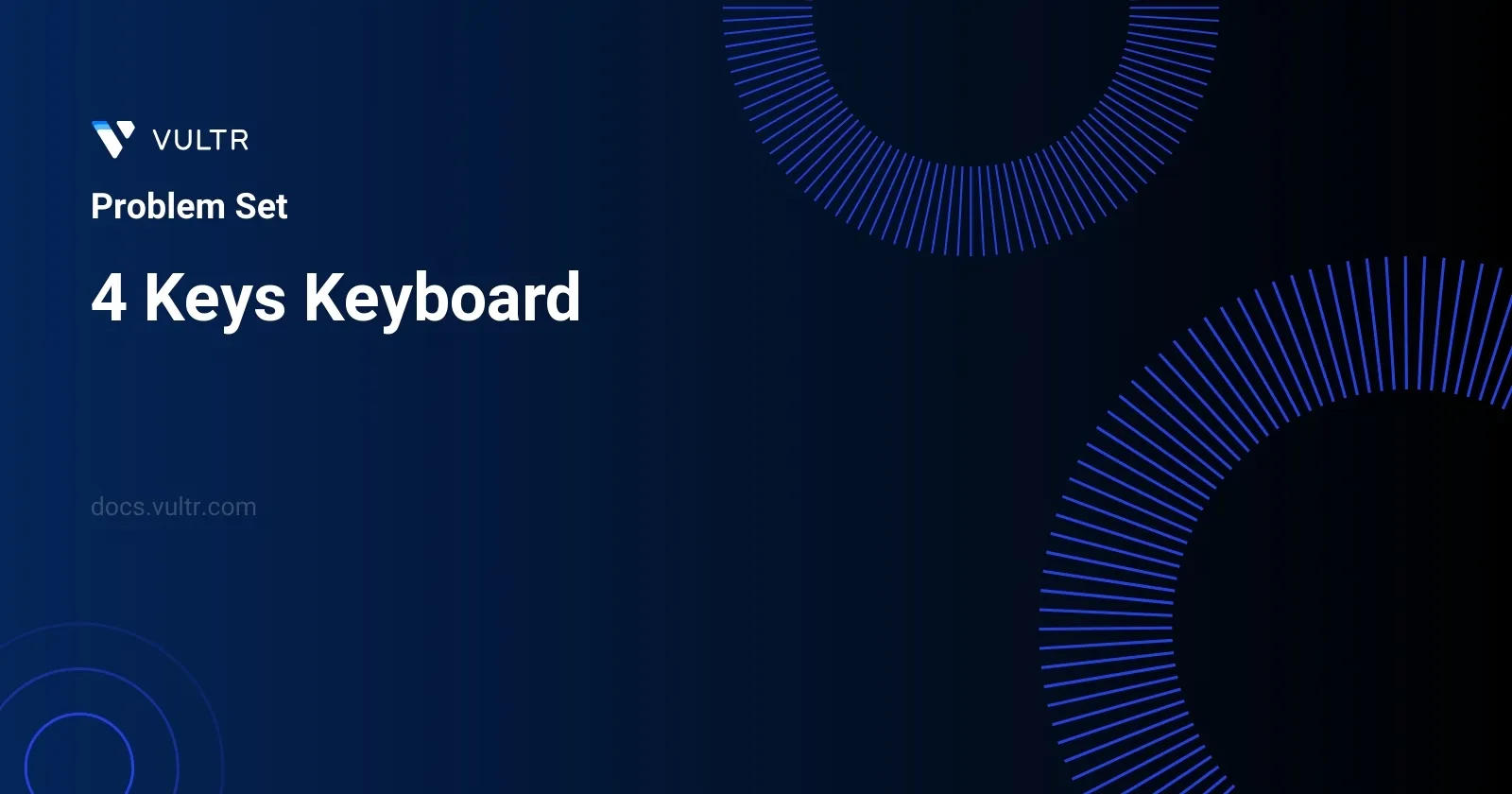
Problem Statement
In this problem, you are provided with a simulated special keyboard that has keys to print the character 'A', select the screen, copy the selection, and paste the copied content. The challenge is to determine the maximum number of 'A's one can generate on the screen using a sequence of no more than n
key presses. The different strategies blending these keys effectively to maximize output vary with the value of n
, requiring a thoughtful approach to balance direct printing versus operations that manipulate multiple characters, such as copying and pasting.
Examples
Example 1
Input:
n = 3
Output:
3
Explanation:
We can at most get 3 A's on screen by pressing the following key sequence: A, A, A
Example 2
Input:
n = 7
Output:
9
Explanation:
We can at most get 9 A's on screen by pressing following key sequence: A, A, A, Ctrl A, Ctrl C, Ctrl V, Ctrl V
Constraints
1 <= n <= 50
Approach and Intuition
The intuitive approach to this problem involves understanding the trade-offs between simply pressing 'A' multiple times and using the combination of select, copy, and paste operations:
For a small number of presses (typically less than 4), the best approach is to directly press 'A' as many times as allowable by
n
, since using copy-paste operations wouldn't be efficient or wouldn't be possible at all with less than three presses (one for copying and at least two for selecting and pasting).As
n
increases, it becomes viable to use a combination of the keys to multiply the 'A's produced:- Initially printing a few 'A's.
- Using Ctrl-A and Ctrl-C to select and copy all 'A's.
- Using Ctrl-V multiple times to double, triple, or further multiply the number of 'A's.
The approach effectively shifts from direct typing to an operational strategy as the value of
n
increases, reflecting a point where the operations (select, copy, and paste) provide a multiplicative effect beyond what direct typing would achieve.Key sequences will generally start to include one cycle of 'Ctrl-A' and 'Ctrl-C' followed by as many 'Ctrl-V' presses as can fit within the remaining key presses after initial 'A' typings. This acknowledges that each paste operation post copying doubles the count of 'A's already present on the screen, maximizing output when planned correctly.
By evaluating these points for different values of n
, we can construct a dynamic approach to calculate the maximum 'A's for any given n
. This involves testing smaller groups of 'A' prints and 'Ctrl-V' operations to see what combination fits 'n' presses while producing the most output. The solution to this kind of problem involves both dynamic programming and insightful breakdowns of operations to maximize the 'A's generated given the transferable nature of the copying operation.
Solutions
- C++
- Java
- Python
class Solution {
public:
int maximumAchievable(int steps) {
vector<int> results(steps + 1);
iota(results.begin(), results.end(), 0);
for (int idx = 0; idx <= steps - 3; idx++) {
for (int jdx = idx + 3; jdx <= min(steps, idx + 6); jdx++) {
results[jdx] = max(results[jdx], (jdx - idx - 1) * results[idx]);
}
}
return results[steps];
}
};
The code outlines a solution to the problem of determining the maximum number of characters you can output on a theoretical keyboard with four special keys. This concept is tackled in the C++ programming language.
- The
maximumAchievable
function calculates the maximum characters that can be produced given a number of keystrokes (steps
). - An array
results
of sizesteps + 1
is initialized, where each element is set to its index value. This setup essentially represents the initial state where the number of characters is equal to the number of keystrokes (e.g., for one key stroke, one character can be directly entered). - The outer loop runs through each step number, starting from zero and stopping three steps shy of the maximum to leave room for potential copy-paste operations.
- The inner loop manages the range of subsequent steps where one would utilize combinations of Ctrl-A, Ctrl-C, and Ctrl-V operations. It checks up to three additional steps to model scenarios where after copying, multiple paste operations might occur.
- Within the inner loop, the maximum number of characters is recalculated by considering one of two options at each step: continuing to type characters (the direct approach) or using the copy-paste operation (multiplying the number of characters by the number of paste operations possible after a copy).
- Finally, the function returns the value of
results[steps]
. This value is the maximum number of characters achievable after the specified number of steps.
class Solution {
public int maximumA(int limit) {
int[] resultArr = new int[limit + 1];
for (int index = 0; index <= limit; index++) {
resultArr[index] = index;
}
for (int start = 0; start <= limit - 3; start++) {
for (int end = start + 3; end <= Math.min(limit, start + 6); end++) {
resultArr[end] = Math.max(resultArr[end], (end - start - 1) * resultArr[start]);
}
}
return resultArr[limit];
}
}
The Java solution provided addresses the problem of maximizing the number of 'A's that can be printed using a keyboard with four keys, given a limit on the number of key presses. The keyboard has the following keys:
- Key 1: Prints 'A'.
- Key 2: Selects all the text on the screen.
- Key 3: Copies the selected text to the clipboard.
- Key 4: Pastes the text from the clipboard.
Follow the explanation for understanding how the code works:
An array
resultArr
is initialized with a size oflimit + 1
to store the maximum number of 'A's that can be produced with each number of key presses from 0 tolimit
.Initially, the array is populated such that the number of key presses directly corresponds to the number of 'A's printed (
resultArr[index] = index
), assuming only the 'A' key is used.A nested loop structure then considers each possible number of key presses, adjusting the array values based on the possibility of using combinations of copying and pasting after a certain initial number of direct 'A' key presses.
The outer loop variable
start
represents the initial number of times the 'A' key is pressed directly.The inner loop variable
end
represents the total number of key presses, which includes the times the other keys (select, copy, paste) could be used.The condition checks (
end <= Math.min(limit, start + 6)
) ensure that after pressing 'A' initially, the next moves consider using select, copy, and paste operations within the bounds of allowed key presses.
Each element in
resultArr
at positionend
is updated to be the maximum value between the current value and the product of(end - start - 1)
timesresultArr[start]
. This calculation simulates the effect of copying all previously generated 'A's and pasting them to maximize the output.Ultimately, the function returns the value of
resultArr[limit]
, which contains the maximum number of 'A's that can be generated withlimit
key presses.
This implementation efficiently leverages dynamic programming principles to solve the problem in a computationally efficient manner. By iteratively updating the array based on previous calculations, the approach avoids redundant recalculations and optimizes performance.
class Solution:
def findMaxA(self, input_n: int) -> int:
dp_table = list(range(input_n + 1))
for idx in range(input_n - 2):
for sub_idx in range(idx + 3, min(input_n, idx + 6) + 1):
dp_table[sub_idx] = max(dp_table[sub_idx], (sub_idx - idx - 1) * dp_table[idx])
return dp_table[input_n]
This solution focuses on solving the problem of determining the maximum number of 'A's that can be printed using a fixed number of key operations when four specific keys can be used.
Initialize a one-dimensional dynamic programming table,
dp_table
, with a length ofinput_n + 1
. The values indp_table
initially match the indices of the array which represents the number of 'A's that can be printed with i key presses without using any special key sequences.Loop through the operations from the beginning up to
input_n - 2
. For each positionidx
, consider the effects of using the operations at indices fromidx + 3
to the minimum ofinput_n
oridx + 6
.In the inner loop, calculate the potential number of 'A's that can be produced by selecting a sequence of operations that includes pressing 'Ctrl-A', 'Ctrl-C', and then 'Ctrl-V' multiple times. This is achieved by multiplying the number of 'A's that could be produced by the time of the first 'Ctrl-A' (
dp_table[idx]
) by the number of pastes ((sub_idx - idx - 1)
). Updatedp_table[sub_idx]
if this value exceeds the current value.At the end of the iteration,
dp_table[input_n]
will contain the maximum number of 'A's that can be printed withinput_n
operations using the optimal sequence of keystrokes.This approach effectively utilizes dynamic programming to solve for the optimal solution at each step, leveraging previously computed results to build up to the final answer, ensuring efficient use of computation resources and time.
No comments yet.