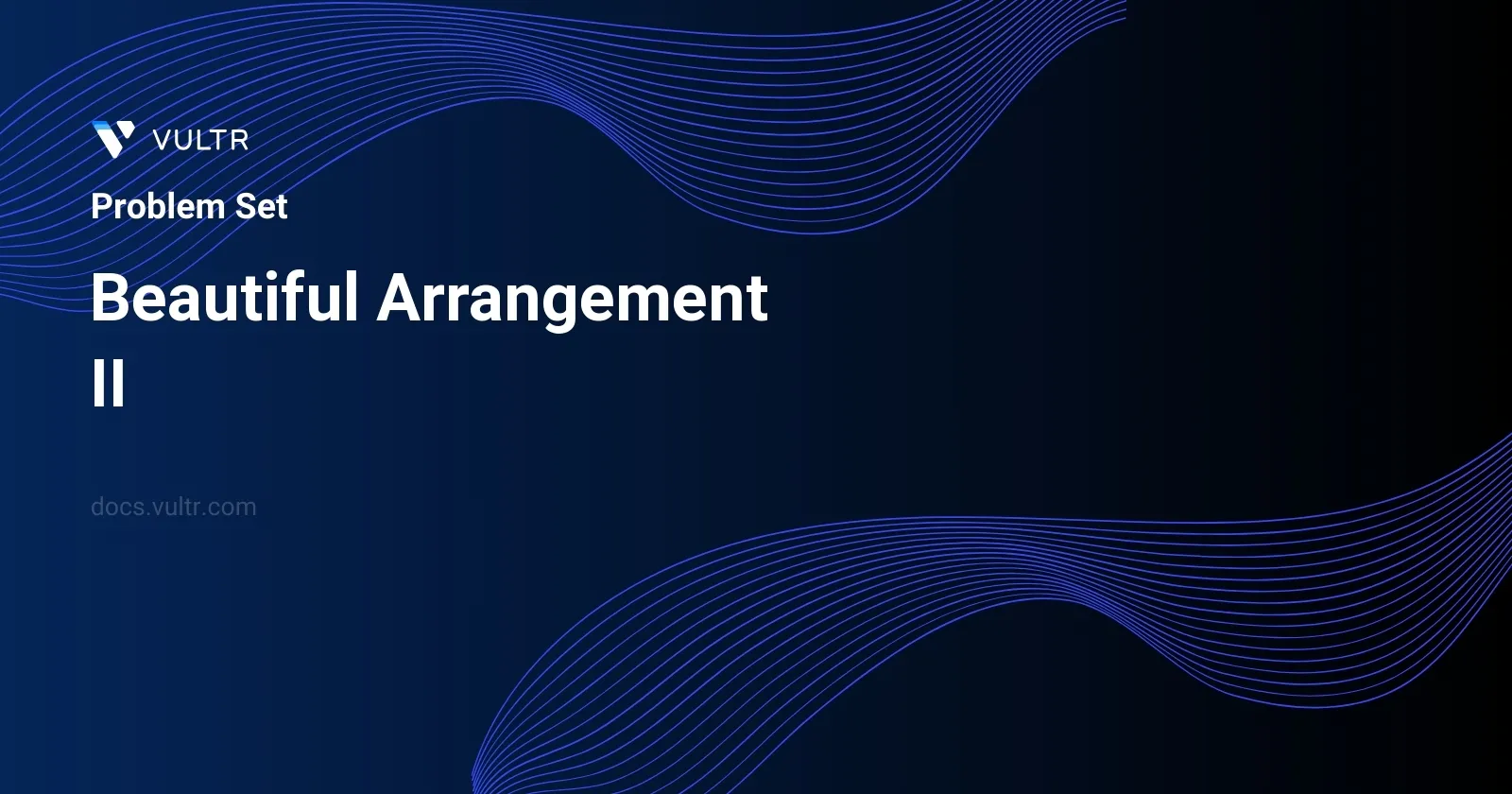
Problem Statement
Given two integers, n
and k
, the objective is to construct a sequence called answer
consisting of n
distinct positive integers, each integer within the range from 1 to n
. The sequence must fulfill a specific condition related to its consecutive differences. Specifically, when you compute the absolute differences between every two consecutive integers in the sequence (answer
), the resulting sequence of differences must contain exactly k
distinct integers. Your goal is to return any valid sequence that meets these criteria. If multiple valid sequences exist, you may return any one of them.
Examples
Example 1
Input:
n = 3, k = 1
Output:
[1,2,3] Explanation: The [1,2,3] has three different positive integers ranging from 1 to 3, and the [1,1] has exactly 1 distinct integer: 1
Example 2
Input:
n = 3, k = 2
Output:
[1,3,2] Explanation: The [1,3,2] has three different positive integers ranging from 1 to 3, and the [2,1] has exactly 2 distinct integers: 1 and 2.
Constraints
1 <= k < n <= 104
Approach and Intuition
The task is to generate a permutation of numbers from 1 to n
such that the number of distinct absolute differences between consecutive numbers is exactly k
. To achieve this, one can use strategic positioning of numbers to control the differences generated. Here’s a general approach:
Understand that the largest difference we can create in an array [1,2,3,...,n] is between 1 and
n
, which isn-1
. Hence, the first point is ensuring our sequence can generatek
distinct differences ranging likely between1
andk
.Start by visualizing how the permutations and their differences work. A direct sequence like [1, 2, 3, ..., n] only gives one distinct difference (
1
). On the other hand, a sequence with a bit more variation such as [1, n, 2, n-1, 3, n-2,...] starts to generate larger and varying differences.Generating the sequence:
- If
k
is1
, simply return the sequence [1, 2, 3, ..., n] since all differences are1
. - If
k
is greater than1
, start by arranging the firstk+1
elements to cover the k differences. For example, placing numbers in the format [1, k+1, 2, k, 3, k-1,...] ensures that we start the list with the maximum difference ofk
and introduce decrementing differences as we continue to complete the series with rest of the left numbers.
- If
Example elaboration:
- For
n = 3
andk = 1
, the sequence [1, 2, 3] fulfills the criteria because all differences are 1 which is the only distinct integer needed. - For
n = 3
andk = 2
, a sequence like [1, 3, 2] will have differences of[2, 1]
fulfilling the need for two distinct differences: 1 and 2.
- For
By strategically placing your integers and understanding the concept of differences, a valid permutation can be constructed efficiently to meet any given k
. The highest value of k
(just below n
) suggests a need for maximized manipulation in arrangement to achieve varied and distinct differences, which is achievable within the given constraints.
Solutions
- C++
- Java
- Python
class Solution {
public:
vector<int> generateArray(int n, int k) {
vector<int> result(n, 0);
int index = 0;
for (int value = 1; value < n - k; value++) {
result[index++] = value;
}
for (int j = 0; j <= k; j++) {
result[index++] = (j % 2 == 0) ? (n - k + j / 2) : (n - j / 2);
}
return result;
}
};
The problem "Beautiful Arrangement II" challenges you to generate an array of integers from 1 to n that consists of exactly k different absolute differences between consecutive elements.
Here's a breakdown of the C++ solution, which utilizes a methodical strategy:
- The solution defines a function
generateArray
that takes two parameters,n
andk
. - It initializes a result vector
result
of sizen
, with all values set to zero. - Utilizes a loop to fill up the first part of the result vector linearly from
1
ton-k
, ensuring that the differences between these consecutive elements are precisely1
. - The second part of the vector is filled in a pattern designed to alternate and create a larger difference between elements, thus ensuring that
k
distinct differences are achieved. This section uses more complex arithmetic to determine element placement based on even and odd indices, focusing on decreasing fromn
towardsn-k
and inversely. - Returns the vector
result
as an output which will satisfy the condition of having exactlyk
unique differences between consecutive values.
The solution cleverly adjusts the arrangement of the later elements to achieve the required k
distinct differences, balancing simpler initial sequences with a more complex subsequent pattern. This method ensures an efficient creation of the desired array using mathematical patterns.
class Solution {
public int[] generateSequence(int length, int diff) {
int[] result = new int[length];
int index = 0;
for (int value = 1; value < length - diff; value++) {
result[index++] = value;
}
for (int j = 0; j <= diff; j++) {
result[index++] = (j % 2 == 0) ? (length - diff + j / 2) : (length - j / 2);
}
return result;
}
}
The Java solution provided generates a sequence of integers based on two parameters: the length of the array and the difference between consecutive elements. The method generateSequence(int length, int diff)
is employed to achieve this in several key steps:
- Initialize an array
result
of integers with a size equal tolength
. - Iterate from
1
up tolength - diff
, and store each value inresult
. - In a second loop, compute values based on the modulo operation to decide if the value should be incremented from the middle point of the sequence up or decremented down, then stored in the
result
array.
The result is a permutation of the numbers from 1
to length
such that the absolute difference between any two consecutive integers is close to the specified diff
. This algorithm efficiently creates an array with the desired properties by splitting the filling process into two parts: a linear increase and a conditional filling based on the difference requirement.
class Solution:
def createSequence(self, size: int, diff: int) -> List[int]:
sequence = [0] * size
index = 0
for value in range(1, size - diff):
sequence[index] = value
index += 1
for j in range(diff + 1):
sequence[index] = size - diff + j // 2 if j % 2 == 0 else size - j // 2
index += 1
return sequence
The mentioned Python function createSequence
constructs a sequence of integers of a specified length that maintains a provided difference between elements, intended to meet certain conditions for "arrangement beauty."
Here's a summary of the function logic:
- Initialize sequence as a list of zeros with size equal to the input
size
. - Populate the sequence with increasing natural numbers until the value is less than
size - diff
. - For the remaining part of the sequence, structure the elements to maintain the specified difference. This involves alternating calculations based on whether the index is even or odd.
- The elements added are either increasing by smaller jumps or directly decreasing depending on their position to align with the difference given.
This function benefits the most:
- When creating patterns within a numeric sequence.
- In scenarios where control over specific differences between consecutive numbers in a sequence is required, such as generating test cases for algorithms focusing on sequence properties or constraints.
Always test with various size
and diff
values to confirm that the sequence meets the desired properties and that it adheres to the intended "beauty" conditions in all cases.
No comments yet.