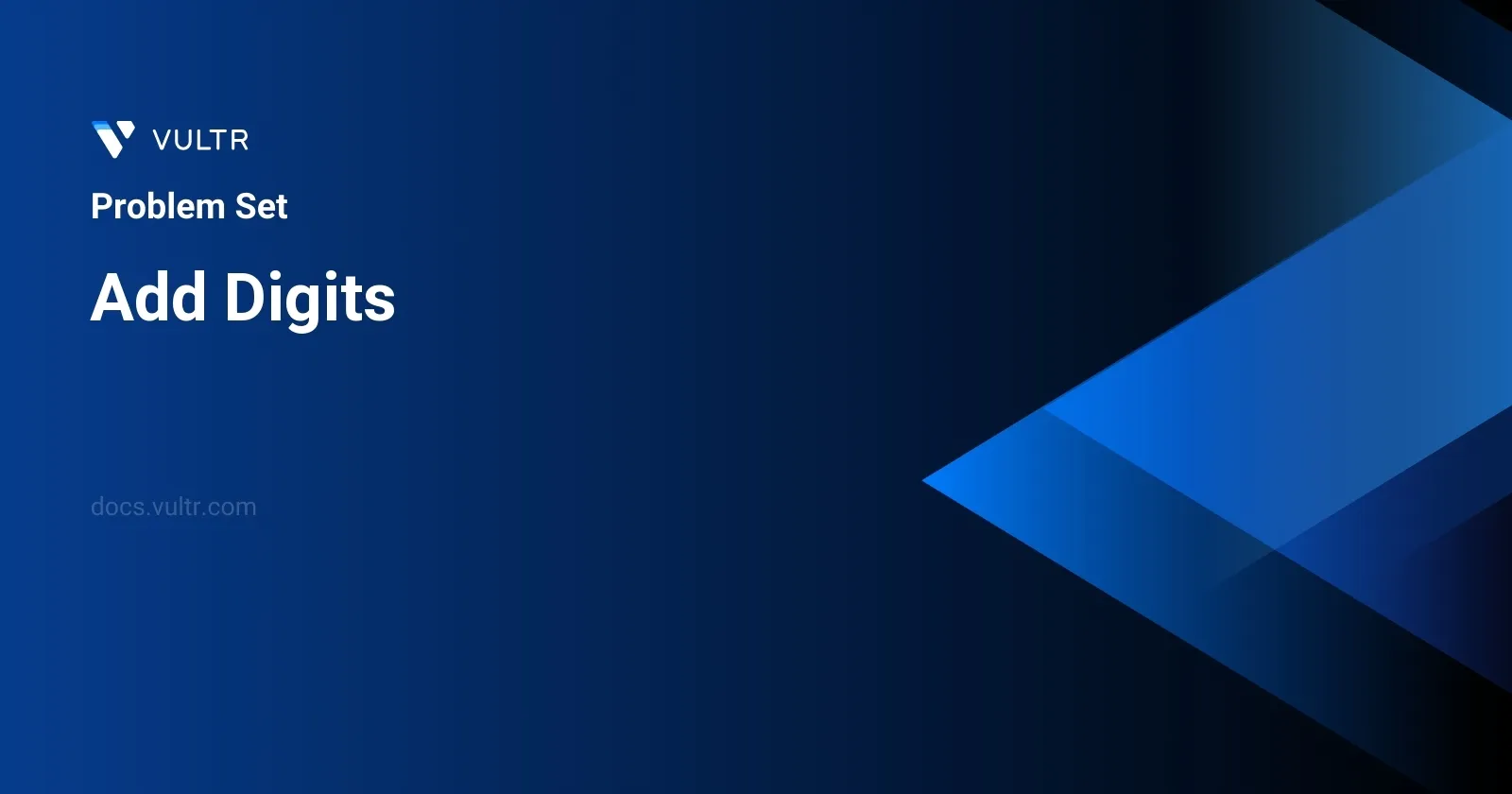
Problem Statement
When you encounter a problem that involves reducing a number down to a single-digit summary or identity, digital root is often behind the scenes. In the given prompt, the task is to compute the digital root of a non-negative integer num
. This is accomplished by repeatedly summing the digits of num
until only one digit remains. The operation involves breaking down the number into its component digits, summing them, and repeating the process with the new sum until it's a single digit.
The input, num
, ranges from 0 up to 2^31 - 1, which sets a wide range for potential input values. For instance, starting with num = 38
, the digits of 38 (3 + 8) sum to 11. Subsequently, adding the digits of 11 (1 + 1) results in 2, and since 2 is a single digit, it's the output.
The minimal nature of this problem inherently relies on both the properties of numbers and a bit of looping logic or recursion to achieve the result.
Examples
Example 1
Input:
num = 38
Output:
2
Explanation:
The process is 38 --> 3 + 8 --> 11 11 --> 1 + 1 --> 2 Since 2 has only one digit, return it.
Example 2
Input:
num = 0
Output:
0
Constraints
0 <= num <= 231 - 1
Approach and Intuition
This problem can be approached directly as described or through the properties of number theory, specifically utilizing the concept of digital roots and modulo operation. The key observations and possible implementation steps can be broken down as follows:
Direct Summation Method:
- Initialize a variable to store the current sum of digits.
- While the number has more than one digit (i.e., 10 or higher):
- Break down the number into its individual digits.
- Sum these digits and assign the result as the new number.
- Repeat until the number is a single digit.
- Return the resulting single-digit number.
Optimized Digital Root Method Using Modulo:
- Recognize that the process of summing the digits repeatedly can be related to finding the digital root through modulo operations.
- Mathematical observation tells us that the digital root of a number is equivalent to that number modulo 9, except when the number is 0 or a multiple of 9.
- Use the formula:
num % 9
and special case handling such as:- If
num
equals zero, return 0 immediately as its digital root. - If
num % 9
equals zero (andnum
is not zero), return 9. - Otherwise, return
num % 9
.
- If
The modulo approach is particularly efficient as it slices down the repetitive manual summation work seen in the direct summation method and reaches the answer in constant time, making it suitable for large numbers. However, it's important to treat both methods, as each holds distinct learning opportunities and insights into processing numbers and their properties.
Solutions
- C++
- Java
- Python
class Solution {
public:
int sumDigits(int n) {
if (n == 0) return 0;
if (n % 9 == 0) return 9;
return n % 9;
}
};
The "Add Digits" solution in C++ efficiently computes the repeated sum of all the digits in a number until a single digit is obtained. The function sumDigits
returns the result using properties of digital roots:
- Check if the input integer
n
is zero; if true, return 0. - Determine if
n
modulo 9 equals zero; if true, return 9 as the digital root of multiples of 9 is always 9. - Otherwise, return
n
modulo 9, which is the digital root for other numbers not divisible by 9.
This approach leverages mathematical properties to avoid iterative or recursive summing of digits, thus providing a constant time computational complexity solution. The function can rapidly determine the digital root for any integer provided as input.
class Solution {
public int computeSumOfDigits(int value) {
if (value == 0) return 0;
if (value % 9 == 0) return 9;
return value % 9;
}
}
This solution, implemented in Java, provides a method named computeSumOfDigits
for the "Add Digits" problem. This method efficiently computes the digital root of a given integer value
. The digital root is the single digit obtained by iteratively summing the digits of the input number until a single digit is obtained. The clever implementation takes advantage of the properties of the number 9 in modular arithmetic to achieve this. If the number is zero, it directly returns zero. For any other number, it checks if the number is divisible by 9, returning 9 in such cases. Otherwise, it returns the remainder of the number when divided by 9. This approach eliminates the need for iterative summing of the digits, resulting in constant time complexity, O(1)
.
class Solution:
def sumDigits(self, number: int) -> int:
if number == 0:
return 0
if number % 9 == 0:
return 9
return number % 9
The provided Python solution offers a simple and efficient method to "Add Digits" in an integer until a single digit number is obtained. Here's how it achieves this:
- Define a function
sumDigits
within the classSolution
that accepts an integernumber
. - If the input number is zero, immediately return 0, as its sum of digits is clearly 0.
- Check if the number is divisible by 9 using the modulus operator. When a number is divisible by 9, its digits add up to 9.
- If not divisible by 9, return the remainder of the division of the number by 9 to get the sum of digits.
- This solution leverages properties of numbers related to the base 9 system, bypassing the need to continuously sum the digits until a single-digit result is obtained, thereby significantly enhancing performance.
No comments yet.